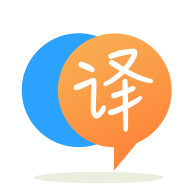
[英]Is there a way to print a toString from a return statement without overriding a toString method?
[英]Return statement in a toString class
所以我有一个Card类,看起来像这样:
public class Card
{
//instance variables
private String faceValue; //the face value of the card
private String suit; //the suit of the card
String[] ranks = {"Ace", "2", "3", "4", "5", "6","7", "8", "9", "10", "Jack", "Queen", "King"};
String[] suits = {"Clubs", "Diamonds", "Hearts", "Spades"};
/**
* Constructor
*/
public Card(int aValue, int aSuit)
{
faceValue = ranks[aValue];
suit = suits[aSuit];
}
//getters
/**
* Getter for faceValue.
*/
public String getFaceValue()
{
return faceValue;
}
/**
* Getter for suit.
*/
public String getSuit()
{
return suit;
}
//end of getters
//methods
/**
* This method returns a String representation of a Card object.
*
* @param none
* @return String
*/
public String toString()
{
return "A card: " + faceValue + " of " + suit;
}
}
还有一个Deck类,如下所示:
public class Deck
{
//instance variables
private Card[] deck;
/**
* Constructor for objects of class Deck
*/
public Deck()
{
deck = new Card[52];
int cardCount = 0; //number of cards created so far.
for (int aSuit = 0; aSuit < 4; aSuit++ )
{
for ( int aValue = 0; aValue < 13; aValue++ ) {
deck[cardCount] = new Test(aValue, aSuit);
cardCount++;
}
}
}
/**
* String representation.
*/
public String toString()
{
for (int i = 0; i < 52; i++)
{
String v = deck[i].getFaceValue();
String s = deck[i].getSuit();
return "Dealt a card: " + v + " of " + s + ".";
}
}
}
我在套牌中的toString方法显示错误“缺少返回语句”。 在每次循环后仍允许其打印卡详细信息的同时,如何更改return语句?
您编写的代码将仅从卡座数组返回第0个元素。 它应该像这样:
public String toString()
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 52; i++)
{
String v = deck[i].getFaceValue();
String s = deck[i].getSuit();
sb.append("Dealt a card: ").append(v).append(" of ").append(s).append(".\n");
}
return sb.toString();
}
该错误是因为您的toString
方法具有返回类型String。
Java检查执行路径。 如果for
循环结束并且从不返回任何内容怎么办?
尽管您可能已经对该逻辑进行了硬编码,但是Java编译器并未对此进行统计分析。 因此,它必须返回String类型,如果没有其他作用,则必须执行。
这将起作用:
public String toString()
{
for (int i = 0; i < 52; i++)
{
String v = deck[i].getFaceValue();
String s = deck[i].getSuit();
return "Dealt a card: " + v + " of " + s + ".";
}
return "";
}
但是我想你想要的是:
public String toString()
{
StringBuilder returnValue = new StringBuilder("");
for (int i = 0; i < 52; i++)
{
String v = deck[i].getFaceValue();
String s = deck[i].getSuit();
returnValue.append("Dealt a card: " + v + " of " + s + ".");
}
return returnValue.toString();
}
如果您只想打印所有卡信息,则可以使用
public String toString(){
for (int i = 0; i < 52; i++)
{
String v = deck[i].getFaceValue();
String s = deck[i].getSuit();
System.out.println( "Dealt a card: " + v + " of " + s + ".");
}
return null;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.