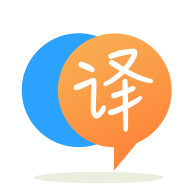
[英]How do I get the name of the current executable in C#? (.NET 5 edition)
[英]How do I get the name of the current executable in C#?
我想获取当前正在运行的程序的名称,即程序的可执行名称。 在 C/C++ 中,您可以从args[0]
获得它。
System.AppDomain.CurrentDomain.FriendlyName
System.AppDomain.CurrentDomain.FriendlyName
- 返回带有扩展名的文件名(例如 MyApp.exe)。
System.Diagnostics.Process.GetCurrentProcess().ProcessName
- 返回不带扩展名的文件名(例如 MyApp)。
System.Diagnostics.Process.GetCurrentProcess().MainModule.FileName
- 返回完整路径和文件名(例如 C:\Examples\Processes\MyApp.exe)。 然后,您可以将其传递给System.IO.Path.GetFileName()
或System.IO.Path.GetFileNameWithoutExtension()
以获得与上述相同的结果。
这应该足够了:
Environment.GetCommandLineArgs()[0];
System.Diagnostics.Process.GetCurrentProcess()
获取当前运行的进程。 您可以使用ProcessName
属性来确定名称。 下面是一个示例控制台应用程序。
using System;
using System.Diagnostics;
class Program
{
static void Main(string[] args)
{
Console.WriteLine(Process.GetCurrentProcess().ProcessName);
Console.ReadLine();
}
}
这是对我有用的代码:
string fullName = Assembly.GetEntryAssembly().Location;
string myName = Path.GetFileNameWithoutExtension(fullName);
上面的所有示例都给了我带有 vshost 的 processName 或正在运行的 dll 名称。
尝试这个:
System.Reflection.Assembly.GetExecutingAssembly()
这将为您返回一个System.Reflection.Assembly
实例,其中包含您可能想知道的有关当前应用程序的所有数据。 我认为Location
属性可能会得到你想要的东西。
为什么没有人建议这个,很简单。
Path.GetFileName(Application.ExecutablePath)
System.Reflection.Assembly.GetExecutingAssembly().ManifestModule.Name;
会给你你的应用程序的文件名,比如; “我的应用程序.exe”
如果您需要程序名称来设置防火墙规则,请使用:
System.Diagnostics.Process.GetCurrentProcess().MainModule.FileName
这将确保在 VisualStudio 中调试和直接在 windows 中运行应用程序时名称正确。
更多选择:
System.Reflection.Assembly.GetExecutingAssembly().GetName().Name
Path.GetFileName(System.Reflection.Assembly.GetExecutingAssembly().GetName().CodeBase
System.Reflection.Assembly.GetEntryAssembly().Location
将返回 exe 名称的位置。System.Reflection.Assembly.GetEntryAssembly().CodeBase
将位置返回为 URL。当不确定或有疑问时,绕圈跑,尖叫和喊叫。
class Ourself
{
public static string OurFileName() {
System.Reflection.Assembly _objParentAssembly;
if (System.Reflection.Assembly.GetEntryAssembly() == null)
_objParentAssembly = System.Reflection.Assembly.GetCallingAssembly();
else
_objParentAssembly = System.Reflection.Assembly.GetEntryAssembly();
if (_objParentAssembly.CodeBase.StartsWith("http://"))
throw new System.IO.IOException("Deployed from URL");
if (System.IO.File.Exists(_objParentAssembly.Location))
return _objParentAssembly.Location;
if (System.IO.File.Exists(System.AppDomain.CurrentDomain.BaseDirectory + System.AppDomain.CurrentDomain.FriendlyName))
return System.AppDomain.CurrentDomain.BaseDirectory + System.AppDomain.CurrentDomain.FriendlyName;
if (System.IO.File.Exists(System.Reflection.Assembly.GetExecutingAssembly().Location))
return System.Reflection.Assembly.GetExecutingAssembly().Location;
throw new System.IO.IOException("Assembly not found");
}
}
我不能声称已经测试了每个选项,但它不会做任何愚蠢的事情,比如在调试会话期间返回虚拟主机。
如果您正在寻找可执行文件的完整路径信息,可靠的方法是使用以下内容:
var executable = System.Diagnostics.Process.GetCurrentProcess().MainModule
.FileName.Replace(".vshost", "");
这消除了中间 dll、vshost 等的任何问题。
您可以使用Environment.GetCommandLineArgs()
获取 arguments 和Environment.CommandLine
获取输入的实际命令行。
此外,您可以使用Assembly.GetEntryAssembly()
或Process.GetCurrentProcess()
。
但是,在调试时,您应该小心,因为最后一个示例可能会给出调试器的可执行文件名称(取决于您附加调试器的方式),而不是您的可执行文件,就像其他示例一样。
如果您只需要没有扩展名的应用程序名称,则此方法有效:
Path.GetFileNameWithoutExtension(AppDomain.CurrentDomain.FriendlyName);
超级简单,看这里:
Environment.CurrentDirectory + "\\" + Process.GetCurrentProcess().ProcessName
On.Net Core(或 Mono),当定义进程的二进制文件是 Mono 或 .Net Core(dotnet)的运行时二进制文件而不是您感兴趣的实际应用程序时,大多数答案都不适用。在这种情况下, 用这个:
var myName = Path.GetFileNameWithoutExtension(System.Reflection.Assembly.GetEntryAssembly().Location);
对于 windows 应用程序(表单和控制台)我使用这个:
在VS中添加对System.Windows.Forms的引用,然后:
using System.Windows.Forms;
namespace whatever
{
class Program
{
static string ApplicationName = Application.ProductName.ToString();
static void Main(string[] args)
{
........
}
}
}
无论我是在 VS 中运行实际的可执行文件还是调试,这对我来说都是正确的。
请注意,它返回不带扩展名的应用程序名称。
约翰
这是你想要的吗:
Assembly.GetExecutingAssembly ().Location
如果您在 net6.0 或更高版本中发布单个文件应用程序,您可以使用Environment.ProcessPath()
尝试 Application.ExecutablePath
获取路径和名称
System.Diagnostics.Process.GetCurrentProcess().MainModule.FileName
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.