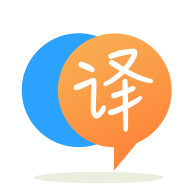
[英]C++ How to sort an array of arrays by one of the elements using comparator?
[英]Sort second Array according to the elements in the first array using c++ and remove some elements
假设我有两个看起来像这样的数组:
first array : 8 5 6 1 4 11 7
second array: 1 1 1 1 0 0 0
我想按降序对第一个数组进行排序,第二个数组中元素的顺序应与第一个数组相同,并删除第二个数组中对应值为0的第一个数组中的元素。对应值为0的第一个数组应放入另一个数组。 最后应打印两个数组的总和。
因此最终数组应如下所示:
first array : 8 6 5 1
second array: 1 1 1 1
sum= 8+6+5+1=20
具有值的新数组:
first array : 11 7 4
second array: 0 0 0
sum = 11+7+4=22
任何想法如何在C ++中做到这一点
到目前为止,这是我所做的...我尝试使用waytoShort()方法:
#include<iostream>
#include<vector>
#include<algorithm>
using namespace std;
bool wayToSort(int a, int b)
{
return a > b;
}
int main()
{
int n;
int sum;
sum=0;
cout<<"no. of elements in first array: "<<endl;
cin>>n;
//no. of elements in both array should be same.
vector<int> l(n);
vector<int> t(n);
for(int i=0;i<n;i++)
{
cin>>l[i]>>t[i];
}
sort(l.begin(),l.end(),wayToSort);
for(int i=0;i<n;i++)
{
cout<<l[i]<<" "<<t[i]<<endl;
}
for(int j= 0; j<n;j++)
{
sum = sum+l[j];
}
cout<<sum<<endl;
return 0;
}
这只会排序第一个数组。
请注意,可以在对数组进行排序之前将数组分为两部分,然后单独对每个数组进行排序。
例:
8 5 6 1 4 11 7
1 1 1 1 0 0 0
分为:
1) [8 5 6 1],[1,1,1,1]
2) [4 11 17],[0,0,0]
然后对每个数组单独排序,结果:
1) [8 6 5 1],[1,1,1,1]
2) [17 11 4],[0,0,0]
如果我对您的理解正确,则希望基于第二个元素过滤一个元组列表,然后希望基于第一个元素对一个元组列表进行排序。 我没有发现对操作顺序有任何重大影响,因此您应该先进行过滤。
将问题表示为两组不同的元素可能不是解决问题的方法(当然,这是假定您由于某些外部约束而不需要使用两个单独的列表)。
与上述保持一致,您应该使用一个列表,但它应该是成对的(std :: pair)。 基于X的任何突变都会将Y隐式拖向行驶。
至于删除其右元素== 0的对。那应该是线性的。 即使您首先这样做,也不会注意到对整体运行时间(通过成对排列的单次运行,无论如何都是真正的繁重工作)的结果影响。
对配对非常简单:
按字符串对std :: vector <std :: pair <std :: string,bool >>排序?
数据表示的选择很重要。 您可能想要使用std :: vector,但由于可能要删除很多东西,因此性能可能会受到影响。 对于大量数据集,这可能会引发大规模的改组。 在这些情况下,最好使用std :: list。
您正在执行的操作表明您的容器类型错误。
multimap
已经完成1,因此您无需对键进行排序(“第一个数组”。)鉴于multimap<int, int> arrays
2可以像这样完成:
multimap<int, int> newarrays;
auto it = begin(arrays);
while(it != end(arrays)) {
if(it->second != 0) {
++it;
} else {
newArrays.insert(*it);
it = arrays.erase(it);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.