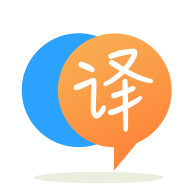
[英]Problem using Gradle and Mapstruct and Lombok and Spring Boot (No implementation for mapper)
[英]Using lombok with gradle and spring-boot
我正在尝试使用 lombok 构建一个项目,这就是我的依赖项。
dependencies {
compile("org.springframework.boot:spring-boot-starter-thymeleaf")
compile("org.springframework.social:spring-social-facebook")
compile("org.springframework.social:spring-social-twitter")
testCompile("org.springframework.boot:spring-boot-starter-test")
testCompile("junit:junit")
compile("org.springframework.boot:spring-boot-devtools")
compile("org.springframework.boot:spring-boot-starter-data-jpa")
compile("mysql:mysql-connector-java")
compileOnly("org.projectlombok:lombok:1.16.10")
}
我能够包含注释,并且我在编辑器中包含了 lombok。 我什至能够使用 lombok 编译代码并对 lombok 生成的方法进行校准。
这是我的实体:
@Data
@Entity
@Table(name = "TEA_USER", uniqueConstraints = {
@UniqueConstraint(columnNames = { "USR_EMAIL" }),
@UniqueConstraint(columnNames = { "USR_NAME" })
})
public class User {
@NotNull
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
@Column(name="USR_ID")
private long id;
@NotNull
@Column(name="USR_FNAME")
private String firstName;
@NotNull
@Column(name="USR_LNAME")
private String lastName;
@NotNull
@Min(5)
@Max(30)
@Column(name="USR_NAME")
private String username;
@Column(name="USR_EMAIL")
private String email;
@Min(8)
@NotNull
@Column(name="USR_PASSWORD")
private String password;
}
这是一个编译良好的函数:
@PostMapping("/registration/register")
public String doRegister (@ModelAttribute @Valid User user, BindingResult result){
user.getEmail();
System.out.println(user.getFirstName());
if (result.hasErrors()) {
return "register/customRegister";
}
this.userRepository.save(user);
return "register/customRegistered";
}
但是当我运行 bootRun 并尝试访问功能时,这是我得到的异常:
org.springframework.beans.NotReadablePropertyException: Invalid property 'firstName' of bean class [com.lucasfrossard.entities.User]: Bean property 'firstName' is not readable or has an invalid getter method: Does the return type of the getter match the parameter type of the setter?
但是,如果我手动包含 setter 和 getter,这可以正常工作。 我不明白发生了什么以及如何解决它。 有什么想法吗?
使用最新的 Lombok 1.18,这很简单。 io.franzbecker.gradle-lombok
插件不是必需的。 我的项目中的示例依赖项:
dependencies {
implementation "org.springframework.boot:spring-boot-starter-thymeleaf"
implementation "org.springframework.social:spring-social-facebook"
implementation "org.springframework.social:spring-social-twitter"
implementation "org.springframework.boot:spring-boot-starter-data-jpa"
testImplementation "org.springframework.boot:spring-boot-starter-test"
runtimeClasspath "org.springframework.boot:spring-boot-devtools"
runtime "mysql:mysql-connector-java"
// https://projectlombok.org
compileOnly 'org.projectlombok:lombok:1.18.4'
annotationProcessor 'org.projectlombok:lombok:1.18.4'
}
其他建议:
testCompile("junit:junit")
不是必需的,因为spring-boot-starter-test
“Starter” 包含 JUnit 。implementation
或api
配置而不是compile
(自Gradle 3.4 起)。 我该如何选择合适的? 不要对devtools
使用compile
配置。 来自开发者工具指南的提示:
在 Maven 中将依赖项标记为可选或在 Gradle 中使用自定义
developmentOnly
配置(如上所示)是一种最佳实践,可以防止 devtools 被传递地应用于使用您项目的其他模块。
如果您使用 IntelliJ IDEA,请确保已安装Lombok 插件并启用注释处理。 为了让新手更容易进行初始项目设置,您还可以根据需要指定 Lombok 插件。
在为此苦苦挣扎了一段时间后,我发现这个插件可以解决问题:
https://github.com/franzbecker/gradle-lombok
我的 gradle 文件看起来像这样:
buildscript {
repositories {
maven { url "https://repo.spring.io/libs-milestone" }
maven { url "https://plugins.gradle.org/m2/" }
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:1.4.0.RELEASE")
classpath 'org.springframework:springloaded:1.2.6.RELEASE'
}
}
plugins {
id 'io.franzbecker.gradle-lombok' version '1.8'
id 'java'
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'idea'
apply plugin: 'spring-boot'
jar {
baseName = 'gs-accessing-facebook'
version = '0.1.0'
}
repositories {
mavenCentral()
maven { url "https://repo.spring.io/libs-milestone" }
}
sourceCompatibility = 1.8
targetCompatibility = 1.8
dependencies {
// compile("org.projectlombok:lombok:1.16.10")
compile("org.springframework.boot:spring-boot-starter-thymeleaf")
compile("org.springframework.social:spring-social-facebook")
compile("org.springframework.social:spring-social-twitter")
testCompile("org.springframework.boot:spring-boot-starter-test")
testCompile("junit:junit")
compile("org.springframework.boot:spring-boot-devtools")
compile("org.springframework.boot:spring-boot-starter-data-jpa")
compile("mysql:mysql-connector-java")
}
idea {
module {
inheritOutputDirs = false
outputDir = file("$buildDir/classes/main/")
}
}
bootRun {
addResources = true
jvmArgs "-agentlib:jdwp=transport=dt_socket,server=y,suspend=y,address=5005"
}
我以前遇到过这个插件,但我做错了,它没有用。 我很高兴它现在起作用了。
谢谢!
如果您使用的是Eclipse
或其一个分支(我使用的是 Spring Tool Suite 4.5.1.RELEASE
, - 同样的步骤适用于另一个其他版本),您需要做的就是:
在build.gradle
,你只需要这样的配置:
dependencies { compileOnly 'org.projectlombok:lombok' annotationProcessor 'org.projectlombok:lombok' }
然后,右键单击您的项目 > Gradle > 刷新 Gradle 项目。 lombok-"version".jar
将出现在您项目的项目和外部依赖项中
右键单击lombok-"version".jar
> Run As > Java Application(类似于双击实际 jar 或在命令行上运行java -jar lombok-"version".jar
。)
将出现一个 GUI,按照所有说明进行操作,其中一件事就是将lombok.jar
复制到 IDE 的根文件夹中。
您唯一需要做的另一件事是将该lombok.jar
添加到您的项目构建/类路径中。
就是这样,干杯!
PS 有关更多信息,您可以访问此页面,尽管它不是必需的。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.