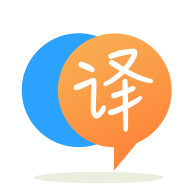
[英]How to add auto layout constraints to UINavigationBar programmatically
[英]How To Set Auto Layout Constraints Programmatically for UITextView For Universal App with swift
func setupView()
{
self.blueView = UIView()
self.blueView?.backgroundColor = UIColor.blueColor()
self.blueView?.translatesAutoresizingMaskIntoConstraints = false
self.addSubview(self.blueView!)
let blueViewCenterXConstraint = NSLayoutConstraint(item: self.blueView!, attribute: NSLayoutAttribute.CenterX, relatedBy: NSLayoutRelation.Equal, toItem: self, attribute: NSLayoutAttribute.CenterX, multiplier: 0.6, constant: 0)
let blueViewCenterYConstraint = NSLayoutConstraint(item: self.blueView!, attribute: NSLayoutAttribute.CenterY, relatedBy: NSLayoutRelation.Equal, toItem: self, attribute: NSLayoutAttribute.CenterY, multiplier: 1.0, constant: 0)
let blueViewWidthConstraint = NSLayoutConstraint(item: self.blueView!, attribute: NSLayoutAttribute.Width, relatedBy: NSLayoutRelation.Equal, toItem: nil, attribute: NSLayoutAttribute.NotAnAttribute, multiplier: 0.6, constant: 150)
let blueViewHeightConstraint = NSLayoutConstraint(item: self.blueView!, attribute: NSLayoutAttribute.Height, relatedBy: NSLayoutRelation.Equal, toItem: nil, attribute: NSLayoutAttribute.NotAnAttribute, multiplier: 1.0, constant: 150)
self.addConstraints([blueViewCenterXConstraint,blueViewCenterYConstraint,blueViewWidthConstraint,blueViewHeightConstraint])
self.redView = UIView()
self.redView?.backgroundColor = UIColor.redColor()
self.redView?.translatesAutoresizingMaskIntoConstraints = false
self.addSubview(self.redView!)
let redViewCenterXConstraint = NSLayoutConstraint(item: self.redView!, attribute: NSLayoutAttribute.Leading, relatedBy: NSLayoutRelation.Equal, toItem: blueView, attribute: NSLayoutAttribute.TrailingMargin , multiplier: 0.5, constant: 0)
let redViewCenterYConstraint = NSLayoutConstraint(item: self.redView!, attribute: NSLayoutAttribute.Top, relatedBy: NSLayoutRelation.Equal, toItem: blueView, attribute: NSLayoutAttribute.Top, multiplier: 0.5, constant: 0)
let redViewWidthConstraint = NSLayoutConstraint(item: self.redView!, attribute: NSLayoutAttribute.Width, relatedBy: NSLayoutRelation.Equal, toItem: blueView, attribute: NSLayoutAttribute.Width, multiplier: 0.5, constant: 150)
let redViewHeightConstraint = NSLayoutConstraint(item: self.redView!, attribute: NSLayoutAttribute.Height, relatedBy: NSLayoutRelation.Equal, toItem: blueView, attribute: NSLayoutAttribute.Height, multiplier: 0.5, constant: 150)
self.addConstraints([redViewCenterXConstraint,redViewCenterYConstraint,redViewWidthConstraint,redViewHeightConstraint])
}
我已将上面的代码应用于为通用应用程序创建和设置UITextView的自动布局约束。 我想为每个设备将这两个UITextView彼此并排放置,它们之间的空间为水平,垂直居中为10。 任何人都将不胜感激修复所提到的问题,这对我很有帮助。
我已经自由地看了您先前的问题 ,而且我使用了NSLayoutAnchors
( 在此介绍),因为我认为它们更易于阅读。
基于以上内容,此UIView
子类:
import UIKit
class UIViewUsingTextField: UIView {
let width: CGFloat = 150.0
var blueview = UIView()
var redview = UIView()
init() {
super.init(frame: CGRect.zero)
self.backgroundColor = UIColor.whiteColor()
self.setupView()
}
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)!
