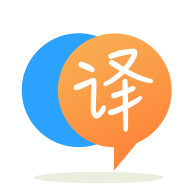
[英]Invalid conversion from ‘const char*’ to ‘unsigned char*’
[英]C++ invalid conversion from ‘char*’ to ‘const unsigned char*’
我正在用NodeJS编写项目,但是我不得不使用C共享库。 因此,我必须使用Node.js插件。 调用C ++代码效果很好,我还能够加载自定义库,并成功调用了两个C函数:一个给我库的版本号,另一种方法根据JS提供的数据生成密钥。
我遇到的问题与生成的密钥有关。 我必须在encrypt
和decrypt
方法中使用。
这是我用来存储密钥的变量:
unsigned char generated_key[256];
这是生成密钥的函数:
extern "C"
{
#ifdef WIN32
VE_EXPORT int CALLBACK generate_key
#else
int generate_key
#endif
(char *variable_msg, /*!< variable seed */
char *shared_seed, /*!< shared seed */
unsigned char *generated_key, /*!< generated key */
unsigned int key_size); /*!< key size */
}
这是使用键的功能:
extern "C"
{
#ifdef WIN32
VE_EXPORT int CALLBACK encrypt
#else
int encrypt
#endif
(const unsigned char * const plain_data, /*!< plain data */
const unsigned int plain_data_len, /*!< number bytes to be encrypted */
unsigned char *encrypted_data, /*!< encrypted data */
const unsigned int encrypted_data_size, /*!< size encrypted_data array */
unsigned int *encrypted_data_len, /*!< number of bytes encrypted */
const unsigned char * const key /*!< symmetric encryption key */
);
}
当我尝试编译代码时,我得到:
gyp info it worked if it ends with ok
gyp info using node-gyp@3.4.0
gyp info using node@6.2.0 | linux | x64
gyp info spawn /usr/bin/python2
gyp info spawn args [ '/usr/local/lib/node_modules/node-gyp/gyp/gyp_main.py',
gyp info spawn args 'binding.gyp',
gyp info spawn args '-f',
gyp info spawn args 'make',
gyp info spawn args '-I',
gyp info spawn args '/home/dgatti/sequr/sequr-experiments/CPP/Sequr/build/config.gypi',
gyp info spawn args '-I',
gyp info spawn args '/usr/local/lib/node_modules/node-gyp/addon.gypi',
gyp info spawn args '-I',
gyp info spawn args '/home/dgatti/.node-gyp/6.2.0/include/node/common.gypi',
gyp info spawn args '-Dlibrary=shared_library',
gyp info spawn args '-Dvisibility=default',
gyp info spawn args '-Dnode_root_dir=/home/dgatti/.node-gyp/6.2.0',
gyp info spawn args '-Dnode_gyp_dir=/usr/local/lib/node_modules/node-gyp',
gyp info spawn args '-Dnode_lib_file=node.lib',
gyp info spawn args '-Dmodule_root_dir=/home/dgatti/sequr/sequr-experiments/CPP/Sequr',
gyp info spawn args '--depth=.',
gyp info spawn args '--no-parallel',
gyp info spawn args '--generator-output',
gyp info spawn args 'build',
gyp info spawn args '-Goutput_dir=.' ]
make: Entering directory `/home/dgatti/sequr/sequr-experiments/CPP/Sequr/build'
gyp info spawn make
gyp info spawn args [ 'BUILDTYPE=Release', '-C', 'build' ]
CXX(target) Release/obj.target/vertx/main.o
../main.cpp: In function ‘void demo::VertxEncrypt(const v8::FunctionCallbackInfo<v8::Value>&)’:
../main.cpp:127:102: error: invalid conversion from ‘char*’ to ‘const unsigned char*’ [-fpermissive]
vertx_encrypt(cPayload, sizeof(cPayload), encMessage, sizeof(encMessage), &encLength, generated_key);
^
In file included from ../main.cpp:3:0:
../libvertx_encryption.h:79:7: error: initializing argument 1 of ‘int vertx_encrypt(const unsigned char*, unsigned int, unsigned char*, unsigned int, unsigned int*, const unsigned char*)’ [-fpermissive]
int vertx_encrypt
^
make: *** [Release/obj.target/vertx/main.o] Error 1
make: Leaving directory `/home/dgatti/sequr/sequr-experiments/CPP/Sequr/build'
gyp ERR! build error
gyp ERR! stack Error: `make` failed with exit code: 2
gyp ERR! stack at ChildProcess.onExit (/usr/local/lib/node_modules/node-gyp/lib/build.js:276:23)
gyp ERR! stack at emitTwo (events.js:106:13)
gyp ERR! stack at ChildProcess.emit (events.js:191:7)
gyp ERR! stack at Process.ChildProcess._handle.onexit (internal/child_process.js:204:12)
gyp ERR! System Linux 3.10.0-327.13.1.el7.x86_64
gyp ERR! command "/usr/local/bin/node" "/usr/local/bin/node-gyp" "rebuild"
