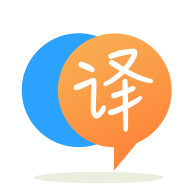
[英]How to consume web socket messages in javascript using stomp.js?
[英]How to plot, in Javascript and on a D3.js line graph, real time time data coming from web socket messages via stomp.js?
使用Java语言,我需要调用Web套接字(使用stomp.js ...),并在D3.js折线图上绘制实时数据。
这是我的实际代码...
<!DOCTYPE HTML>
<html>
<head>
<title>Client Stomp 1</title>
<style>
body { font: 12px Arial;}
path {
stroke: steelblue;
stroke-width: 2;
fill: none;
}
.axis path,
.axis line {
fill: none;
stroke: grey;
stroke-width: 1;
shape-rendering: crispEdges;
}
</style>
<script src="https://cdnjs.cloudflare.com/ajax/libs/stomp.js/2.3.3/stomp.min.js"></script>
<!-- *** D3.js library inclusion ... *** -->
<script src="http://d3js.org/d3.v3.min.js"></script>
</head>
<body>
<script>
var urlServer;
var topics;
// *** Service variable to interrupt te values visualization ...
var i = 0;
// *** The vector that will mantain the couple values data - temperatura that there will be plotted in the line graph ...
var dataLineGraph = [];
// *** Setting the server and topic parameters ...
urlServer = "ws://stream.smartdatanet.it/ws";
topics = "/topic/output.arpa_rumore.4c5d7481-fa5e-4f2f-d26d-d4e8095b9dd2_s_01";
// *** Create the stomp client and estabilish the connection ...
// *** username and password are public
client = Stomp.client(urlServer);
client.connect("guest" , "Aekieh6F" , connectCallBack, errorCallback);
plotLineGraph (dataLineGraph);
// *** Manage the connection ...
function connectCallBack(x) {
client.subscribe(topics, messageCallback);
if (i < 1) {alert("Make the connection !")}
i = i + 1;
}
// *** Manage the connection error...
function errorCallback(x) {
if (i < 1) {alert("Connection error !")}
i = i + 1;
}
// *** Manage the message callback...
function messageCallback(x) {
if (i < 5) {
alert("First messages sent form the platform! To see the others open the web browser console !!!");
// *** Convert the body message in JSON format ...
var theJson = JSON.parse(x.body);
// *** Alert the time and the b_6300_Hz value ...
//alert(theJson.values[0].time + " - " + theJson.values[0].components.b_6300_Hz);
// *** Alert the time and the b_6300_Hz value ...
time = theJson.values[0].time;
// *** Hardcoded but it's only to test the date parsing ...
day = time.substring(8,10);
//alert ("Day = " + day);
month = time.substring(5,7);
//alert ("month = " + month);
year = time.substring(0,4);
//alert ("Year = " + year);
hour = time.substring(11,13);
//alert ("Hour = " + hour);
minutes = time.substring(14,16);
//alert ("Minutes = " + minutes);
seconds = time.substring(17,19);
//alert ("Seconds= " + seconds);
// formattedDate = (dt.getMonth() + 1) + "/" + dt.getDate() + "/" + dt.getFullYear() + "-" + (dt.getHours()) + ":" + dt.getMinutes() + ":" + dt.getSeconds();
formattedDate = month + "/" + day + "/" + year + "-" + hour + ":" + minutes + ":" + seconds;
value = theJson.values[0].components.b_6300_Hz;
// 2016-07-01T15:18:31+0200 - 50.2
alert ("formattedDate = " + formattedDate);
dataLineGraph.push({time: formattedDate, value:value});
}
i = i + 1;
}
// *** Function for to draw the line graph ...
function plotLineGraph (data) {
// Set the dimensions of the canvas / graph
var margin = {top: 30, right: 20, bottom: 30, left: 50},
width = 600 - margin.left - margin.right,
height = 270 - margin.top - margin.bottom;
// Set the ranges
var x = d3.time.scale().range([0, width]);
var y = d3.scale.linear().range([height, 0]);
// Define the axes
var xAxis = d3.svg.axis().scale(x).orient("bottom").ticks(5);
var yAxis = d3.svg.axis().scale(y).orient("left").ticks(5);
// Define the line
var valueline = d3.svg.line()
.x(function(d) { return x(d.date); })
.y(function(d) { return y(d.close); });
// Adds the svg canvas
var svg = d3.select("body")
.append("svg")
.attr("width", width + margin.left + margin.right)
.attr("height", height + margin.top + margin.bottom)
.append("g")
.attr("transform",
"translate(" + margin.left + "," + margin.top + ")");
// Parse the date / time
var parseDate = d3.time.format("%d/%m/%Y-%H:%M:%S").parse;
// Get the data
// d3.csv("data.csv", function(error, data) {
data.forEach(function(d) {
d.date = parseDate(d.time);
d.close = +d.value;
});
// Scale the range of the data
x.domain(d3.extent(data, function(d) { return d.date; }));
y.domain([0, d3.max(data, function(d) { return d.value; })]);
// Add the valueline path.