setupView()
}
override init(frame: CGRect) {
super.init(frame: frame)
setupView()
}
func setupView() {
//add blue view
blueview.backgroundColor = UIColor.blueColor()
blueview.translatesAutoresizingMaskIntoConstraints = false
addSubview(blueview)
//center the blue view and move it 1/2 view width to the left
blueview.centerXAnchor.constraintEqualToAnchor(self.centerXAnchor, constant: -(width/2)).active = true
blueview.centerYAnchor.constraintEqualToAnchor(self.centerYAnchor).active = true
blueview.widthAnchor.constraintEqualToConstant(width).active = true
blueview.heightAnchor.constraintEqualToConstant(width).active = true
//add red view
redview.backgroundColor = UIColor.redColor()
redview.translatesAutoresizingMaskIntoConstraints = false
addSubview(redview)
//place red view 10 px to the right of blue view
redview.leadingAnchor.constraintEqualToAnchor(blueview.trailingAnchor, constant: 10.0).active = true
redview.centerYAnchor.constraintEqualToAnchor(blueview.centerYAnchor).active = true
redview.widthAnchor.constraintEqualToAnchor(blueview.widthAnchor).active = true
redview.heightAnchor.constraintEqualToAnchor(blueview.heightAnchor).active = true
}
}
给我这个布局
希望这类似于您的追求。
我已经包含了使用您的代码的解决方案,但同时也想向您展示如何使用constraintWithVisualFormat完成此操作,因为我认为它更容易阅读。
针对您的代码的解决方案: 请注意,此处的宽度设置为固定值。 不知道这是否真的是您想要的。
func setupView()
{
self.blueView = UIView()
self.blueView?.backgroundColor = UIColor.blueColor()
self.blueView?.translatesAutoresizingMaskIntoConstraints = false
self.view.addSubview(self.blueView!)
let blueViewCenterXConstraint = NSLayoutConstraint(item: self.blueView!, attribute: NSLayoutAttribute.Trailing, relatedBy: NSLayoutRelation.Equal, toItem: self.view, attribute: NSLayoutAttribute.CenterX, multiplier: 1, constant: -5)
let blueViewCenterYConstraint = NSLayoutConstraint(item: self.blueView!, attribute: NSLayoutAttribute.CenterY, relatedBy: NSLayoutRelation.Equal, toItem: self.view, attribute: NSLayoutAttribute.CenterY, multiplier: 1.0, constant: 0)
let blueViewWidthConstraint = NSLayoutConstraint(item: self.blueView!, attribute: NSLayoutAttribute.Width, relatedBy: NSLayoutRelation.Equal, toItem: nil, attribute: NSLayoutAttribute.NotAnAttribute, multiplier: 1, constant: 150)
let blueViewHeightConstraint = NSLayoutConstraint(item: self.blueView!, attribute: NSLayoutAttribute.Height, relatedBy: NSLayoutRelation.Equal, toItem: nil, attribute: NSLayoutAttribute.NotAnAttribute, multiplier: 1.0, constant: 150)
self.view.addConstraints([blueViewCenterXConstraint,blueViewCenterYConstraint,blueViewWidthConstraint,blueViewHeightConstraint])
self.redView = UIView()
self.redView?.backgroundColor = UIColor.redColor()
self.redView?.translatesAutoresizingMaskIntoConstraints = false
self.view.addSubview(self.redView!)
let redViewCenterXConstraint = NSLayoutConstraint(item: self.redView!, attribute: NSLayoutAttribute.Leading, relatedBy: NSLayoutRelation.Equal, toItem: blueView, attribute: NSLayoutAttribute.Trailing , multiplier: 1, constant: 10)
let redViewCenterYConstraint = NSLayoutConstraint(item: self.redView!, attribute: NSLayoutAttribute.Top, relatedBy: NSLayoutRelation.Equal, toItem: blueView, attribute: NSLayoutAttribute.Top, multiplier: 1, constant: 0)
let redViewWidthConstraint = NSLayoutConstraint(item: self.redView!, attribute: NSLayoutAttribute.Width, relatedBy: NSLayoutRelation.Equal, toItem: blueView, attribute: NSLayoutAttribute.Width, multiplier: 1, constant: 1)
let redViewHeightConstraint = NSLayoutConstraint(item: self.redView!, attribute: NSLayoutAttribute.Height, relatedBy: NSLayoutRelation.Equal, toItem: blueView, attribute: NSLayoutAttribute.Height, multiplier: 1, constant: 1)
self.view.addConstraints([redViewCenterXConstraint,redViewCenterYConstraint,redViewWidthConstraint,redViewHeightConstraint])
}
使用视觉约束的解决方案
let views = ["redView": redView!, "blueView": blueView!]
//setup the horizontal constraints to have 15 leading and trailing constraints, equal widths for blueView and redView and 10 spacing in between.
self.view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("H:|-15-[blueView(==redView)]-10-[redView]-15-|", options: .AlignAllCenterY, metrics: nil, views: views))
//set the height of the two views
self.view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:[blueView(150)]", options: .AlignAllCenterX, metrics: nil, views: views))
self.view.addConstraints(NSLayoutConstraint.constraintsWithVisualFormat("V:[redView(150)]", options: .AlignAllCenterX, metrics: nil, views: views))
//align the two views relative to the centre of the superview.
blueView!.centerYAnchor.constraintEqualToAnchor(view!.centerYAnchor, constant: 0).active = true
redView!.centerYAnchor.constraintEqualToAnchor(blueView!.centerYAnchor).active = true
为您的示例将
self.view
替换为self
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.