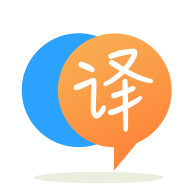
[英]Typescript: Why is it that we cannot assign default value to generic type?
[英]Default value of Type in Generic classes in Typescript
我需要根据下面的Typescript泛型类中的类型设置变量的默认值
class MyClass<T>{
myvariable: T // Here I want to set the value of this variable
// with the default value of the type passed in 'T'
}
例如,如果T是数字,则变量myvariable
的默认值应为“0”,类似于字符串,它应为空字符串,依此类推。
你不能这样做,因为T
的实际类型只能在运行时知道。
你可以做什么:
abstract class MyClass<T> {
myvariable: T;
constructor() {
this.myvariable = this.getInitialValue();
}
protected abstract getInitialValue(): T;
}
现在你只需扩展这个类,如下所示:
class MyStringClass extends MyClass<string> {
protected getInitialValue(): string {
return "init-value";
}
}
你要求的是无法完成的,因为T
只存在于typescript领域,并且它不会在编译过程中“存活”。
例如,这个:
class MyClass<T> {
myvariable: T;
constructor(value: T) {
this.myvariable = value;
}
}
编译成:
var MyClass = (function () {
function MyClass(value) {
this.myvariable = value;
}
return MyClass;
}());
如您所见,在编译版本中没有T
,因此您无法在运行时使用该信息来生成默认值。
另一个解决方案是拥有默认值的映射:
var defaultValues = {
"string": "",
"number": 0,
"boolean": false
}
class MyClass<T> {
myvariable: T;
constructor(value: T) {
this.myvariable = value;
}
}
let a = new MyClass<string>(defaultValues.string);
let b = new MyClass<boolean>(defaultValues.boolean);
您还可以使用静态工厂方法:
class MyClass<T> {
myvariable: T;
constructor(value: T) {
this.myvariable = value;
}
static stringInstance(): MyClass<string> {
return new MyClass<string>("");
}
static numberInstance(): MyClass<number> {
return new MyClass<number>(0);
}
static booleanInstance(): MyClass<boolean> {
return new MyClass<boolean>(false);
}
}
let a = MyClass.stringInstance();
let b = MyClass.booleanInstance();
T
在运行时丢失,因此您需要传递类似值的类型。 你可以通过传递构造函数来做到这一点。
当我有类似需求时,我通常使用这样的界面:
interface Type<T> {
new() : T;
}
并像这样创建MyClass:
class MyClass<T>{
myvariable: T;
constructor(type: Type<T>) {
this.myvariable = new type();
}
}
然后我可以这样使用MyClass:
let myinstance = new MyClass(TheOtherType);
它适用于类,但不适用于字符串和数字等内置函数。
TheOtherType
是类的构造函数,例如:
class TheOtherType {
}
嗯,我想我想出了一个可能的解决方案。 无论如何,我必须说Nitzan Tomer是正确的,并且类型在运行时不可用。 这是TS的搞笑一面:)
在这里,您可以检查所获对象的类型,然后设置默认值。 您也可以更改地点和要检查的对象,以便按照您想要的方式进行检查,但我想这对您来说可能是一个很好的起点。 请注意,您必须进行双重转换,因为TS无法保证类型兼容。 我还没有尝试过,但编译得很好
class MyClass<T>{
myvariable: T
constructor(param: any) {
if (typeof param === 'string') {
this.myvariable = <T><any> "";
}
else if (typeof param === 'number') {
this.myvariable = <T><any> 0;
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.