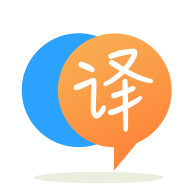
[英]how to detect unique ibeacon advertisements react-native on ios device?
[英]How to detect ibeacon device without knowing UUID in iOS?
我想在不知道uuid的情况下开发能够检测到许多信标设备的应用程序。 但是我找不到办法做到这一点。 我必须在代码中定义uuid。
我开发了一种可与已知设备配合使用的POC。
我的视图控制器代码:
-(void)setUpview
{
// Regardless of whether the device is a transmitter or receiver, we need a beacon region.
NSUUID * uid = [[NSUUID alloc] initWithUUIDString:@"78CDC73D-D678-4B35-A88A-C2E09E5B963F"];//[UIDevice currentDevice].identifierForVendor;
treasureId = @"com.eden.treasure";
self.beaconRegion = [[CLBeaconRegion alloc] initWithProximityUUID:uid identifier:treasureId];
// Location manager.
self.locationManager = [[CLLocationManager alloc] init];
self.locationManager.delegate = self;
if([self.locationManager respondsToSelector:@selector(requestAlwaysAuthorization)]) {
[self.locationManager requestAlwaysAuthorization];
}
[self.locationManager startMonitoringForRegion:self.beaconRegion];
[self.locationManager startRangingBeaconsInRegion:self.beaconRegion];
[self locationManager:self.locationManager didStartMonitoringForRegion:self.beaconRegion];
[self.beaconRegion setNotifyEntryStateOnDisplay:YES];
[self.beaconRegion setNotifyOnEntry:YES];
[self.beaconRegion setNotifyOnExit:YES];
// self.peripheralManager = [[CBPeripheralManager alloc] initWithDelegate:self queue:nil];
if ([UIDevice currentDevice].userInterfaceIdiom==UIUserInterfaceIdiomPad || [[[UIDevice currentDevice] model] isEqualToString:@"iPad Simulator"])
{
[self configureTransmitter];
}
else {
[self configureReceiver];
}
}
-(void)configureTransmitter
{
// The received signal strength indicator (RSSI) value (measured in decibels) for the device. This value represents the measured strength of the beacon from one meter away and is used during ranging. Specify nil to use the default value for the device.
NSNumber * power = [NSNumber numberWithInt:-63];
self.peripheralData = [self.beaconRegion peripheralDataWithMeasuredPower:power];
// Get the global dispatch queue.
dispatch_queue_t queue = dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0);
// Create a peripheral manager.
self.peripheralManager = [[CBPeripheralManager alloc] initWithDelegate:self queue:queue];
}
-(void)configureReceiver {
// Location manager.
self.locationManager = [[CLLocationManager alloc] init];
self.locationManager.delegate = self;
[self.locationManager requestAlwaysAuthorization];
[self.locationManager startMonitoringForRegion:self.beaconRegion];
[self.locationManager startRangingBeaconsInRegion:self.beaconRegion];
}
#pragma mark - CBPeripheralManagerDelegate methods
-(void)peripheralManagerDidUpdateState:(CBPeripheralManager *)peripheral
{
// The peripheral is now active, this means the bluetooth adapter is all good so we can start advertising.
if (peripheral.state == CBPeripheralManagerStatePoweredOn)
{
[self.peripheralManager startAdvertising:self.peripheralData];
}
else if (peripheral.state == CBPeripheralManagerStatePoweredOff)
{
NSLog(@"Powered Off");
[self.peripheralManager stopAdvertising];
}
}
-(void)locationManager:(CLLocationManager *)manager didDetermineState:(CLRegionState)state forRegion:(CLRegion *)region
{
if(state == CLRegionStateInside)
{
NSLog(@"%@",[NSString stringWithFormat:@"You are inside region %@", region.identifier]);
}
else if(state == CLRegionStateOutside)
{
NSLog(@"%@",[NSString stringWithFormat:@"You are outside region %@", region.identifier]);
}
else
{
return;
}
}
-(void)locationManager:(CLLocationManager *)manager didRangeBeacons:(NSArray *)beacons inRegion:(CLBeaconRegion *)region
{
if ([beacons count] == 0)
return;
NSString * message;
UIColor * bgColor;
CLBeacon * beacon = [beacons firstObject];
switch (beacon.proximity) {
case CLProximityUnknown:
message = [NSString stringWithFormat:@"ProximityUnknown -- %ld", (long)beacon.proximity];
bgColor = [UIColor blueColor];
break;
case CLProximityFar:
message = [NSString stringWithFormat:@"ProximityFar -- %ld", (long)beacon.proximity];
bgColor = [UIColor colorWithRed:.0f green:.0f blue:230.0f alpha:1.0f];
break;
case CLProximityNear:
message = [NSString stringWithFormat:@"ProximityNear -- %ld", (long)beacon.proximity];
bgColor = [UIColor orangeColor];
break;
case CLProximityImmediate:
default:
message = [NSString stringWithFormat:@"ProximityImmediate -- %ld", (long)beacon.proximity];
bgColor = [UIColor redColor];
break;
}
if (beacon.proximity != self.previousProximity)
{
[lblStatus setText:message];
[self.view setBackgroundColor:bgColor];
self.previousProximity = beacon.proximity;
}
}
那么有没有办法在不知道uuid的情况下检测iBeacon?
