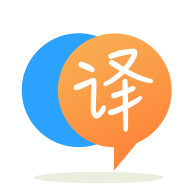
[英]Get Nested json array of data Laravel Eloquent model with Relationship
[英]Laravel Eloquent - Get Eloquent Relationship Model Data From nested Relationship
我有3个这样的模型
用户
<?php
namespace App;
use Illuminate\Foundation\Auth\User as Authenticatable;
class User extends Authenticatable
{
protected $fillable = [
'profile_picture',
'first_name',
'last_name',
'email',
'cell_no',
'website',
'date_of_birth',
'social_security_number_p1',
'social_security_number_p2',
'social_security_number_p3',
'address',
'location_latitude',
'location_longitude',
'password',
'user_type',
'is_enabled'
];
protected $hidden = [
'password',
'remember_token',
'id',
'user_type',
'is_enabled',
'created_at',
'updated_at'
];
public function advertisement()
{
return $this->hasMany('App\Advertisement', 'user_id');
}
}
广告
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use Auth;
class Advertisement extends Model
{
protected $fillable = [
'user_id',
'category_id',
'sub_category_id',
'title',
'price',
'description',
'address',
'location_lat',
'location_lon',
'is_active',
'deleted_at'
];
public function User()
{
return $this->belongsTo('App\User','user_id', 'id');
}
public function Offer()
{
return $this->hasMany('App\Offer', 'add_id', 'id');
}
}
提供
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Offer extends Model
{
protected $fillable = [
'add_id',
'user_id',
'price',
'message'
];
public function User()
{
return $this->hasOne('App\User','user_id','id');
}
public function Advertisement()
{
return $this->hasOne('App\advertisement','add_id','id');
}
}
我想获得带有报价的广告以及带有报价发送者(用户)的报价。
所以,我正在做这样的事情-
return Advertisement::with('AdvertisementImages')
->with('Offer')
->with('User')
->where('user_id', Auth::user()->id)
->get();
并得到这个-
[
{
"id": 1,
"user_id": 16,
"title": "123asd",
"price": 123,
"description": "asd asd asd ",
"address": "Dhaka University Campus, Dhaka, Dhaka Division, Bangladesh",
"location_lat": 23.73,
"location_lon": 90.39,
"total_views": "110",
"avg_rating": "4.0000",
"is_reviewed": 0,
"is_offer_sent": 1,
"advertisement_images": [
{
"image_name": "16-68261.jpg"
},
{
"image_name": "16-34746.JPG"
},
{
"image_name": "16-79570.jpg"
}
],
"offer": [
{
"id": 1,
"add_id": 1,
"user_id": 9,
"price": 123,
"message": "asd ad asdasd ",
"created_at": "2016-08-11 02:22:43",
"updated_at": "2016-08-11 02:22:43"
},
{
"id": 2,
"add_id": 1,
"user_id": 15,
"price": 43,
"message": "as dfasd ",
"created_at": "2016-08-11 02:24:04",
"updated_at": "2016-08-11 02:24:04"
},
{
"id": 3,
"add_id": 1,
"user_id": 16,
"price": 23,
"message": "hgf",
"created_at": "2016-08-11 02:25:31",
"updated_at": "2016-08-12 01:37:42"
}
],
"user": {
"profile_picture": "",
"first_name": "Ne",
"last_name": "Ajgor",
"cell_no": null,
"email": "ajgor@me.com",
"website": "",
"date_of_birth": "0000-00-00",
"social_security_number_p1": "",
"social_security_number_p2": "",
"social_security_number_p3": "",
"address": "",
"location_latitude": 0,
"location_longitude": 0
}
}
]
所以,这是错误的。 我正在从Advertisement获取用户。
但是我喜欢从Offer获得用户。
所以,我需要这样的东西-
[
{
"id": 1,
"user_id": 16,
"title": "123asd",
"price": 123,
"description": "asd asd asd ",
"address": "Dhaka University Campus, Dhaka, Dhaka Division, Bangladesh",
"location_lat": 23.73,
"location_lon": 90.39,
"total_views": "110",
"avg_rating": "4.0000",
"is_reviewed": 0,
"is_offer_sent": 1,
"advertisement_images": [
{
"image_name": "16-68261.jpg"
},
{
"image_name": "16-34746.JPG"
},
{
"image_name": "16-79570.jpg"
}
],
"offer": [
{
"id": 1,
"add_id": 1,
"user_id": 9,
"price": 123,
"message": "asd ad asdasd ",
"created_at": "2016-08-11 02:22:43",
"updated_at": "2016-08-11 02:22:43",
"user": {
"profile_picture": "",
"first_name": "Me",
"last_name": "Ajgor",
"cell_no": null,
"email": "ajgor@me.com",
"website": "",
"date_of_birth": "0000-00-00",
"social_security_number_p1": "",
"social_security_number_p2": "",
"social_security_number_p3": "",
"address": "",
"location_latitude": 0,
"location_longitude": 0
}
},
{
"id": 2,
"add_id": 1,
"user_id": 15,
"price": 43,
"message": "as dfasd ",
"created_at": "2016-08-11 02:24:04",
"updated_at": "2016-08-11 02:24:04",
"user": {
"profile_picture": "",
"first_name": "Ne",
"last_name": "Ajgor",
"cell_no": null,
"email": "ajgor@me.com",
"website": "",
"date_of_birth": "0000-00-00",
"social_security_number_p1": "",
"social_security_number_p2": "",
"social_security_number_p3": "",
"address": "",
"location_latitude": 0,
"location_longitude": 0
}
},
{
"id": 3,
"add_id": 1,
"user_id": 16,
"price": 23,
"message": "hgf",
"created_at": "2016-08-11 02:25:31",
"updated_at": "2016-08-12 01:37:42",
"user": {
"profile_picture": "",
"first_name": "Je",
"last_name": "Ajgor",
"cell_no": null,
"email": "ajgor@me.com",
"website": "",
"date_of_birth": "0000-00-00",
"social_security_number_p1": "",
"social_security_number_p2": "",
"social_security_number_p3": "",
"address": "",
"location_latitude": 0,
"location_longitude": 0
}
}
]
}
]
有人可以帮我吗,我需要打电话给我吗?
在此先感谢您的帮助。
我已经解决了
Advertisement::with('AdvertisementImages')
->with('Offer','Offer.User')
->where('user_id', Auth::user()->id)
->has('Offer')
->get();
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.