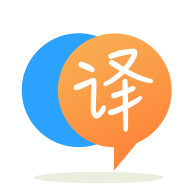
[英]Add custom WooCommerce registration fields to admin WordPress user contact fields
[英]How to add custom fields to WooCommerce registration form
我见过类似的问题,但找不到适合我的解决方案。
我正在尝试将自定义字段添加到 WooCommerce 注册表单,特别是名字和姓氏字段。 我已经设法创建了这些字段,但是当用户登录时,输入的信息不会传递到“帐户详细信息”页面。其他教程提到了验证这些字段,但我不确定它是否与我相关。 我正在研究 Wordpress 子主题。
请访问codepad .org查看代码。 我尝试使用代码示例选项将代码粘贴到此处,但无法正常工作。
希望我已经清楚地解释了自己。 如果没有,请告诉我,我会澄清。
我认为您已经覆盖了woocommerce/templates/myaccount/form-login.php
模板,并且通过这样做您已经设法显示billing_first_name
和billing_last_name
但您忘记使用woocommerce_created_customer
钩子,这是将这些数据保存到您的数据库中所需的。
我建议您保留模板并通过function.php
添加这些字段
这是在 WooCommerce 注册表单中添加自定义字段的代码:
/**
* To add WooCommerce registration form custom fields.
*/
function text_domain_woo_reg_form_fields() {
?>
<p class="form-row form-row-first">
<label for="billing_first_name"><?php _e('First name', 'text_domain'); ?><span class="required">*</span></label>
<input type="text" class="input-text" name="billing_first_name" id="billing_first_name" value="<?php if (!empty($_POST['billing_first_name'])) esc_attr_e($_POST['billing_first_name']); ?>" />
</p>
<p class="form-row form-row-last">
<label for="billing_last_name"><?php _e('Last name', 'text_domain'); ?><span class="required">*</span></label>
<input type="text" class="input-text" name="billing_last_name" id="billing_last_name" value="<?php if (!empty($_POST['billing_last_name'])) esc_attr_e($_POST['billing_last_name']); ?>" />
</p>
<div class="clear"></div>
<?php
}
add_action('woocommerce_register_form_start', 'text_domain_woo_reg_form_fields');
关于验证问题的第二部分,它是完全可选的,取决于您想要什么的业务逻辑,一般来说,大多数站点都需要名字和姓氏,但同样完全取决于您,如果您不这样做不想验证这一点,然后从上面的代码中删除<span class="required">*</span>
并跳过本节。
/**
* To validate WooCommerce registration form custom fields.
*/
function text_domain_woo_validate_reg_form_fields($username, $email, $validation_errors) {
if (isset($_POST['billing_first_name']) && empty($_POST['billing_first_name'])) {
$validation_errors->add('billing_first_name_error', __('<strong>Error</strong>: First name is required!', 'text_domain'));
}
if (isset($_POST['billing_last_name']) && empty($_POST['billing_last_name'])) {
$validation_errors->add('billing_last_name_error', __('<strong>Error</strong>: Last name is required!.', 'text_domain'));
}
return $validation_errors;
}
add_action('woocommerce_register_post', 'text_domain_woo_validate_reg_form_fields', 10, 3);
现在这是一个主要部分,这是您错过的部分,需要以下代码来保存自定义数据:
/**
* To save WooCommerce registration form custom fields.
*/
function text_domain_woo_save_reg_form_fields($customer_id) {
//First name field
if (isset($_POST['billing_first_name'])) {
update_user_meta($customer_id, 'first_name', sanitize_text_field($_POST['billing_first_name']));
update_user_meta($customer_id, 'billing_first_name', sanitize_text_field($_POST['billing_first_name']));
}
//Last name field
if (isset($_POST['billing_last_name'])) {
update_user_meta($customer_id, 'last_name', sanitize_text_field($_POST['billing_last_name']));
update_user_meta($customer_id, 'billing_last_name', sanitize_text_field($_POST['billing_last_name']));
}
}
add_action('woocommerce_created_customer', 'text_domain_woo_save_reg_form_fields');
以上所有代码都在您的活动子主题(或主题)的function.php
文件中。 或者也可以在任何插件 php 文件中。
代码已经过测试并且功能齐全。
希望这有帮助!
<?php
/**
* Add new register fields for WooCommerce registration
* To add WooCommerce registration form custom fields.