注意:使用与检查相同功能的示例应用程序此链接广播广播信号(虚拟信标)。
任何帮助将受到高度赞赏。
在没有首先知道信标的ProximityUUID的情况下,iOS中没有用于检测信标的公共API 。 这是设计 - Apple只希望您能够看到自己的信标,这意味着了解ProximityUUID。
也就是说,操作系统当然知道如何查看任何信标,并且可能有私有API可用于执行此操作 。 只需了解您不能使用私有API为App Store开发应用程序,因为Apple不会批准使用此类代码。 您可以使用私有API来创建使用您自己的iOS设备的实用程序,但私有API可能会停止使用任何iOS升级
有了这些警告,使用CoreLocation
,可以定义一个通配符CLBeaconRegion
,可用于使用私有API查找CLBeaconRegion
标。 请参阅此处了解一种方法。
您需要使用CoreBluetooth
库来扫描设备。 从已发现设备列表中找到iBeacon后,您将查询iBeacon的服务和特征以查找UUID。 我是这样做的:
import CoreBluetooth
import CoreLocation
extension Data {
public var hexString: String {
var str = ""
enumerateBytes { (buffer, index, stop) in
for byte in buffer {
str.append(String(format: "%02X", byte))
}
}
return str
}
}
class viewController: UIViewController, CLLocationManagerDelegate, CBCentralManagerDelegate, CGPeripheralDelegate {
var locationManager: CLLocationManager = CLLocationManager()
var myBeaconRegion: CLBeaconRegion!
var centralManager: CBCentralManager!
var discoveredPeripheral: CBPeripheral?
override viewDidLoad() {
centralManager = CBCentralManager(delegate: self, queue: nil)
}
func centralManagerDidUpdateState(_ central: CBCentralManager) {
if central.state != .poweredOn {
return
}
centralManager.scanForPeripherals(withServices: nil, options: [CBCentralManagerScanOptionAllowDuplicateKeys: true])
}
func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String: Any], rssi RSSI: NSNumber) {
if (RSSI.intValue > -15 || RSSI.intValue < -35) {
return // With those RSSI values, probably not an iBeacon.
}
if peripheral != discoveredPeripheral {
discoveredPeripheral = peripheral // Need to retain a reference to connect to the beacon.
centralManager.connect(peripheral, options: nil)
central.stopScan() // No need to scan anymore, we found it.
}
}
func centralManager(_ central: CBCentralManager, didConnect peripheral: CBPeripheral) {
peripheral.discoverServices(nil)
}
func centralManager(_ central: CBCentralManager, didDisconnectPeripheral peripheral: CBPeripheral, error: Error?) {
discoveredPeripheral = nil
}
func peripheral(_ peripheral: CBPeripheral, didDiscoverServices error: Error?) {
guard error == nil else {
return
}
if let services = peripheral.services {
for service in services {
peripheral.discoverCharacteristics(nil, for: service)
}
}
}
func peripheral(_ peripheral: CBPeripheral, didDiscoverCharacteristicsFor service: CBService, error: Error?) {
guard error == nil else {
return
}
if let characteristics = service.characteristics {
for characteristic in characteristics {
if characteristic.uuid.uuidString == "2B24" { // UUID
peripheral.readValue(for: characteristic)
}
}
}
}
func peripheral(_ peripheral: CBPeripheral, didUpdateValueFor characteristic: CBCharacteristic, error: Error?) {
guard error == nil else {
return;
}
if value = characteristic.value {
// value will be a Data object with bits that represent the UUID you're looking for.
print("Found beacon UUID: \(value.hexString)")
// This is where you can start the CLBeaconRegion and start monitoring it, or just get the value you need.
}
}
我也在寻找同样的东西,我在这个例子中得到了答案,在这里输入链接描述
这是在没有UUID的情况下搜索所有iBeacons
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.