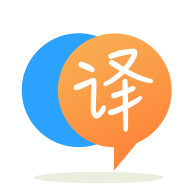
[英]How check check-box dynamically in click event using Angular.js/Javascript
[英]How to limit check box clicked using Angular.js/Javascript
我需要一个帮助。我在循环中有很多复选框,我需要使用 Angular.js 来限制使用某些条件的复选框。让我先解释一下我的代码。 在 plunkr 中,我的代码存在。我可以检查那里每天有一个复选框,如果用户单击+
按钮,复选框会增加,这里我需要当用户从任何一天开始选中最多2
复选框时被禁用。从7
天开始,只能从整个表中检查 2 个复选框。单击store
按钮后,我还需要两个复选框值和相应的行下拉数据应提取到控制器端功能中。请在此处检查我的代码并提供帮助我。
所以,另一个答案会让你走上正确的轨道,而这个答案应该会让你一路走好。 您基本上想利用 angular 的ng-disabled
属性,当您给它的表达式求值为true
,它所附加的表单字段将被禁用。
在您的 html 中,您可以在满足条件时将以下内容放在您希望禁用的任何表单字段上:
ng-disabled="isDisabled($parent.$index, $index)"
父索引将引用当前外循环的日期,索引将引用内循环中的当前答案。
我用来实现这个解决方案的主要控制器逻辑如下:
// initialize an array of selections
$scope.selectedAnswers = [];
// check if answer is selected...
// have to keep track of both the index of the answer and its parent day
$scope.answerIsSelected = function(dayIdx, answerIdx) {
var thisAnswer = $scope.days[dayIdx].answers[answerIdx];
return $scope.selectedAnswers.indexOf(thisAnswer) > -1;
};
// depending on whether answer is already selected,
// adds it to or splices it from the selectedAnswers array
$scope.toggleAnswerSelected = function(dayIdx, answerIdx) {
var thisAnswer = $scope.days[dayIdx].answers[answerIdx];
if ($scope.answerIsSelected(dayIdx, answerIdx)) {
$scope.selectedAnswers.splice($scope.selectedAnswers.indexOf(thisAnswer), 1);
} else {
$scope.selectedAnswers.push(thisAnswer);
}
};
// the check on each form element to see if should be disabled
// returns true if the answer is not an element in the selected array
// and if there are already two answers in the array
$scope.isDisabled = function(dayIdx, answerIdx) {
return !$scope.answerIsSelected(dayIdx, answerIdx) && $scope.selectedAnswers.length === 2;
};
最后,在行末尾的复选框中需要的属性:
<input type="checkbox"
name="chk"
value="true"
ng-checked="answerIsSelected($parent.$index, $index)"
ng-click="toggleAnswerSelected($parent.$index, $index)"
ng-disabled="isDisabled($parent.$index, $index)">
完整片段如下...
var app = angular.module('plunker', []); app.controller('MainCtrl', function($scope,$q, $filter) { $scope.submitted = false; $scope.init = function() { $scope.days = []; $scope.listOfCategory = [{ id:1, name: 'Category 1', value: 1 }, { id:2, name: 'Category 2', value: 2 },{ id:3, name: 'Category 3', value: 3 }]; $scope.listOfSubCategory=[]; //emulating ajax response: this should be removed, days reponse var response = { data: [{ day_name: "Monday" }, { day_name: "Tuesday" }, { day_name: "Wednesday" }, { day_name: "Thursday" }, { day_name: "Friday" }, { day_name: "Saturday", }, { day_name: "Sunday" }] }; //end response //success angular.forEach(response.data, function(obj) { obj.answers = []; $scope.addNewRow(obj.answers); $scope.days.push(obj); }); }; $scope.renderWithMode = function(index,catvalue,parent,isEditMode){ if(isEditMode){ $scope.removeBorder(index,catvalue,parent); } }; $scope.removeBorder = function(index,catvalue,parent){ //make $http call here //below code should be removed, its emulated response var subcategories = [{ id:1, name: 'SubCategory 1', value: 1 }, { id:2, name: 'SubCategory 2', value: 2 }, { id:3, name: 'SubCategory 3', value: 3 }, { id:4, name: 'SubCategory 4', value: 4 }]; //after reponse from server as subcaegory if(!$scope.listOfSubCategory[parent]){ $scope.listOfSubCategory[parent] = []; } //console.log('value',catvalue); var subcategories1=[]; // $scope.listOfSubCategory[parent][index] = angular.copy(subcategories); var result = $filter('filter')(subcategories, {id:catvalue})[0]; // console.log('result',result); subcategories1.push(result) $scope.listOfSubCategory[parent][index] = subcategories1; }; $scope.addNewRow = function(answers) { answers.push({ category: null, subcategory: null, comment: null }); }; $scope.submitAnswers = function(){ // console.log($scope.selectedAnswers); $scope.submitted = true; $scope.sendToServer = $scope.selectedAnswers; }; $scope.getResponse = function(){ //emulating reponse: this should come from server, var response = { data: [{ day_name: "Monday", answers:[{ category:2, subcategory:1, comment:'This is answer 1' },{ category:1, subcategory:2, comment:'This is answer 