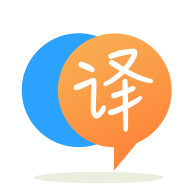
[英]count total number of children and the total number of objects and split into different array
[英]Count the total number of strings in object and all children
我有一个对象,可能包含对象(反过来可能包含或不包含对象,等等到无穷大),并带有字符串,如下所示:
var obj = {
"foo": "hello",
"bar": "hi",
"test": {
"foo": "foo"
"bar": {
"test": "hi",
"hello": "howdy"
}
}
}
我想要做的是计算整个obj
对象及其子对象中的字符串数。 在这个例子中,正确的答案是5。
关于计算此站点上的对象中的键的众多主题都建议使用.hasOwnProperty
的循环或新的Object.keys(obj)
方式,但这些都不是递归的,并且它们都计算子对象本身。
完成此任务的最佳方法是什么?
您可以创建递归函数,循环嵌套对象并返回计数。
var obj = { "foo": "hello", "bar": "hi", "test": { "foo": "foo", "bar": { "test": "hi", "hello": "howdy" } } } function countS(data) { var c = 0; for (var i in data) { if (typeof data[i] == 'object') c += countS(data[i]); if (typeof data[i] == 'string') c += 1 } return c; } console.log(countS(obj))
这是功能编程风格的ES6功能:
function countPrimitives(obj) { return +(Object(obj) !== obj) || Object.keys(obj).reduce( (cnt, key) => cnt + countPrimitives(obj[key]), 0 ); } var obj = { "foo": "hello", "bar": "hi", "test": { "foo": "foo", "bar": { "test": "hi", "hello": "howdy" } } }; console.log(countPrimitives(obj));
实际上,这也将计算其他原始值,包括数字和布尔值,......:任何不是嵌套对象的东西。
您可以使用Array#reduce
迭代键并检查Object。
function getCount(object) { return Object.keys(object).reduce(function (r, k) { return r + (object[k] && typeof object[k] === 'object' ? getCount(object[k]) : 1); }, 0); } var obj = { foo: "hello", bar: "hi", test: { foo: "foo", bar: { test: "hi", hello: "howdy" } } }, count = getCount(obj); console.log(count);
使用您提到的任何一种方法循环。
像这样的东西:
var obj = { "foo": "hello", "bar": "hi", "test": { "foo": "foo", "bar": { "test": "hi", "hello": "howdy" } } } function count_strings(obj) { var count = 0; var keys = Object.keys(obj); for (var i=0, l=keys.length; i<l; i++) { if (typeof obj[keys[i]] === 'object') { count += count_strings(obj[keys[i]]); } else if (typeof obj[keys[i]] === 'string') { count++; } } return count; } document.querySelector('.output').innerHTML = count_strings(obj);
<div class="output"></div>
这也是我的50分。
function checkString(str){
var count = 0;
if(typeof str === 'string'){
return 1;
}
Object.keys(str).forEach(function(v){
console.log(str[v]);
if(typeof str[v] === 'object') count = count + checkString(str[v])
else count ++;
})
return count;
}
var obj = {
"foo": "hello",
"bar": "hi",
"test": {
"foo": "foo"
"bar": {
"test": "hi",
"hello": "howdy"
}
}
};
console.log(checkString(obj));
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.