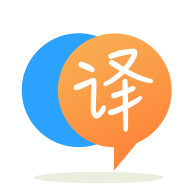
[英]Overloading variadic-templated member function and variadic-templated functions
[英]Overloading a templated member function through enum
假设我有一个EventHandle
类,该类由READ
, WRITE
和SIGNAL
唯一枚举,并且我正在实现一个成员模板,该模板应针对不同的枚举返回不同的数据类型。
enum class EventType {
READ,
WRITE,
SIGNAL
};
class EventHandle {
public:
template <EventType type, typename = enable_if_t<is_same<type, READ>::value>>
ReadEventHandle* cast () { return static_cast<ReadEventHandle>(this); }
template <EventType type, typename = enable_if_t<is_same<type, WRITE>::value>>
WriteEventHandle* cast () { return static_cast<WriteEventHandle>(this); }
template <EventType type, typename = enable_if_t<is_same<type, SIGNAL>::value>>
SignalEventHandle* cast () { return static_cast<SignalEventHandle>(this); }
};
我有三个EventHandle
派生类。
class ReadEventHandle : public EventHandle {...}
class WriteEventHandle : public EventHandle {...}
class SignalEventHandle : public EventHandle {...}
我写的东西显然不能编译。 有什么办法可以在“编译”时实现这种类型的重载(例如,没有打开枚举)?
我不能说我完全理解您要做什么,但是您肯定没有正确使用SFINAE。 这是可编译的代码,我希望可以作为指导:
#include <type_traits>
enum class EventType {
READ,
WRITE,
SIGNAL
};
class ReadEventHandle { };
class WriteEventHandle { };
class SignalEventHandle { };
ReadEventHandle re;
WriteEventHandle we;
SignalEventHandle se;
class EventHandle {
public:
template <EventType type, std::enable_if_t<type == EventType::READ>* = nullptr >
ReadEventHandle* cast () { return &re; }
template <EventType type, std::enable_if_t<type == EventType::WRITE>* = nullptr >
WriteEventHandle* cast () { return &we; }
template <EventType type, std::enable_if_t<type == EventType::SIGNAL>* = nullptr>
SignalEventHandle* cast () { return &se; }
};
void* check() {
return EventHandle().cast<EventType::READ>(); // Depending on cast argument, different pointers returned
}
具有某些特征和专业化的替代方案:
enum class EventType {
READ,
WRITE,
SIGNAL
};
class ReadEventHandle;
class WriteEventHandle;
class SignalEventHandle;
template <EventType E> struct EventHandleType;
template <> struct EventHandleType<EventType::READ> { using type = ReadEventHandle; };
template <> struct EventHandleType<EventType::WRITE> { using type = WriteEventHandle; };
template <> struct EventHandleType<EventType::SIGNAL> { using type = SignalEventHandle; };
接着:
class EventHandle {
public:
template <EventType E>
typename EventHandleType<E>::type* cast();
};
template <EventType E>
typename EventHandleType<E>::type*
EventHandle::cast() { return static_cast<typename EventHandleType<E>::type*>(this); }
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.