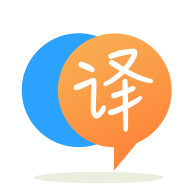
[英]Can I filter out duplicates within an array list using hashset in Java without distorting the order of the array?
[英]Java - Is there anyway I can prevent duplicates in an array without using an ArrayList?
我正在尝试创建代码以防止重复的数组元素。 无论如何,我无需创建arrayList就可以做到这一点?
当运行程序时,当我输入第一个#时会发生此错误:
线程“主”中的异常java.lang.ArrayIndexOutOfBoundsException:在DuplicateElimination.main(DuplicateElimination.java:32)处为-1
这是我的代码:
int[] numList = new int[5];
int newValue;
boolean invalid;
for (int i = 0; i < numList.length; i++){
do{
System.out.print("Please enter number");
System.out.println(" ");
newValue = input.nextInt();
//This is where the error occurs when I try to compare
//The last list element to the input value
invalid = numList[i-1] == newValue;
if(newValue < 10 || newValue > 100){
System.out.print("Invalid number, Please enter a number between 10 and 100");
newValue = input.nextInt();
}
if(invalid){
System.out.print("That number was entered already try again");
}
}while(invalid);
insertIntoArray(numList, i, newValue);
printArray(numList);
}
您可以通过以下方式防止集合中的重复项
另一种方法是:在添加新元素之前; 您只需简单地遍历完整的现有数组,以查看它是否已经包含要添加的内容。 如果是这样,您的代码将拒绝添加已知的“新”元素。
本质上:您绝对不需要“第二” ArrayList来执行此操作。 如果您的应用程序的全部目的是“收集”某些对象而不重复,那么您只需要使用Set。 您只需踢出阵列即可; 而您只使用一个Set。
使用“ Arrays”类binarySearch方法还有另一种方法。
binarySearch方法接受要在数组上搜索的数组和键,如果找到键,则返回索引。
数组输入应按排序顺序。 您可以使用Arrays.sort对数组进行排序并将其用作输入。
例:
int index = Arrays.binarySearch(Arrays.sort(inputArray),key);
如果找到键,请不要将值添加到数组。 否则,将值添加到数组。
您的代码只有在以下位置启动矩阵时才是好的:
invalid = numList[i-1] == newValue;
尝试这个 :
invalid = numList[i] == newValue;
如果要防止重复元素,则HashSet是一个不错的选择。
在数组中,您将需要一些东西来跟踪元素以进行重复。 HashSet
将以O(1)
时间复杂度为您完成此任务。
使用HashSet.contains(Object)
,可以检查元素是否已经存在。 使用HashSet.toArray(T[])
,您可以最后获取数组。
关于java.lang.ArrayIndexOutOfBounds
,您的迭代变量从0开始,所以:
if i = 0; numList[i-1] = numList[-1]
这是无效索引,因为数组索引从0开始。因此,更改为numList[i]
或将循环更改为for(int i = 1; i <= numList.length; i++)
。
此答案将单词用作您的问题标题,并防止重复使用而不使用ArrayList
或其他集合类。 我将您的内部do
循环更改为:
do {
System.out.print("Please enter number");
System.out.println(" ");
newValue = input.nextInt();
// See if value was already entered; search all array elements left of index i
int ix = 0;
while (ix < i && numList[ix] != newValue) {
ix++;
}
// now either ix == i or numList[ix] == newValue;
// if ix is not i, it means we encountered a duplicate left of index i
invalid = ix < i;
if (invalid) {
System.out.println("That number was entered already, try again");
} else if (newValue < 10 || newValue > 100) {
System.out.println("Invalid number, please enter a number between 10 and 100");
invalid = true;
}
} while (invalid);
这可以确保最终插入numList[i]
满足两个条件:它的范围是10到100,并且不是重复的值。 在所有先前输入的值中搜索重复项(也就是说,由于没有先前输入的值,因此首次在所有值中都没有)。
这不是我建议用于生产的代码(而不是使用Set
),但是对于练习来说很好。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.