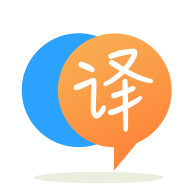
[英]Jquery copying the attribute of the the thead tr td to the tbody tr td
[英]move first Tr to thead and replace td to th using jquery
我有一个像这样的 ajax 调用返回的字符串
<table>
<tbody>
<tr>
<td>Name</td>
<td>Age</td>
</tr>
<tr>
<td>Ron</td>
<td>28</td>
</tr>
</tbody>
</table>
现在我想将其重组为
<table>
<thead>
<tr>
<th>Name</th>
<th>Age</th>
</tr></thead>
<tbody>
<tr>
<td>Ron</td>
<td>28</td>
</tr>
</tbody>
</table>
我怎样才能在 jQuery 中做到这一点?
下面的行插入一个 thead 并将第一个 tr 复制到其中
$(data.d).prepend("<thead></thead>").find("thead").html($(data.d).find("tr:eq(0)"))
我正在尝试使用此行删除上一行之后 tbody 中的第一行
$(data.d).find("tbody tr:eq(0)").remove()
如何附加thead
,将tbody
第一个tr
移动到它,将其中的所有td
替换为th
,最后从tbody
删除第一个tr
我的建议是:
$(function () { var th = $('table tbody tr:first td').map(function(i, e) { return $('<th>').append(e.textContent).get(0); }); $('table tbody').prepend($('<thead>').append(th)).find('tr:first').remove(); console.log($('table').html()); });
<script src="https://code.jquery.com/jquery-1.12.4.min.js"></script> <table> <tbody> <tr> <td>Name</td> <td>Age</td> </tr> <tr> <td>Ron</td> <td>28</td> </tr> </tbody> </table>
一个更简单的解决方案:
t = $('table#tbl2') firstTr = t.find('tr:first').remove() firstTr.find('td').contents().unwrap().wrap('<th>') t.prepend($('<thead></thead>').append(firstTr))
table thead tr th { background: green; } table tbody tr td { background: red; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <table id="tbl1"><tbody><tr><td>Name</td><td>Age</td></tr><tr><td>Ron</td><td>28</td></tr></tbody></table> <br /><br /> <table id="tbl2"><tbody><tr><td>Name</td><td>Age</td></tr><tr><td>Ron</td><td>28</td></tr></tbody></table>
下面是代码的解释:
dom
删除第一个tr
元素。td
元素,获取它们的contents()
并解开td
。th
标签包裹td
每个contents()
。<thead>
标记添加到 table 并将我们处理的tr
附加到thead
一些分步解决方案,
var jsonString = "<table><tbody><tr><td>Name</td><td>Age</td></tr><tr><td>Ron</td><td>28</td></tr></tbody></table>"; var t =$(jsonString); //jquery Table Object var firstTR = $('tr:first',t); //geting firstTR $('<thead></thead>') .prependTo(t) .append( $('td',firstTR).map( function(i,e){ return $("<th>").html(e.textContent).get(0); } ) ); firstTR.remove();//removing First TR $("#div").html(t); //Output console.log(t.html());
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <div id="div"> </div>
你可以:
$('table').prepend('<thead></thead>'); // Add thead
$('table tr:eq(0)').prependTo('table thead'); // move first tr to thead
$('table thead').html(function(id, html) { // you might use callback to set html in jquery
return html.replace(/td>/g, 'th>'); // replace td by th
// use regExp to replace all
});
当您使用 .prependTo 时,这会将元素移动到顶部的另一个元素,您可能会在 jquery 的 .html 函数中使用回调。
参考:
几年过去了(所以不再有 jQuery),我想这样做并像这样解决了它。 这个想法是 1)获取第一行( <tr>
),2)将第一行上的每个<td>
标签替换为<th>
。
const txt = `<table>
<tbody>
<tr>
<td>Name</td>
<td>Age</td>
</tr>
<tr>
<td>Ron</td>
<td>28</td>
</tr>
</tbody>
</table>`;
const el = document.createElement('table');
el.innerHTML = txt;
[...el.querySelector('tr').querySelectorAll('td')].forEach(td =>
td.outerHTML = td.outerHTML
.replace(/<td([^>]*)>/, '<th$1>')
.replace(/<\/td>/, '</th>')
);
console.log(el.outerHTML);
/*
<table>
<tbody>
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td>Ron</td>
<td>28</td>
</tr>
</tbody>
</table>
*/
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.