*/
add_action( 'woocommerce_register_form', 'misha_add_register_form_field' );
function misha_add_register_form_field(){
woocommerce_form_field(
'billing_first_name',
array(
'type' => 'text',
'required' => true, // just adds an "*"
'label' => 'First name'
),
( isset($_POST['billing_first_name']) ? $_POST['billing_first_name'] : '' )
);
woocommerce_form_field(
'billing_last_name',
array(
'type' => 'text',
'required' => true, // just adds an "*"
'label' => 'Last name'
),
( isset($_POST['billing_last_name']) ? $_POST['billing_last_name'] : '' )
);
woocommerce_form_field(
'billing_phone',
array(
'type' => 'tel',
'required' => true, // just adds an "*"
'label' => 'Phone'
),
( isset($_POST['billing_phone']) ? $_POST['billing_phone'] : '' )
);
}
/**
* To validate WooCommerce registration form custom fields.
*/
add_action( 'woocommerce_register_post', 'misha_validate_fields', 10, 3 );
function misha_validate_fields( $username, $email, $errors ) {
if ( empty( $_POST['billing_first_name'] ) ) {
$errors->add( 'billing_first_name_error', 'First name is required!' );
}
if ( empty( $_POST['billing_last_name'] ) ) {
$errors->add( 'billing_last_name_error', 'Last name is required!' );
}
if ( empty( $_POST['billing_phone'] ) ) {
$errors->add( 'billing_phone_error', 'Phone is required!' );
}
}
/**
* To save WooCommerce registration form custom fields.
*/
add_action( 'woocommerce_created_customer', 'misha_save_register_fields' );
function misha_save_register_fields( $customer_id ){
//First name field
if ( isset( $_POST['billing_first_name'] ) ) {
//update_user_meta( $customer_id, 'country_to_visit', wc_clean( $_POST['country_to_visit'] ) );
update_user_meta($customer_id, 'first_name', sanitize_text_field($_POST['billing_first_name']));
update_user_meta($customer_id, 'billing_first_name', sanitize_text_field($_POST['billing_first_name']));
}
//Last name field
if (isset($_POST['billing_last_name'])) {
update_user_meta($customer_id, 'last_name', sanitize_text_field($_POST['billing_last_name']));
update_user_meta($customer_id, 'billing_last_name', sanitize_text_field($_POST['billing_last_name']));
}
// WooCommerce billing phone
if ( isset( $_POST['billing_phone'] ) ) {
update_user_meta( $customer_id, 'billing_phone', sanitize_text_field( $_POST['billing_phone'] ) );
}
}
?>
使用过滤器钩子woocommerce_forms_field
添加 Woocommerce 注册表单自定义字段并使用随机数验证保存数据。
/**
* Adding more fields to Woocommerce Registration form and My account page
*/
function how_woocommerce_registration_form_fields() {
return apply_filters( 'woocommerce_forms_field', array(
'billing_company' => array(
'type' => 'text',
'label' => __( 'Company Name', ' how' ),
'required' => false,
),
'how_user_job_title' => array(
'type' => 'text',
'label' => __( 'Job Title', ' how' ),
'required' => false,
),
'how_user_industry' => array(
'type' => 'select',
'label' => __( 'Industry', 'how' ),
'options' => array(
'' => __( 'Select an Industry', 'how' ),
'Lending' => __( 'Lending', 'how' ),
'Real Estate' => __( 'Real Estate', 'how' ),
'Investment' => __( 'Investment', 'how' ),
'Servicing' => __( 'Servicing', 'how' ),
'Other' => __( 'Other', 'how' ),
'Mortgage Servicing' => __( 'Mortgage Servicing', 'how' ),
'Mortgage Lending' => __( 'Mortgage Lending', 'how' ),
)
)
) );
}
function how_woocommerce_edit_registration_form() {
$fields = how_woocommerce_registration_form_fields();
foreach ( $fields as $key => $field_args ) {
woocommerce_form_field( $key, $field_args );
}
}
add_action( 'woocommerce_register_form', 'how_woocommerce_edit_registration_form', 15 );
/**
* Save registration form custom fields
*/
function wooc_save_extra_register_fields( $customer_id ) {
if( wp_verify_nonce( sanitize_text_field( $_REQUEST['woocommerce-register-nonce'] ), 'woocommerce-register' ) ) {
if ( isset( $_POST['billing_company'] ) ) {
update_user_meta( $customer_id, 'billing_company', sanitize_text_field( $_POST['billing_company'] ) );
update_user_meta( $customer_id, 'shipping_company', sanitize_text_field( $_POST['billing_company'] ) );
}
if ( isset( $_POST['how_user_job_title'] ) ) {
update_user_meta( $customer_id, 'how_user_job_title', sanitize_text_field( $_POST['how_user_job_title'] ) );
}
if ( isset( $_POST['how_user_industry'] ) ) {
update_user_meta( $customer_id, 'how_user_industry', sanitize_text_field( $_POST['how_user_industry'] ) );
}
}
}
add_action( 'woocommerce_created_customer', 'wooc_save_extra_register_fields' );
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.