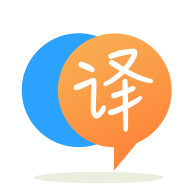
[英]Is this default behaviour for instances whose constructor's prototype property is not set to an object?
[英]How can I set a default property for an object that's passed into a constructor?
我试图在一处进行更改以影响传递给对象的所有实例化的配置对象。 该对象在全局范围内可用,如下所示:
function Crayons(){
return {
foo: ThirdPartyFoo
}
}
在我的项目中使用var myFoo = new Crayons().foo({color: "red"});
初始化该对象var myFoo = new Crayons().foo({color: "red"});
我想将{color: "blue"}
为默认值,这样,如果某人没有通过颜色,则设置为蓝色。
我试着做
function Crayons(){
var fooWithDefaults = function(){
this = new ThirdPartyFoo(arguments); //this is invalid
this.color = "blue"; //and this would overwrite color if it was set
}
return {
foo: fooWithDefaults
}
}
但是new
关键字让我失望,因为我不知道如何创建本质上说this = new 3rdPartyFoo
的javascript构造函数。
我想念什么?
您可以装饰构造函数:
function Crayons(){
function fooWithDefaults() {
3rdPartyFoo.apply(this, arguments); // this is what you're looking for
if (!this.color) // or whatever to detect "not set"
this.color = "blue";
}
fooWithDefaults.prototype = 3rdPartyFoo.prototype; // to make `new` work
return {
foo: fooWithDefaults
}
}
或者只是将其设为返回实例的工厂:
function Crayons(){
function fooWithDefaults(arg) {
var that = new 3rdPartyFoo(arg); // you should know how many arguments it takes
if (!that.color) // or whatever to detect "not set"
that.color = "blue";
return that;
}
return {
foo: fooWithDefaults
}
}
在这里,您还可以在调用var myFoo = Crayons.foo({color: "red"});
时删除new
var myFoo = Crayons.foo({color: "red"});
创建实例后修改实例的另一种方法是装饰传入的选项,这通常是更好的解决方案:
function Crayons(){
function fooWithDefaults(arg) {
if (!arg.color) // or whatever to detect "not set"
arg.color = "blue";
return new 3rdPartyFoo(arg);
}
return {
foo: fooWithDefaults
}
}
function ThirdPartyCrayon(config) { // constructor :: possible original Crayon implementation Object.assign(this, config); this.type = "thirdpartycrayon"; } function createCrayonSetting(defaultConfig) { // factory (creates a closure) function CrayonWithDefaults() { // constructor :: customized Crayon wrapper Object.assign(this, defaultConfig); ThirdPartyCrayon.apply(this, arguments); } return { Crayon: CrayonWithDefaults } } var setting = createCrayonSetting({color: "blue", strokeWidth: "thin"}), crayon1 = new setting.Crayon({color: "red", strokeWidth: "bold"}), crayon2 = new setting.Crayon({color: "green"}); crayon3 = new setting.Crayon(); console.log("crayon1 : ", crayon1); console.log("crayon2 : ", crayon2); console.log("crayon3 : ", crayon3);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.