gyp ERR! cwd /home/dgatti/sequr/sequr-experiments/CPP/Sequr
gyp ERR! node -v v6.2.0
gyp ERR! node-gyp -v v3.4.0
gyp ERR! not ok
这就是我调用函数的方式,现在我只是按原样传递变量,但是我尝试了许多不同的转换,转换和更改方式。
encrypt(cPayload, sizeof(cPayload), encMessage, sizeof(encMessage), &encLength, generated_key);
我用C语言编写了一个示例,一切正常,但是当我尝试在C ++中使用此C函数时,事情就刹车了。 我有C方面的经验,但绝对没有C ++。 我花了几天的时间找到解决方案,但Google叔叔和哥们Stack没办法提出解决方案。
这是我从NodeJS调用以访问C函数的C ++中的完整代码:
#include <node.h>
#include <iostream>
#include "lib_encryption.h"
using namespace std;
namespace demo {
using v8::Exception;
using v8::FunctionCallbackInfo;
using v8::Isolate;
using v8::Local;
using v8::Number;
using v8::Object;
using v8::String;
using v8::Value;
unsigned char generated_key[256];
unsigned int key_size = 32;
unsigned int encLength;
unsigned char encMessage[256] = {0};
//
// This is the implementation of the "add" method
// Input arguments are passed using the
// const FunctionCallbackInfo<Value>& args struct
//
void GenerateKey(const FunctionCallbackInfo<Value>& args)
{
v8::String::Utf8Value param1(args[0]->ToString());
std::string strMessage = std::string(*param1);
v8::String::Utf8Value param2(args[1]->ToString());
std::string strSecret = std::string(*param2);
char cMessage[4];
strcpy(cMessage, strMessage.c_str());
char cSecret[11];
strcpy(cSecret, strSecret.c_str());
//
// Creating the key
//
_generate_key(cMessage, cSecret, generated_key, key_size);
//
// 1. Create isolation storage
//
Isolate* isolate = args.GetIsolate();
//
// 2. Save the value in to a isolate storage
//
Local<Value> str = String::NewFromUtf8(isolate, "Key done");
//
// 3. Set the return value (using the passed in
// FunctionCallbackInfo<Value>&)
//
args.GetReturnValue().Set(str);
}
void Encrypt(const FunctionCallbackInfo<Value>& args)
{
Isolate* isolate = args.GetIsolate();
v8::String::Utf8Value param1(args[0]->ToString());
std::string payload = std::string(*param1);
char cPayload[4];
strcpy(cPayload, payload.c_str());
for (int i=0; i<256; i++)
{
printf("%.2X ", generated_key[i]);
}
printf("\n");
encrypt(cPayload, sizeof(cPayload), encMessage, sizeof(encMessage), &encLength, generated_key);
//
// 1. Create isolation storage
//
//
// 2. Save the value in to a isolate storage
//
Local<Value> str = String::NewFromUtf8(isolate, "Encrypt");
//
// 3. Set the return value (using the passed in
// FunctionCallbackInfo<Value>&)
//
args.GetReturnValue().Set(str);
}
//
// * Initialize the object and expose the functions that need exposure.
//
void Init(Local<Object> exports)
{
NODE_SET_METHOD(exports, "GenerateKey", GenerateKey);
NODE_SET_METHOD(exports, "Encrypt", Encrypt);
}
//
// There is no semi-colon after NODE_MODULE as it's not a function
//
NODE_MODULE(, Init)
}
其他答案提到了背景,但没有提到具体问题,或者他们对generated_key
疑问,但是可以。 [edit]我现在看到molbdnilo的评论; 我以为有人提到过它,但是我似乎找不到它! [/ edit]这是真正的原因:
对象cPayload
是char[4]
。 像任何数组一样,它的名称在多个上下文中(包括将其作为函数参数传递时)隐式转换(通常用俗语称为“ decays”)转换为char *
。 您将其传递给函数encrypt()
,该函数在该位置需要一个unsigned char *
。 但是char *
和unsigned char *
是两种不同的类型。 因此,错误。
回到背景,因为它值得指定。 char
必须具有与signed char
或unsigned char
相同的值范围,可以是具有可能的截断的static_cast
,并且具有相同的别名能力-等等-但是,在任何情况下,它都被视为不同的类型。 您可以通过检查3种类型的typeid
结果来验证这一点而没有任何编译错误。
我想我不明白代码的哪一部分出了问题。 但是我认为您正在尝试将char *转换为两种不同类型的const unsigned char *。 尝试将char *类型更改为unsigned char *,或者在代码中的任何地方都使用unsigned char *。 这是一个答案,可能会帮助您说普通字符,有符号字符和无符号字符是三种不同的类型。
默认的char
类型具有实现定义的签名,可以是有符号的也可以是无符号的,具体取决于编译器。 因此,除了字符串之外,它永远不能用于存储任何内容。 切勿使用它存储其他形式的数据。 它不一定与unsigned char
兼容。
之所以不进行编译,可能是因为char
与C和C ++编译器之间char
变化所引起的签名,但也可能是因为C ++具有比C更严格和更好的类型安全系统。即使在C和C ++中将这两种类型混合在一起始终是一种不好的做法,但代码却不会产生诊断消息就可以通过。
只需更改为unsigned char
或最好更改为uint8_t
,问题就会消失。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.