2' }] }, { day_name: "Tuesday", answers:[{ category:1, subcategory:2, comment:'This is answer 1' }] }, { day_name: "Wednesday" }, { day_name: "Thursday" }, { day_name: "Friday" }, { day_name: "Saturday", }, { day_name: "Sunday" }] }; $scope.days = []; angular.forEach(response.data, function(obj) { if(!obj.answers){ obj.answers = [{ category:null, subcategory:null, comment:null }]; } $scope.days.push(obj); }); $scope.isEditMode = true; }; $scope.selectedAnswers = []; $scope.answerIsSelected = function(dayIdx, answerIdx) { var thisAnswer = $scope.days[dayIdx].answers[answerIdx]; return $scope.selectedAnswers.indexOf(thisAnswer) > -1; }; $scope.toggleAnswerSelected = function(dayIdx, answerIdx) { var thisAnswer = $scope.days[dayIdx].answers[answerIdx]; if ($scope.answerIsSelected(dayIdx, answerIdx)) { $scope.selectedAnswers.splice($scope.selectedAnswers.indexOf(thisAnswer), 1); } else { $scope.selectedAnswers.push(thisAnswer); } }; $scope.isDisabled = function(dayIdx, answerIdx) { return !$scope.answerIsSelected(dayIdx, answerIdx) && $scope.selectedAnswers.length === 2; }; $scope.removeRow = function(answers,index,parent){ answers.splice(index, 1); $scope.listOfSubCategory[parent].splice(index,1); // answers.splice(answers.length-1,1); }; });
<!DOCTYPE html> <html ng-app="plunker"> <head> <meta charset="utf-8" /> <title>AngularJS Plunker</title> <script> document.write('<base href="' + document.location + '" />'); </script> <link rel="stylesheet" href="style.css" /> <script data-require="angular.js@1.4.x" src="https://code.angularjs.org/1.4.9/angular.js" data-semver="1.4.9"></script> <script src="script.js"></script> <body ng-controller="MainCtrl"> Plunker <table ng-init="init()" class="table table-bordered table-striped table-hover" id="dataTable"> <thead> <tr> <th>Day</th> <th>Category</th> <th>Sub Subcategory</th> <th>Comments Or special promotion</th> <th>Add More</th> </tr> </thead> <tbody id="detailsstockid"> <tr ng-repeat="d in days track by $index"> <td>{{d.day_name}}</td> <td> <table> <tbody> <tr ng-repeat="answer in d.answers track by $index"> <td> <select class="form-control" id="answer_{{$index}}_category" name="answer_{{$index}}_category" ng-model="answer.category" ng-options="cat.name for cat in listOfCategory track by cat.value" ng-init="renderWithMode($index,answer.category.value,$parent.$index,isEditMode)" ng-change="removeBorder($index,answer.category.value,$parent.$index)"> <option value="">Select Category</option> </select> </td> </tr> </tbody> </table> </td> <td> <table> <tbody> <tr ng-repeat="answer in d.answers track by $index"> <td> <select class="form-control" id="answer_{{$index}}_subcategory" name="answer_{{$index}}_subcategory" ng-model="answer.subcategory" ng-options="sub.name for sub in listOfSubCategory[$parent.$index][$index]"> <option value="">Select Subcategory</option> </select> </td> </tr> </tbody> </table> </td> <td> <table> <tbody> <tr ng-repeat="answer in d.answers"> <td> <input type="text" id="answer_{{$index}}_comment" name="answer_{{$index}}_comment" placeholder="Add Comment" ng-model="answer.comment" /> </td> </tr> </tbody> </table> </td> <td> <table> <tbody> <tr ng-repeat="answer in d.answers track by $index"> <td style="width:20px"> <input ng-show="d.answers.length>1" class="btn btn-red" type="button" name="minus" id="minus" value="-" style="width:20px; text-align:center;" ng-click="removeRow(d.answers, $index,$parent.$index)" /> </td> <td ng-show="$last"> <input type="button" class="btn btn-success" name="plus" id="plus" value="+" style="width:20px; text-align:center;" ng-click="addNewRow(d.answers)" /> </td> <td> <input type="checkbox" name="chk" value="true" ng-checked="answerIsSelected($parent.$index, $index)" ng-click="toggleAnswerSelected($parent.$index, $index)" ng-disabled="isDisabled($parent.$index, $index)" /> </td> </tr> </tbody> </table> </td> </tr> </tbody> </table> <input type="submit" ng-click="submitAnswers()" value="Store"> <pre ng-if="submitted"> {{ sendToServer | json }} </pre> <!-- <input type="button" value="Edit" ng-click="getResponse()">--> </body> </html>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.