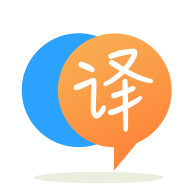
[英]How to correctly change MinSDKVersion from 15 to 16 for firebase
[英]Changing minSdkVersion from 15 to 16
当我更改我的minSdkVersion然后我收到此错误:
android.view.InflateException:二进制XML文件行#43:错误膨胀类TextView
在我做出改变之前,它的工作正常。
这是我的风格:
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- All customizations that are NOT specific to a particular API-level can go here. -->
</style>
<style name="AppTheme.Dark" parent="Theme.AppCompat.NoActionBar">
<item name="colorPrimary">@color/primary</item>
<item name="colorPrimaryDark">@color/primary_dark</item>
<item name="colorAccent">@color/white</item>
<item name="colorControlNormal">@color/greyDark</item>
<item name="colorControlActivated">@color/off_white</item>
<item name="android:windowBackground">@color/pink</item>
<item name="android:fontFamily">sans-serif-light</item>
<item name="android:textStyle">normal</item>
<item name="android:windowContentTransitions" tools:targetApi="lollipop">true</item>
<item name="android:windowAllowEnterTransitionOverlap" tools:targetApi="lollipop">true</item>
<item name="android:windowAllowReturnTransitionOverlap" tools:targetApi="lollipop">true</item>
<item name="android:windowSharedElementEnterTransition" tools:targetApi="lollipop">@android:transition/move</item>
<item name="android:windowSharedElementExitTransition" tools:targetApi="lollipop">@android:transition/move</item>
</style>
<style name="AlertDialogCustom" parent="@android:style/Theme.Dialog">
<item name="android:textColor">@color/pink</item>
<item name="android:typeface">normal</item>
<item name="android:height">5dp</item>
<item name="android:textSize">20sp</item>
<item name="android:textStyle">bold</item>
<item name="android:background">@color/white</item>
</style>
我想样式的父属性有问题。
如果您正在更改您的版本,那么还要更改支持库。您必须更改支持库,例如,对于egfor api 23,您必须将appcompact库版本更改为23.希望此帮助。:)
你应该尝试改变
<style name="AlertDialogCustom" parent="@android:style/Theme.Dialog">
至
<style name="AlertDialogCustom" parent="Theme.AppCompat.Dialog">
这是您正在使用的字体系列的问题。 您的assests >> fonts文件夹中可能没有sans-serif-light字体。 所以请查看如何在android中使用自定义字体。
以下是要遵循的步骤:
Android Studio:
第1步:将字体系列文件添加到应用程序
A)转到(项目文件夹)
B)然后app> src> main
C)在主文件夹中创建文件夹'assets> fonts'。
D)将'abc.ttf'或'abc.otf'字体文件放入fonts文件夹中。
第2步:现在在res文件夹下创建attrs.xml(如果它不存在)并添加declare-styleable
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="Font">
<attr name="typeface">
<enum name="FuturaLT" value="0" />
<enum name="FuturaLT_Heavy" value="1" />
<enum name="FuturaLT_Light" value="2" />
</attr>
</declare-styleable>
</resources>
//这里的FuturaLT / FuturaLT_Heavy / FuturaLT_Light是文件名
注意: - 枚举名称应该是下划线(_)使用的字体类型名称,您可以很容易地理解本机代码中的字体系列用法。 没有特殊字符或其他字母,否则你会在gen文件夹中获得相同属性id的错误。
第3步:创建自定义视图(Button,TextView,EditText)类: -
package com.myapp.views;
import android.content.Context;
import android.content.res.TypedArray;
import android.util.AttributeSet;
import android.widget.TextView;
import com.myapp.R;
public class CustomTextView extends TextView {
public CustomTextView(Context context) {
super(context);
}
public CustomTextView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public CustomTextView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
try {
TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.Font);
int font = a.getInt(R.styleable.Font_typeface, 0);
a.recycle();
String str;
switch (font) {
case 1:
str = "fonts/FuturaLT.otf";
break;
case 2:
str = "fonts/FuturaLT_Heavy.otf";
break;
case 3:
str = "fonts/FuturaLT_Light.otf";
break;
default:
str = "fonts/FuturaLT.otf";
break;
}
setTypeface(FontManager.getInstance(getContext()).loadFont(str));
} catch (Exception e) {
e.printStackTrace();
}
}
@SuppressWarnings("unused")
private void internalInit(Context context, AttributeSet attrs) {
}
}
第4步:添加FontManager.java支持类
package com.myapp.views;
import android.content.Context;
import android.graphics.Typeface;
import java.util.HashMap;
import java.util.Map;
public class FontManager {
private Map<String, Typeface> fontCache = new HashMap<String, Typeface>();
private static FontManager instance = null;
private Context mContext;
private FontManager(Context mContext2) {
mContext = mContext2;
}
public synchronized static FontManager getInstance(Context mContext) {
if (instance == null) {
instance = new FontManager(mContext);
}
return instance;
}
public Typeface loadFont(String font) {
if (false == fontCache.containsKey(font)) {
fontCache.put(font, Typeface.createFromAsset(mContext.getAssets(), font));
}
return fontCache.get(font);
}
}
第5步:在XML布局中使用
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.myapp.views.CustomTextView
android:id="@+id/tv_time_slot"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
app:typeface="FuturaLT" />
</LinearLayout>
您也可以直接在java代码中使用:
Typeface tf = Typeface.createFromAsset(getAssets(), "fonts/FuturaLT.ttf");
tvText.setTypeface(tf);
注意: -如果您不使用FontManager应用字体并直接使用,那么您将无法在图形预览中看到您的视图。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.