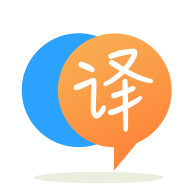
[英]Why does the 'replaceAll' method not add an empty space at the beginning of the String?
[英]Why does the list appear empty while trying to add nodes in the beginning?
我真的失去了理智,想弄清楚为什么我的add()
和print()
方法不起作用。 我几乎尝试了所有东西,但我不能这样做。 我知道我的代码是错误的(我甚至无法判断我的代码是否正确,因为我删除它以尝试新的东西)所以它可能有什么问题?
感谢您抽出宝贵时间阅读。
NodeFN类:
public class NodeFN {
private String data; // Data for node.
private NodeFN next; // Next node.
public NodeFN(String data) {
this.data = data; // Take the data value passed in & store it in the data field.
this.next = null; // Take the next node & store it in the next field.
}
// Mutator functions.
public String getData() {return data;}
public NodeFN getNext() {return next;}
public void setData(String d) {data = d;}
public void setNext(NodeFN n) {next = n;}
}
队列类:
public class Queue {
NodeFN head; // Head of node.
public String n;
public Queue(String n) {
head = new NodeFN(n); // head is now an object of NodeFN which holds a string.
}
public void add(String n) {
NodeFN nn = new NodeFN(n); // nn is now an object of NodeFN which holds a string, it should return something.
if(head == null) {
head = nn;
}
while(nn.getData().compareTo(head.getData()) < 0) {
nn.setNext(head); // Put node in beginning of the list.
nn.setData(n);
}
}
public void print() {
NodeFN nn = new NodeFN(n);
while(nn != null) {
nn.getNext().getData();
System.out.println(nn.getData() + " ");
}
}
public static void main(String[] args) {
Queue q = new Queue("string to test");
q.add("another string to test if add method works.");
q.print();
}
}
您正在创建链接列表。 为了打印它,您需要循环遍历列表,直到在getNext()
达到null。 基本上,你应该得到这样的东西:
public void print() {
NodeFN current = head;
while(current != null) {
System.out.println(current.getData());
current = current.getNext();
}
}
至于你的add()
方法,我们的想法是基本上把任何新节点作为列表中最后一个节点的next
引用。 只需保留对Queue
类中last
节点的引用。 添加时,将last
节点的next
设置为新创建的节点,并将新节点设置为last
节点。
我不能为你的add方法讲,但什么是n
在这里?
public void print() {
NodeFN nn = new NodeFN(n);
while(nn != null) {
nn.getNext().getData();
System.out.println(nn.getData() + " ");
}
}
队列类根本不应该关心public String n
。 您只需要head
节点。
然后, nn.getNext().getData();
回来了,是吗? 但是,你不打印它,也不是你在列表中“前进”。 (您不将nn
分配给下一个节点)。
尝试这样的事情
public void print() {
if (head == null) System.out.println("()");
NodeFN tmp = head;
while(tmp != null) {
System.out.println(tmp.getData() + " ");
tmp = tmp.getNext();
}
}
如果您希望在列表的开头添加节点,那么这应该可行。
public void add(String n) {
NodeFN nn = new NodeFN(n);
if(head == null) {
head = nn;
}
// Don't use a while loop, there is nothing to repeat
if (n.compareTo(head.getData()) < 0) {
// Both these operations put 'nn' in beginning of the list.
nn.setNext(head);
head = nn;
}
}
对于add方法,您有正确的想法开始。 如果列表中没有元素,请将头部设为nn
。 否则,遍历列表到最后一个元素并将其添加到结尾(因为它是一个队列)。
public void add(String n) {
NodeFN nn = new NodeFN(n);
if(head == null) {
head = nn;
}
else {
NodeFN cursor = this.head;
while(cursor.getNext() != null) {
cursor = cursor.getNext();
}
cursor.setNext(nn);
}
}
如果你想将新节点添加到开头(出于任何奇怪的原因),它会更容易。
public void add(String n) {
NodeFN nn = new NodeFN(n);
nn.setNext(this.head);
this.head = nn;
}
对于打印方法。 您没有设置nn
(光标)来引用实际队列中的任何节点。 您应该将nn
设置为队列的头部,然后迭代队列。 NodeFN nn = this.head
。 然后在while循环的主体中,打印数据nn.getData()
,然后移动到下一个节点nn = nn.getNext()
。
public void print() {
NodeFN cursor= this.head;
while(cursor != null) {
System.out.println(cursor.getData() + " ");
cursor= cursor.getNext();
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.