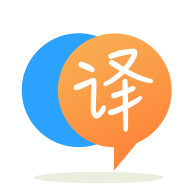
[英]SPRING: Requested bean is currently in creation: Is there an unresolvable circular reference
[英]Spring Boot + Quartz: "Requested bean is currently in creation: Is there an unresolvable circular reference?"
我已经按照本教程 ( spring-boot-quartz-demo ) 在我的 Spring Boot 应用程序中使用 Quartz Scheduler 实现了一个调度程序例程,并做了一些修改来满足我的目的。
例如,我的作业服务必须能够列出数据库中的所有对象及其子项,设置新值并最终更新。 所有这些都必须是交易性的。
出于某种原因,MyJob 类不允许在其中声明事务方法,因此我通过注入具有事务方法的新服务类来解决此问题。
这项工作运行良好,但每次运行应用程序时,它都会给我一些警告消息:
[main] WARN o.s.b.f.s.DefaultListableBeanFactory - Bean creation exception on FactoryBean type check: org.springframework.beans.factory.BeanCurrentlyInCreationException: Error creating bean with name 'testJobBean': Requested bean is currently in creation: Is there an unresolvable circular reference?
[main] WARN o.s.b.f.s.DefaultListableBeanFactory - Bean creation exception on FactoryBean type check: org.springframework.beans.factory.BeanCurrentlyInCreationException: Error creating bean with name 'testJobBean': Requested bean is currently in creation: Is there an unresolvable circular reference?
[main] WARN o.s.b.f.s.DefaultListableBeanFactory - Bean creation exception on FactoryBean type check: org.springframework.beans.factory.BeanCurrentlyInCreationException: Error creating bean with name 'testJobBean': Requested bean is currently in creation: Is there an unresolvable circular reference?
[main] WARN o.s.b.f.s.DefaultListableBeanFactory - Bean creation exception on FactoryBean type check: org.springframework.beans.factory.BeanCurrentlyInCreationException: Error creating bean with name 'testJobBean': Requested bean is currently in creation: Is there an unresolvable circular reference?
[main] WARN o.s.b.f.s.DefaultListableBeanFactory - Bean creation exception on FactoryBean type check: org.springframework.beans.factory.BeanCurrentlyInCreationException: Error creating bean with name 'testJobBean': Requested bean is currently in creation: Is there an unresolvable circular reference?
[main] WARN o.s.b.f.s.DefaultListableBeanFactory - Bean creation exception on FactoryBean type check: org.springframework.beans.factory.BeanCurrentlyInCreationException: Error creating bean with name 'testJobBean': Requested bean is currently in creation: Is there an unresolvable circular reference?
[main] WARN o.s.b.f.s.DefaultListableBeanFactory - Bean creation exception on FactoryBean type check: org.springframework.beans.factory.BeanCurrentlyInCreationException: Error creating bean with name 'testJobBeanTrigger': Requested bean is currently in creation: Is there an unresolvable circular reference?
我减少了警告消息: @DependsOn("testJobBean")
我注意到工作类中的自动装配服务导致了它,但为什么呢? 我必须做些什么才能摆脱此警告消息?
欢迎解决如何在作业类中声明@Transacional
方法!
QuartzConfig.java
@Configuration
@ConditionalOnProperty(name = "quartz.enabled")
public class QuartzConfig {
@Autowired
private DataSource dataSource;
@Autowired
private PlatformTransactionManager transactionManager;
@Autowired
private ApplicationContext applicationContext;
@Autowired
List<Trigger> triggers;
@Bean
public JobFactory jobFactory() {
AutowiringSpringBeanJobFactory jobFactory = new AutowiringSpringBeanJobFactory();
jobFactory.setApplicationContext(applicationContext);
return jobFactory;
}
@Bean
public SchedulerFactoryBean schedulerFactoryBean(JobFactory jobFactory) throws IOException {
SchedulerFactoryBean factory = new SchedulerFactoryBean();
factory.setOverwriteExistingJobs(true);
factory.setDataSource(dataSource);
factory.setTransactionManager(transactionManager);
factory.setJobFactory(jobFactory);
factory.setQuartzProperties(quartzProperties());
if (triggers != null && !triggers.isEmpty()) {
factory.setTriggers(((Trigger[]) triggers.toArray(new Trigger[triggers.size()])));
}
return factory;
}
@Bean
public Properties quartzProperties() throws IOException {
PropertiesFactoryBean propertiesFactoryBean = new PropertiesFactoryBean();
propertiesFactoryBean.setLocation(new ClassPathResource("/quartz.properties"));
propertiesFactoryBean.afterPropertiesSet();
return propertiesFactoryBean.getObject();
}
public static SimpleTriggerFactoryBean createTrigger(JobDetail jobDetail, long pollFrequencyMs) {
SimpleTriggerFactoryBean factoryBean = new SimpleTriggerFactoryBean();
factoryBean.setJobDetail(jobDetail);
factoryBean.setRepeatInterval(pollFrequencyMs);
factoryBean.setMisfireInstruction(SimpleTrigger.MISFIRE_INSTRUCTION_RESCHEDULE_NEXT_WITH_REMAINING_COUNT);
return factoryBean;
}
