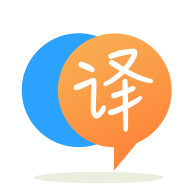
[英]Java Class isntance(with ToString method overriding) outputs “adt.BinaryNode@1e24e45”
[英]toString() method for a LinkedBinaryTree<E> class
有人可以帮我弄清楚如何为此类创建toString()吗? 它是一个包含Node类的LinkedBinaryTree类。 我认为提供这些类所需的超类和接口不会帮助创建toString方法,但是如果我错了,则可以纠正我。 这是课程:
public class LinkedBinaryTree<E> extends AbstractBinaryTree<E>{
protected static class Node<E> implements Position<E> {
private E element; // an element stored at this node
private Node<E> parent; // a reference to the parent node (if any)
private Node<E> left; // a reference to the left child (if any)
private Node<E> right; // a reference to the right child (if any)
public Node(E e, Node<E> above, Node<E> leftChild, Node<E> rightChild) {
element = e;
parent = above;
left = leftChild;
right = rightChild;
}
// accessor methods
public E getElement( ) { return element; }
public Node<E> getParent( ) { return parent; }
public Node<E> getLeft( ) { return left; }
public Node<E> getRight( ) { return right; }
// update methods
public void setElement(E e) { element = e; }
public void setParent(Node<E> parentNode) { parent = parentNode; }
public void setLeft(Node<E> leftChild) { left = leftChild; }
public void setRight(Node<E> rightChild) { right = rightChild; }
} //----------- end of nested Node class -----------
protected Node<E> createNode(E e, Node<E> parent,
Node<E> left, Node<E> right) {
return new Node<E>(e, parent, left, right);
}
// LinkedBinaryTree instance variables
protected Node<E> root = null; // root of the tree
private int size = 0; // number of nodes in the tree
// constructor
public LinkedBinaryTree( ) { } // constructs an empty binary tree
// nonpublic utility
protected Node<E> validate(Position<E> p) throws IllegalArgumentException {
if (!(p instanceof Node))
throw new IllegalArgumentException("Not valid position type");
Node<E> node = (Node<E>) p; // safe cast
if (node.getParent( ) == node) // our convention for defunct node
throw new IllegalArgumentException("p is no longer in the tree");
return node;
}
// accessor methods (not already implemented in AbstractBinaryTree)
public int size( ) {
return size;
}
public Position<E> root( ) {
return root;
}
public Position<E> parent(Position<E> p) throws IllegalArgumentException {
Node<E> node = validate(p);
return node.getParent( );
}
public Position<E> left(Position<E> p) throws IllegalArgumentException {
Node<E> node = validate(p);
return node.getLeft( );
}
public Position<E> right(Position<E> p) throws IllegalArgumentException {
Node<E> node = validate(p);
return node.getRight( );
}
public Position<E> addRoot(E e) throws IllegalStateException {
if (!isEmpty( )) throw new IllegalStateException("Tree is not empty");
root = createNode(e, null, null, null);
size = 1;
return root;
}
public Position<E> addLeft(Position<E> p, E e)
throws IllegalArgumentException {
Node<E> parent = validate(p);
if (parent.getLeft( ) != null)
throw new IllegalArgumentException("p already has a left child");
Node<E> child = createNode(e, parent, null, null);
parent.setLeft(child);
size++;
return child;
}
public Position<E> addRight(Position<E> p, E e)
throws IllegalArgumentException {
Node<E> parent = validate(p);
if (parent.getRight( ) != null)
throw new IllegalArgumentException("p already has a right child");
Node<E> child = createNode(e, parent, null, null);
parent.setRight(child);
size++;
return child;
}
public E set(Position<E> p, E e) throws IllegalArgumentException {
Node<E> node = validate(p);
E temp = node.getElement( );
node.setElement(e);
return temp;
}
public void attach(Position<E> p, LinkedBinaryTree<E> t1,
LinkedBinaryTree<E> t2) throws IllegalArgumentException {
Node<E> node = validate(p);
if (isInternal(p)) throw new IllegalArgumentException("p must be a leaf");
size += t1.size( ) + t2.size( );
if (!t1.isEmpty( )) { // attach t1 as left subtree of node
t1.root.setParent(node);
node.setLeft(t1.root);
t1.root = null;
t1.size = 0;
}
if (!t2.isEmpty( )) { // attach t2 as right subtree of node
t2.root.setParent(node);
node.setRight(t2.root);
t2.root = null;
t2.size = 0;
}
}
public E remove(Position<E> p) throws IllegalArgumentException {
Node<E> node = validate(p);
if (numChildren(p) == 2)
throw new IllegalArgumentException("p has two children");
Node<E> child = (node.getLeft( ) != null ? node.getLeft( ) : node.getRight( ) );
if (child != null)
child.setParent(node.getParent( )); // child’s grandparent becomes its parent
if (node == root)
root = child; // child becomes root
else {
Node<E> parent = node.getParent( );
if (node == parent.getLeft( ))
parent.setLeft(child);
else
parent.setRight(child);
}
size--;
E temp = node.getElement( );
node.setElement(null); // help garbage collection
node.setLeft(null);
node.setRight(null);
node.setParent(node); // our convention for defunct node
return temp;
}
}
对此,我们将不胜感激,谢谢。
您可以使用简单的递归来实现。
public String toString(){
String stringVal = "[";
if(root != null){
stringVal+=getChildNoteString(root);
}
return stringVal+"]";
}
private String getChildNodeString(Node n){
String nodeStringVal = n+",";
if(n.getLeft()!=null){
nodeStringVal += ","+ getChildNodeString(n.getLeft());
}
if(n.getRight()!=null){
nodeStringVal += ","+ getChildNodeString(n.getRight());
}
}
在LinkedBinaryTree<E>.class
某个位置,您需要重写toString()
,例如:
@Override // Not strictly necessary but good practice.
public String toString() {
return "Hello World!"; // Replace this with what you want to be returned.
}
您将需要定义该方法返回的值以满足您的需求。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.