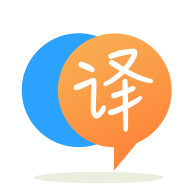
[英]How to call a rest API which returns object of two different types
[英]How do I write my REST API call when expecting two types of return Objects
我使用Spring编写了一个REST端点。
@RequestMapping(value = "/staticdata/userPreference/extUserId/{extUserId}/type/{type}", method = RequestMethod.GET)
public ResponseEntity<List<UserPreference>> getData(
@PathVariable String extUserId,
@PathVariable String type) {
List<UserPreference> userPreferences = null;
userPreferences = staticDataService.getUserPreference(extUserId, type);
return new ResponseEntity<List<UserPreference>>(userPreferences, HttpStatus.OK);
}
@ExceptionHandler(RuntimeException.class)
public ResponseEntity<String> handleError(HttpServletRequest req, Exception exception) {
return new ResponseEntity<String>(exception.getMessage(), HttpStatus.INTERNAL_SERVER_ERROR);
}
当getData()方法能够成功获取数据时,它需要返回List <>。 但是当有例外时。 将返回该消息。 这由handleError()方法处理。 此方法返回一个String。
我的问题是:对这个休息服务的调用应该如何使用Spring提供的restTemplate? 根据失败成功,方法的返回可以是List或String。
您可以尝试使用RestTemplate#exchange
来执行请求并将响应作为ResponseEntity
获取,然后通过ResponseEntity#getBody
获取List<UserPreference>
。
如果有错误,您可以从HttpStatusCodeException.getResponseBodyAsString
获取错误响应。
以下是一个例子:
try {
HttpHeaders headers = new HttpHeaders();
// add some headers
HttpEntity<String> request = new HttpEntity<String>(headers);
ResponseEntity<List<UserPreference>> response = restTemplate.exchange(
YOUR_URL, HttpMethod.GET, request, new ParameterizedTypeReference<List<UserPreference>>() {});
List<UserPreference> userPerference = response.getBody();
} catch (HttpStatusCodeException e) {
String errorBody = e.getResponseBodyAsString();
}
如果您需要对错误进行更多特殊处理,可以尝试实现自定义ResponseErrorHandler
。
编辑 :
以下是演示的代码示例:
测试RestController
有一个RequestMapping
,如果请求路径为“/ test?error = true”,它将抛出异常。 否则,返回List<String>
响应:
@RestController
public class TestController {
@RequestMapping(value = "/test", method = RequestMethod.GET)
public ResponseEntity<List<String>> getData(@RequestParam(value = "error", required = false) String error) {
if (error != null && error.equals("true")) {
throw new HttpClientErrorException(HttpStatus.NOT_FOUND);
} else {
List<String> list = new ArrayList<>();
list.add("test");
return new ResponseEntity<List<String>>(list, HttpStatus.OK);
}
}
@ExceptionHandler(RuntimeException.class)
public ResponseEntity<String> handleError(HttpServletRequest req, Exception exception) {
return new ResponseEntity<String>("Exception Message", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
测试配置:
@SpringBootApplication
public class TestConfig {
}
集成测试有2个测试用例。 一种是测试获取List响应,另一种是测试从错误响应中获取消息体:
@RunWith(SpringJUnit4ClassRunner.class)
@SpringApplicationConfiguration(classes = TestConfig.class)
@WebIntegrationTest
public class IntegrationTest {
@Value("${local.server.port}")
int port;
final RestTemplate template = new RestTemplate();
@Test
public void testGetListResponse() {
String url = "http://localhost:" + port + "/test";
ResponseEntity<List<String>> response = template.exchange(
url, HttpMethod.GET, null, new ParameterizedTypeReference<List<String>>() {});
List<String> list = response.getBody();
Assert.assertEquals(list.size(), 1);
Assert.assertEquals(list.get(0), "test");
}
@Test
public void testGetErrorResponse() {
String url = "http://localhost:" + port + "/test?error=true";
String errorBody = null;
try {
ResponseEntity<List<String>> response = template.exchange(
url, HttpMethod.GET, null, new ParameterizedTypeReference<List<String>>() {
});
} catch (HttpStatusCodeException e) {
errorBody = e.getResponseBodyAsString();
}
Assert.assertEquals(errorBody, "Exception Message");
}
}
希望这可以提供帮助。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.