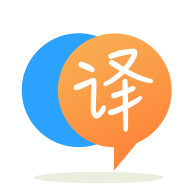
[英]How to compare two arrays containing dates to check if any values match?
[英]How to check if two arrays of dates have matching items?
我有两个日期数组。 第一个具有所有预订的日期,第二个具有用户将选择的“开始日期”和“结束日期”之间的日期。
现在,我必须确认从预定满的日期数组中找不到开始和停止之间的日期。
我正在使用Vue.js更新数据。
这是我为获取这些日期所做的事情:
/**
* Get dates from datepicker and format it to the same format that fully booked array has it's dates.
**/
var day1 = moment( this.startDate, 'DD/MM/Y').format('Y,M,D');
var day2 = moment( this.endDate, 'DD/MM/Y').format('Y,M,D');
var start = new Date(day1);
var end = new Date(day2);
/**
* Get dates between start and stop and return them in the dateArray.
**/
Date.prototype.addDays = function(days) {
var dat = new Date(this.valueOf());
dat.setDate(dat.getDate() + days);
return dat;
};
function getDates(startDate, stopDate) {
var dateArray = [];
var currentDate = startDate;
while (currentDate <= stopDate) {
dateArray.push(currentDate);
currentDate = currentDate.addDays(1);
}
return dateArray;
}
var dateArray = getDates(start, end);
/**
* Set dateArray in to Vue.js data.
**/
this.$set('daysBetweenStartStop', dateArray);
/**
* Get arrays of dates from the Vue.js data. calendar = Booked dates | matchingDays = Dates between start and stop.
**/
var calendar = this.fullDates;
var matchingDays = this.daysBetweenStartStop;
/**
* @description determine if an array contains one or more items from another array.
* @param {array} haystack the array to search.
* @param {array} arr the array providing items to check for in the haystack.
* @return {boolean} true|false if haystack contains at least one item from arr.
*/
var findIfMatch = function (haystack, arr) {
return arr.some(function (v) {
return haystack.indexOf(v) >= 0;
});
};
var matching = findIfMatch(calendar, matchingDays);
/**
* Check the results. If false we are good to go.
**/
if (matching){
alert('WE FOUND A MATCH');
} else {
alert('GOOD TO GO');
}
数组采用以下格式:
var calendar = [
Sun Oct 02 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 09 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 16 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 23 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 30 2016 00:00:00 GMT+0300 (EEST)
]
var matchingDays = [
Fri Oct 28 2016 00:00:00 GMT+0300 (EEST),
Sat Oct 29 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 30 2016 00:00:00 GMT+0300 (EEST)
]
我的问题是 ,即使这两个数组具有完全相同的日期,它们仍将以某种方式被视为不相同。 任何想法如何使它工作?
要从两个数组中获取匹配的记录,请使用此
var calendar = [
Sun Oct 02 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 09 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 16 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 23 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 30 2016 00:00:00 GMT+0300 (EEST)
];
var matchingDays = [
Fri Oct 28 2016 00:00:00 GMT+0300 (EEST),
Sat Oct 29 2016 00:00:00 GMT+0300 (EEST),
Sun Oct 30 2016 00:00:00 GMT+0300 (EEST)
];
var newCalendar = [];
var newMatchingDays = []
$.map(calendar, function(date){
newCalendar.push(date.toString())
});
$.map(matchingDays, function(date){
newMatchingDays.push(date.toString())
});
var result = [];
$.map(newCalendar, function (val, i) {
if ($.inArray(val, newMatchingDays) > -1) {
result.push(val);
}
});
console.log(result);
您的match函数应如下所示:
findIfMatch = function (haystack, arr){
var i = 0;//array index
var j = 0;//array index
while(j < arr.length && i < haystack.length){
cur_cal = Date.parse(haystack[i]);
cur_match = Date.parse(arr[j]);
if(cur_cal > cur_match){
j++;
}else if(cur_cal < cur_match){
i++;
}else{
return true;
}
}
return false;
}
首先,您不能像这样比较日期,日期是一个对象。 例如。
var d1 = new Date('2016-09-30');
var d1 = new Date('2016-09-30');
console.log(d1 === d2); // = false
您将需要循环数组并比较每个项目,而不是使用indexOf。 或者可以使用Array.filter()。 或者,也可以使用和对象作为查询。
例如。 如果说你有->
var calendar = {
"Sun Oct 02 2016 00:00:00 GMT+0300 (EEST)": true,
"Sun Oct 09 2016 00:00:00 GMT+0300 (EEST)": true,
"Sun Oct 16 2016 00:00:00 GMT+0300 (EEST)": true,
"Sun Oct 23 2016 00:00:00 GMT+0300 (EEST)": true,
"Sun Oct 30 2016 00:00:00 GMT+0300 (EEST)": true
};
if (calendar["Sun Oct 16 2016 00:00:00 GMT+0300 (EEST)"]) {
console.log("We have date");
}
请注意,我如何使用字符串表示形式表示日期,例如,它也可能是数字。 从Date.getTime()。
这是使用Array.filter的方法,我认为这不是对象查找的速度。 但是,我们开始。
var calendar = [
new Date('2016-09-01'),
new Date('2016-09-12'),
new Date('2016-09-10')
];
var finddate = new Date('2016-09-12');
var found = calendar.filter(function (a) { return a.getTime() === finddate.getTime();});
而且,如果您不介意使用第三方库,请尝试使用下划线。
例如。
var days = [new Date('2016-09-01'), new Date('2016-09-10'), new Date('2016-09-30')];
var finddays = [new Date('2016-09-01'), new Date('2016-09-30')];
var found = _.intersectionWith(days, finddays,
function (a,b) { return a.getTime() === b.getTime(); });
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.