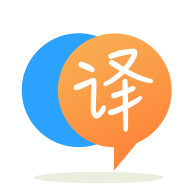
[英]Javascript Iterate an array, count the number of times each element appears and push it to an object
[英]Count the number of times a same value appears in a javascript array
我想知道是否有与此相同的本机 javascript 代码:
function f(array,value){
var n = 0;
for(i = 0; i < array.length; i++){
if(array[i] == value){n++}
}
return n;
}
为此目的可能有不同的方法。
而且您使用for
循环的方法显然没有放错地方(除了它的代码量看起来多余)。
这里有一些额外的方法来获取数组中某个值的出现:
使用Array.forEach
方法:
var arr = [2, 3, 1, 3, 4, 5, 3, 1]; function getOccurrence(array, value) { var count = 0; array.forEach((v) => (v === value && count++)); return count; } console.log(getOccurrence(arr, 1)); // 2 console.log(getOccurrence(arr, 3)); // 3
使用Array.filter
方法:
function getOccurrence(array, value) { return array.filter((v) => (v === value)).length; } console.log(getOccurrence(arr, 1)); // 2 console.log(getOccurrence(arr, 3)); // 3
您可以使用 reduce 到达那里:
var a = [1,2,3,1,2,3,4];
var map = a.reduce(function(obj, b) {
obj[b] = ++obj[b] || 1;
return obj;
}, {});
另一种选择是:
count = myArray.filter(x => x == searchValue).length;
这是我的解决方案,不使用其他对象并使用reduce
:
const occurrencesOf = (number,numbers) => numbers.reduce((counter, currentNumber)=> (number === currentNumber ? counter+1 : counter),0);
occurrencesOf(1, [1,2,3,4,5,1,1]) // returns 3
occurrencesOf(6, [1,2,3,4,5,1,1]) // returns 0
occurrencesOf(5, [1,2,3,4,5,1,1]) // returns 1
您也可以使用 forEach
let countObj = {};
let arr = [1,2,3,1,2,3,4];
let countFunc = keys => {
countObj[keys] = ++countObj[keys] || 1;
}
arr.forEach(countFunc);
// {1: 2, 2: 2, 3: 2, 4: 1}
let ar = [2,2,3,1,4,9,5,2,1,3,4,4,8,5];
const countSameNumber = (ar: any, findNumber: any) => {
let count = 0;
ar.map((value: any) => {
if(value === findNumber) {
count = count + 1;
}
})
return count;
}
let set = new Set(ar);
for (let entry of set) {
console.log(entry+":", countSameNumber(ar, entry));
}
您可以使用数组过滤器方法并像这样找到新数组的长度
const count = (arr, value) => arr.filter(val => val === value).length
const arr = ["a", "a", "a", "b", "b", "b", "b", "c", "c", "c"];
count = 0;
function countValues(array, countItem) {
array.forEach(itm => {
if (itm == countItem) count++;
});
console.log(`${countItem} ${count}`);
}
countValues(arr, "c");
let countValue = 0;
Array.forEach((word) => {
if (word === searchValue) {
return countValue ++;
}
});
console.log(`The number of word 'asdf': ${countValue}`);
我使用此代码来计算先前转换为数组的文本中给定单词的数量。
这就是我只使用 for 循环的方式。 它不像上面的一些答案那么复杂,但它对我有用。
function getNumOfTimes(arrayOfNums){
let found = {}
for (let i = 0; i < units.length; i++) {
let keys = units[i].toString()
found[keys] = ++found[units[i]] || 1
}
return found
}
getNumOfTimes([1, 4, 4, 4, 5, 3, 3, 3])
// { '1': 1, '3': 3, '4': 3, '5': 1 }
您可能想使用indexOf()
函数来查找和计算array
每个value
function g(array,value){
var n = -1;
var i = -1;
do {
n++;
i = array.indexOf(value, i+1);
} while (i >= 0 );
return n;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.