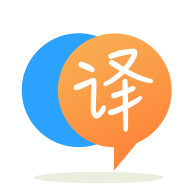
[英]Using an Array to print out a number of asterisks, dependent on number inputted from user in C
[英]C language- How to print out the largest number inputted into an array?
我希望能够打印出用户输入的最大年龄以及最小年龄。
另外,我注意到我的程序不包括小数点后的数字。 例如,它只会说 25.00,而不是 25.25。
任何帮助是极大的赞赏!
#include "stdafx.h"
#include "stdio.h"
void main()
{
int age[11];
float total = 0;
int i = 1;
float average;
do
{
printf("# %d: ", i);
scanf_s("%d", &age[i]);
total = (total + age[i]);
i = i + 1;
} while (i <= 10);
average = (total / 10);
printf("Average = %.2f", average);
}
我认为这会有所帮助。 对于小数点,您必须将数组声明为float
。 FLT_MAX这些宏定义了float
最大值。 期运用FLT_MAX之前,您应该inclue
float.h中头文件。
#include <stdio.h>
#include <float.h>
int main(void)
{
float age[11];
float total = 0;
int i = 1;
float average;
float largestInput = 0.0;
float smallestInput = FLT_MAX;
do
{
printf("# %d: ", i);
scanf("%f", &age[i]);
total = total + age[i];
//here i am checking the largest input
if (age[i]> largestInput){
largestInput = age[i];
}
//here i am checking the smallest input
if (age[i] < smallestInput) {
smallestInput = age[i];
}
i = i + 1;
} while (i <= 10);
average = (total / 10);
printf("Average = %.2f\n", average);
printf("Largest Input Is = %.2f\n", largestInput);
printf("Smallest Input IS = %.2f", smallestInput);
return 0;
}
伪代码。 保留两个额外的变量。 长最大输入,小测试输入。
设置最大输入 = 小测试输入 = 年龄 [0];
for (i = 0; i < 10; i++) {
if age[i]> largestInput{
largestInput = age[i];
}
if age[i] < smallestInput {
smalltestInput = age[i];
}
}
随心所欲打印
您的代码在我的系统上运行良好。 我已将您的代码修改为非 Windows 特定代码,并向您展示了如何计算输入的最小和最大数字。 这个想法是不断地将当前条目与当前最小和最大数字进行比较,并根据需要更改最小和最大。 下面的代码还显示了如何从数组索引 0 开始。
#include <stdio.h>
int main()
{
int age[10];
float total = 0;
int i = 0;
float average;
int large = -9999;
int small = 9999;
do
{
printf("# %d: ", i+1);
scanf("%d", &age[i]);
total = (total + age[i]);
if( age[i] < small )
{
small = age[i];
}
if( age[i] > large )
{
large = age[i];
}
i++;
} while (i < 10);
average = (total / 10.00);
printf("Smallest = %d Largest = %d \n", small, large);
printf("Average = %.2f\n", average);
}
# 1: 30
# 2: 40
# 3: 50
# 4: 2
# 5: 3
# 6: 4
# 7: 6
# 8: -1
# 9: 4
# 10: 5
Smallest = -1 Largest = 50
Average = 14.30
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.