public static CronTriggerFactoryBean createCronTrigger(JobDetail jobDetail, String cronExpression) {
CronTriggerFactoryBean factoryBean = new CronTriggerFactoryBean();
factoryBean.setJobDetail(jobDetail);
factoryBean.setCronExpression(cronExpression);
factoryBean.setMisfireInstruction(SimpleTrigger.MISFIRE_INSTRUCTION_FIRE_NOW);
return factoryBean;
}
public static JobDetailFactoryBean createJobDetail(Class<?> jobClass) {
JobDetailFactoryBean factoryBean = new JobDetailFactoryBean();
factoryBean.setJobClass(jobClass);
factoryBean.setDurability(true);
return factoryBean;
}
}
自动装配SpringBeanJobFactory.java
public final class AutowiringSpringBeanJobFactory extends SpringBeanJobFactory implements ApplicationContextAware {
private transient AutowireCapableBeanFactory beanFactory;
@Override
public void setApplicationContext(final ApplicationContext context) {
beanFactory = context.getAutowireCapableBeanFactory();
}
@Override
protected Object createJobInstance(final TriggerFiredBundle bundle) throws Exception {
final Object job = super.createJobInstance(bundle);
beanFactory.autowireBean(job);
return job;
}
}
我的工作
@Component
@DisallowConcurrentExecution
public class TestJob implements Job {
@Autowired
private TestService service;
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
service.execute();
}
@Bean(name = "testJobBean")
public JobDetailFactoryBean createJobDetail() {
return QuartzConfig.createJobDetail(this.getClass());
}
@DependsOn("testJobBean")
@Bean(name = "testJobBeanTrigger")
public CronTriggerFactoryBean createTrigger(@Qualifier("testJobBean") JobDetail jobDetail,
@Value("${quartz.job.test.cronExpression}") String cronExpression) {
return QuartzConfig.createCronTrigger(jobDetail, cronExpression);
}
}
我的服务
@Service
@Slf4j
public class MyService {
private TestRepository testRepository;
@Autowired
public MyService(TestRepository testRepository) {
this.testRepository = testRepository;
}
@Transactional(propagation = Propagation.REQUIRES_NEW, rollbackFor = Throwable.class)
public void execute() {
log.debug("Job has been started");
List<TestObject> list = testRepository.findAll();
if (list != null && !list.isEmpty()) {
System.out.println("\n");
list.forEach(obj -> {
System.out.println(
"TestObject ID: " + obj.getId() + "\n" +
"SubTestObjects IDs: " + (obj.getSubTestObjects() == null ? "null" : obj.getSubTestObjects().toString()) +
"AnotherSubTestObjects IDs: " + (obj.getAnotherSubTestObjects() == null ? "null" : obj.getAnotherSubTestObjects().toString())
);
});
System.out.println();
log.info(list.size() + " test objects found");
} else {
log.info("No test objects were found");
}
log.debug("Job has been finished");
}
}
石英属性
#============================================================================
# Configure Main Scheduler Properties
#============================================================================
org.quartz.scheduler.instanceId = AUTO
org.quartz.scheduler.makeSchedulerThreadDaemon = true
#============================================================================
# Configure ThreadPool
#============================================================================
org.quartz.threadPool.class = org.quartz.simpl.SimpleThreadPool
org.quartz.threadPool.threadCount = 1
org.quartz.threadPool.makeThreadsDaemons = true
#============================================================================
# Configure JobStore
#============================================================================
org.quartz.jobStore.class = org.quartz.impl.jdbcjobstore.JobStoreTX
org.quartz.jobStore.driverDelegateClass = org.quartz.impl.jdbcjobstore.PostgreSQLDelegate
org.quartz.jobStore.useProperties = true
org.quartz.jobStore.tablePrefix = QRTZ_
org.quartz.jobStore.isClustered = true
org.quartz.jobStore.misfireThreshold = 60000
org.quartz.jobStore.clusterCheckinInterval 3600000
看起来问题可能出在createJobDetail
方法中,请考虑以下代码段:
@Bean(name = "testJobBean")
public JobDetailFactoryBean createJobDetail() {
return QuartzConfig.createJobDetail(this.getClass());
}
@DependsOn("testJobBean")
@Bean(name = "testJobBeanTrigger")
public CronTriggerFactoryBean createTrigger(@Qualifier("testJobBean") JobDetail jobDetail,
@Value("${quartz.job.test.cronExpression}") String cronExpression) {
return QuartzConfig.createCronTrigger(jobDetail, cronExpression);
}
它在它的实现中使用this
来保存对当前对象的引用。 但是,当前对象当时也可能正在创建中,从而导致此错误。 我会建议将这两个移动到Config
类。
此外,使用@DependsOn
是一个好习惯。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.