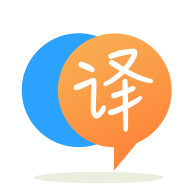
[英]How can I create a (C) function to read data from a file into a linked-list using 'read'?
[英]How can I read a file and determine its data representation as I pass it into a linked list?
因此,在这段代码中,我试图获取一个文本文件,获取所有字符串标记,并使添加到链接列表中的节点成为可能。 我能够从中获取每个单独的字符串标记,并正确地填写“数据”字段,以创建一个节点。 当我尝试使用确定的函数填充“类型”字段时,问题就来了。 我知道在这段代码中只有八进制的一次检查,但是即使在那之前,我的gcc编译器仍在告诉我,我不理解对指针/字符的强制转换。 我确定的函数中我缺少什么或做错了什么? 我觉得参数等可能也不正确,但是我不确定如何...
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node {
char *data;
char *type;
struct node *next;
} node;
void readfile(char* filename) {
FILE *file = fopen(filename, "r");
if(file == NULL) {
exit(1);
}
char buffer[51];
while(fscanf(file, "%s", buffer) != EOF) {
add(buffer);
}
fclose(file);
}
void add(char* line) {
node *temp = malloc(sizeof(node));
temp->data = strdup(line);
temp->type = determine(line);
temp->next = NULL;
current = start;
if(start == NULL) {
start = temp;
} else {
while(current->next != NULL) {
current = current->next;
}
current->next = temp;
}
}
char* determineDOHF(node* line){
/*supposed to determine whether the string is represented as
decimal, octal, hex, or floating point*/
char *dohfType;
char input[51] = line;
if(input[0] == '0'){
dohfType = 'Octal';
}
return dohfType;
}
3件事:
在您的add函数中,最好在执行malloc之后始终释放内存。 Malloc将为您的程序分配内存,只有在您手动执行或关闭程序后才会释放该内存。
此外,在determinedohf
您将返回指向该函数局部变量的指针。 函数返回后,该内存位置可能无效,并且您将读取垃圾值。 如果要使用该返回的字符串,则要使用malloc分配input
字符串。 我强烈建议您用整数代替类型,并使用枚举来提高可读性。
枚举类型{OCTAL = 0,DECIMAL,HEX}
您正在将node *
的类型分配给char
类型,这是无效的分配。 我怀疑您想使用node *
的类型元素。 因此,您应该执行以下操作:
strcpy(输入,链接->类型)
在C语言中,您不能仅在变量初始化后分配字符串。 您需要使用strcpy这样做。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.