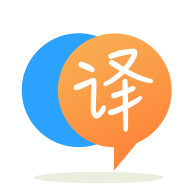
[英]How can I split string and integer when user input both the string and the integer in the same line in C++?
[英]C++ : How to assign the same input to a string and an integer in 2 lines?
我是C ++的初学者,实际上我在关注Google教程 。
尝试进一步讲第二个例子,这是我的问题: 检查输入是否为数字,如果不是,则可以在错误消息中重新输入 。
这是我用来解决该问题的一种方法,但是代码长度告诉我,有一种更短的方法:
#include <cstddef>
#include <iomanip>
#include <iostream>
#include <stdlib.h>
using namespace std;
bool IsInteger(string str) {
size_t non_num_position = str.find_first_not_of("0123456789-");
size_t sign_position = str.find_first_of("-", 1);
if (non_num_position == string::npos && sign_position == string::npos) {
return true;
}
return false;
}
void Guess() {
int input_number = 0;
string input_string;
do {
cout << "Try to guess the number between 0 and 100 (type -1 to quit) : ";
cin >> input_string;
if (!IsInteger(input_string)) {
int input_string_length = input_string.size();
cout << "Sorry but « " << input_string << " » is not a number." << endl;
cin.clear();
cin.ignore(input_string_length, '\n');
continue;
}
input_number = atoi(input_string.c_str());
if (input_number != -1) {
cout << "You chose " << input_number << endl;
}
} while (input_number != -1);
cout << "The End." << endl;
}
int main() {
Guess();
return 0;
}
这是我尝试遵循的较短方法,但是一旦分配给input_number
(由于按位运算符?), cin
似乎就被“清空了”:
void Guess() {
int input_number = 0;
string input_string;
do {
cout << "Try to guess the number between 0 and 100 (type -1 to quit) : ";
if (!(cin >> input_number)) {
getline(cin, input_string);
cout << "Sorry but " << input_string << " is not a number." << endl;
cin.clear();
cin.ignore(100, '\n');
continue;
}
if (input_number != -1) {
cout << "You chose " << input_number << endl;
}
} while (input_number != -1);
cout << "The End." << endl;
}
解决方案:
#include <iomanip>
#include <iostream>
#include <string>
using namespace std;
void Guess() {
int input_number = 0;
string input_string;
do {
cout << "Try to guess the number between 0 and 100 (type -1 to quit) : ";
cin >> input_string;
try {
input_number = stoi(input_string);
if (input_number != -1) {
cout << "You chose " << input_number << endl;
}
}
catch (const exception&) {
cout << "Sorry but " << input_string << " is not a number." << endl;
}
} while (input_number != -1);
cout << "The End." << endl;
}
int main() {
Guess();
return 0;
}
第一次尝试的问题是IsInteger不必要地复杂且冗长。 否则,您有正确的想法。 您的第二次尝试的正确性要差得多。...从cin读取后,数据就消失了。 因此,与第一次尝试一样,您需要将数据存储在字符串中。
这是一个短一些的示例,根本不需要IsInteger:
size_t p = 0;
int input_number = std::stoi(input_string, &p);
if (p < input_string.length())
{
cout << "Sorry but " << input_string << " is not a number." << endl;
conitnue;
}
stoi
的第二个参数告诉您到整数的转换在哪里停止工作。 因此,如果字符串中包含非整数数据(例如'123abc'),则p将在字符串末尾之前。 如果p位于末尾,则整个字符串必须是一个数字。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.