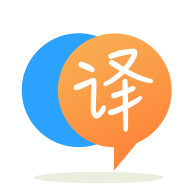
[英]How to delete a specific user in a list from firebase in flutter
[英]Delete a specific user from Firebase
有没有办法从 firebase 获取特定用户帐户,然后将其删除?
例如:
// I need a means of getting a specific auth user.
var user = firebase.auth().getUser(uid);
// Note the getUser function is not an actual function.
之后,我想删除该用户及其附加数据:
// This works
user.delete().then(function() {
// User deleted.
var ref = firebase.database().ref(
"users/".concat(user.uid, "/")
);
ref.remove();
});
Firebase 文档指出,如果用户当前已登录,则可以将其删除:
firebase.auth().currentUser.delete()
我的目标是允许登录的管理员用户从系统中删除其他用户。
使用客户端 SDK 进行 Firebase 身份验证时,您只能删除当前登录的用户帐户。其他任何事情都会带来巨大的安全风险,因为它会允许您的应用程序用户删除彼此的帐户。
Firebase 身份验证的Admin SDK旨在用于受信任的环境,例如您的开发机器、您控制的服务器或 Cloud Functions。 因为它们运行在受信任的环境中,所以它们可以执行某些客户端 SDK 无法执行的操作,例如只需知道用户的 UID 即可删除用户帐户。
另见:
另一种常见的方法是在 Firebase 数据库中保留一个白名单/黑名单,并根据它授权用户。 请参阅如何在 Firebase 3.x 中禁用注册
我知道这是一个老问题,但我找到了另一个解决方案。 您绝对不想在您的应用程序本身中使用 firebase-admin,正如我认为 Ali Haider 所建议的那样,因为它需要一个您不想与您的代码一起部署的私钥。
但是,您可以在 Firebase 中创建一个 Cloud Functions 函数,该函数在您的 Firestore 或实时数据库中删除用户时触发,并让该 Cloud Functions 函数使用 firebase-admin 删除用户。 就我而言,我的 Firestore 中有一组用户,其用户 ID 与 Firebase Auth 创建的用户 ID 相同,我在其中保存了额外的用户数据,例如名称和角色等。
如果您像我一样使用 Firestore,则可以执行以下操作。 如果您使用的是实时数据库,只需在文档中查找如何为此使用触发器。
确保您的 Firebase 项目已初始化云函数。 在您的项目目录中应该有一个名为“functions”的文件夹。 如果不是:使用以下命令为您的项目初始化 Cloud Functions: firebase init functions
。
在以下页面的 Firebase 控制台中为您的服务帐户获取私钥:设置 > 服务帐户。
将包含私钥的 json 文件放在 index.ts 文件旁边的functions\\src
文件夹中。
在 index.ts 中导出以下函数:
export const removeUser = functions.firestore.document("/users/{uid}")
.onDelete((snapshot, context) => {
const serviceAccount = require('path/to/serviceAccountKey.json');
admin.initializeApp({
credential: admin.credential.cert(serviceAccount),
databaseURL: "https://<DATABASE_NAME>>.firebaseio.com"
});
return admin.auth().deleteUser(context.params.uid);
});
firebase deploy --only functions
部署您的云firebase deploy --only functions
当用户在 Firebase Firestore 中被删除时,此代码将运行并从 Firebase Auth 中删除该用户。
有关 Firebase Cloud Functions 的更多信息,请参阅https://firebase.google.com/docs/functions/get-started
只需以与您进行身份验证相同的方式应用此代码。
var user = firebase.auth().currentUser;
user.delete().then(function() {
// User deleted.
}).catch(function(error) {
// An error happened.
});
就像这个答案指出的用户登录一样,必须创建第二个应用程序才能删除登录用户以外的其他用户。
我是这样做的:
async deleteUser (user) {
// Need to create a second app to delete another user in Firebase auth list than the logged in one.
// https://stackoverflow.com/a/38013551/2012407
const secondaryApp = firebase.initializeApp(config, 'Secondary')
if (!user.email || !user.password) {
return console.warn('Missing email or password to delete the user.')
}
await secondaryApp.auth().signInWithEmailAndPassword(user.email, user.password)
.then(() => {
const userInFirebaseAuth = secondaryApp.auth().currentUser
userInFirebaseAuth.delete() // Delete the user in Firebase auth list (has to be logged in).
secondaryApp.auth().signOut()
secondaryApp.delete()
// Then you can delete the user from the users collection if you have one.
})
}
在我看来,您可以在没有 Firebase Admin SDK 的情况下删除特定用户。 您必须存储要管理的帐户的用户名、密码。 并使用帐户登录 - 您声明了一个管理员帐户。 之后只需按照以下步骤操作:使用 firebase auth 注销 -> 使用 firebase auth 使用要删除的帐户登录 -> 使用 firebase auth 删除该帐户 -> 使用 firebase auth 注销 -> 再次使用该“管理员帐户”登录. 希望此解决方案可以帮助您在不使用 Firebase Admin SDK 的情况下删除帐户
npm i --save firebase-admin
从“firebase-admin”导入 * 作为管理员
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.