svg.append("path")
.attr("class", "line")
.attr("d", valueline(data));
// Add the X Axis
svg.append("g")
.attr("class", "x axis")
.attr("transform", "translate(0," + height + ")")
.call(xAxis);
// Add the Y Axis
svg.append("g")
.attr("class", "y axis")
.call(yAxis);
// });
}
</script>
</body>
</html>
您可以复制/粘贴并尝试执行(...应该工作...)。
您可以从警报中看到消息,也可以通过Web浏览器控制台查看消息,从而可以使用流中的消息,但是我不知道如何将这些信息放在动态折线图上,例如http://jsfiddle.net/ peDzT / 。
如何在折线图的dataLineGraph数组中添加数据,以及在向量中添加了每个新数据后如何刷新数据?
请注意,如果我将“ plotLineGraph”功能与一次加载的“静态”数据一起使用,则可以使用。
任何建议/示例将不胜感激!
切萨雷
我已经整理好代码并将其重构,以向您展示示例实现。 在其中绘制时间与b_400_Hz的关系,因为我不确定您要跟踪哪个信号。 我已经很好地注释了下面的代码,并且它可以运行,因此,请问您是否有任何问题:
<!DOCTYPE HTML> <html> <head> <title>Client Stomp 1</title> <style> body { font: 12px Arial; } path { stroke: steelblue; stroke-width: 2; fill: none; } .axis path, .axis line { fill: none; stroke: grey; stroke-width: 1; shape-rendering: crispEdges; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/stomp.js/2.3.3/stomp.min.js"></script> <!-- *** D3.js library inclusion ... *** --> <script src="https://d3js.org/d3.v3.min.js"></script> </head> <body> <script> var urlServer; var topics; // *** The vector that will mantain the couple values data - temperatura that there will be plotted in the line graph ... var dataLineGraph = []; // *** Setting the server and topic parameters ... urlServer = "ws://stream.smartdatanet.it/ws"; topics = "/topic/output.arpa_rumore.4c5d7481-fa5e-4f2f-d26d-d4e8095b9dd2_s_01"; // *** Create the stomp client and estabilish the connection ... // *** username and password are public client = Stomp.client(urlServer); client.connect("guest", "Aekieh6F", connectCallBack, function(){}); // *** Manage the connection ... function connectCallBack(x) { client.subscribe(topics, messageCallback); } function compare(a,b) { if (a.time < b.time) return -1; if (a.time > b.time) return 1; return 0; } // parse the times from the server var parseDate = d3.time.format("%Y-%m-%dT%H:%M:%S%Z").parse; // *** Manage the message callback... function messageCallback(x) { // *** Convert the body message in JSON format ... var theJson = JSON.parse(x.body); // grab the values of interest dataLineGraph.push({ time: parseDate(theJson.values[0].time), value: +theJson.values[0].components.b_400_Hz }); // make sure we stay sorted dataLineGraph.sort(compare); plotLineGraph(); } // intial graph setup setupGraph(); // keep reference to these variables from setupGraph var valueline, x, y, xAxisG, yAxisG, line; function setupGraph() { // Set the dimensions of the canvas / graph var margin = { top: 30, right: 20, bottom: 30, left: 50 }, width = 600 - margin.left - margin.right, height = 270 - margin.top - margin.bottom; // Set the ranges x = d3.time.scale().range([0, width]); y = d3.scale.linear().range([height, 0]); // Define the line valueline = d3.svg.line() .x(function(d) { console.log(d) return x(d.time); }) .y(function(d) { return y(d.value); }); // Adds the svg canvas var svg = d3.select("body") .append("svg") .attr("width", width + margin.left + margin.right) .attr("height", height + margin.top + margin.bottom) .append("g") .attr("transform", "translate(" + margin.left + "," + margin.top + ")"); // Add the X Axis xAxisG = svg.append("g") .attr("class", "x axis") .attr("transform", "translate(0," + height + ")"); // Add the Y Axis yAxisG = svg.append("g") .attr("class", "y axis"); line = svg.append("path"); } // *** Function for to draw the line graph ... function plotLineGraph() { // Scale the range of the data x.domain(d3.extent(dataLineGraph, function(d) { return d.time; })); y.domain([0, d3.max(dataLineGraph, function(d) { return d.value; })]); // transistion axis xAxisG .transition() .duration(500) .ease("linear") .call(d3.svg.axis().scale(x).orient("bottom")); yAxisG .transition() .duration(500) .ease("linear") .call(d3.svg.axis().scale(y).orient("left")); // change the line line.transition() .duration(500) .attr("d", valueline(dataLineGraph)); } </script> </body> </html>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.