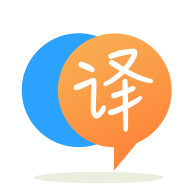
[英]How to store different objects of arrayList in memory and later retrieve them?
[英]How to store a list of objects to memory for later use
我正在尝试编写一个程序,将有助于管理一些数据。 到目前为止,用户可以使用“新文件”对话框创建新文件。 用户输入一些参数后,我将创建“文件”类的新实例。 我现在的问题是:如何在内存中存储多个文件(如选项卡)。 我正在考虑使用某种ArrayList作为“应用程序”类中的变量。 在这里什么是理想的选择?
是的,您只需使用List
即可存储实例
int maxfiles = 150; //You can set the length of the list, by default is 10
List<File> files = new ArrayList<File>(maxfiles);
//Your multiple files here
File file1 = null;
File file2 = null;
File file3 = null;
//Simply add it to the List
files.add(file1);
files.add(file2);
files.add(file3);
//And remove it by
files.remove(0); //Index
//Or remove all
files.clear();
您可以使用地图将文件存储在内存中。
public class FileStorage{
Map<String, File> fileMap;
public FileStorage(){
fileMap = new HashMap<String, File>();
}
public void addNewFile(File f, String fileName){
if (fileMap.get(fileName) != null){
// Do something if you do not want to erase previous file...
else{
fileMap.put(fileName, f);
}
}
public File getStoredFile(String fileName){
File f = fileMap.get(fileName);
if (f==null){
// alert user the file is not found.
}
return f;
}
}
然后在您的主类中,您可以简单地使用FileStorage类来管理文件
public static void main(String[] args){
FileStorage fileStorage = new FileStorage();
// User create a new file
File file1 = new File();
fileStorage.addNewFile(file1, "file1"); // name can also be file1.getName()
[...]
// Later you can access the file
File storedFile = fileStorage.getStoredFile("file1");
}
访问或存储文件的复杂度为O(1)。 它比访问文件列表要好。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.