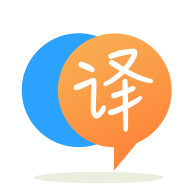
[英]how to send multiple json objects /data to server using volley in android
[英]Send data to server as json format using android Volley
我想以JSON格式将数据从android应用发送到远程服务器。 以下是我的json格式:-
{
"contacts": [
{
"name": "ritva",
"phone_no": "12345657890",
"user_id": "1"
},
{
"name": "jisa",
"phone_no": "12345657890",
"user_id": "1"
},
{
"name": "tithi",
"phone_no": "12345657890",
"user_id": "1"
}
]
}
谁能告诉我如何使用Volley发送此数据?
POST/GET
, url
, response & error
侦听器之类的方法。 对于发送json,请重写getBody()
方法,并在其中传递要发送的json。 RequestQueue
并将请求添加到其中。 您可以通过调用start()
来启动它 尝试这个 :
// Instantiate the RequestQueue.
RequestQueue queue = Volley.newRequestQueue(this);
String url ="http://www.google.com";
// Request a string response from the provided URL.
StringRequest stringRequest = new StringRequest(Request.Method.POST, url,
new Response.Listener<String>() {
@Override
public void onResponse(String response) {
// your response
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
// error
}
}){
@Override
public byte[] getBody() throws AuthFailureError {
String your_string_json = ; // put your json
return your_string_json.getBytes();
}
};
// Add the request to the RequestQueue.
queue.add(stringRequest);
requestQueue.start();
欲了解更多信息请参阅 本
为了发送JSON
类型的数据,您应该使用齐射发出JSON
请求
// Instantiate the RequestQueue.
RequestQueue queue = Volley.newRequestQueue(this);
String url ="http://www.google.com";
JsonObjectRequest jsObjRequest = new JsonObjectRequest
(Request.Method.POST, url, obj, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
// TODO Auto-generated method stub
}
});
// Add the request to the RequestQueue.
queue.add(stringRequest);
requestQueue.start();
其中object
是您要发送的JSONObject
。 询问是否要进一步澄清。
如果可以,请对此进行标记。
1.将Volley和Gson Dependency添加到build.gradle:
'com.mcxiaoke.volley:library:1.0.19'
'com.google.code.gson:gson:2.7'
注意:如果String变量中包含JSON数据,则只需将String变量作为第三个参数传递给JsonObjectRequest。 (转到步骤6)
如果您的类中包含JSON数据,则只需在JsonObjectRequest的第三个参数的gson.toJson()中传递该类即可。 (转到步骤6)
如果要在类中获取数据,则需要创建与JSON数据相同的类结构。 (转到步骤2)
2.然后使用http://www.jsonschema2pojo.org/为上述JSON结构创建POJO类。
图片中的示例: 红色标记显示需要在现场进行的更改
然后您将获得两个类,分别为ContactsTop和Contact。 注意:ContactsTop是从jsonschema2pojo.com创建POJO类时提供的名称。
3.将上面生成的类添加到您的项目中
4.创建Volley RequestQueue对象和gson对象。
RequestQueue requestQueue = Volley.newRequestQueue(this);
Gson gson = new Gson();
5.然后将上述JSON数据添加到POJO类。
ContactsTop contactsTop=new ContactsTop();
List<Contact> contactList =new ArrayList();
Contact contact=new Contact();
contact.setPhoneNo("12345657890");
contact.setName("ritva");
contact.setUserId("1");
contactList.add(contact);
contactsTop.setContacts(contactList);
6.创建JSONObject以使用您的数据调用Web服务。
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest(Request.Method.POST, "www.your-web-service-url.com/sendContact.php", gson.toJson(contactsTop), new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
Log.v("Volley:Response ", ""+response.toString());
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Log.v("Volley:ERROR ", error.getMessage().toString());
}
});
7.将您的jsonObjectRequest添加到requestQueue中。 (不要忘记添加此行。这将在RequestQueue中添加您的请求,然后只有您将从服务中获得JSON响应或错误)。 不要忘记在AndroidManifest.xml中添加INTERNET权限
requestQueue.add(jsonObjectRequest);
然后,您将从android Log Monitor中的“远程服务”获得响应或错误。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.