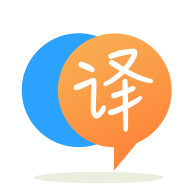
[英]How set text in textview and formatted it as code? (Android Java)
[英]Set text for a textview in Java code
这是我的activity_main.xml
<EditText
android:layout_width="200dp"
android:layout_height="100dp"
android:hint="@string/str3"
android:id="@+id/line2"
android:layout_below="@id/line1" />
<Button
android:layout_width="wrap_content"
android:layout_height="100dp"
android:layout_toRightOf="@id/line2"
android:layout_below="@id/line1"
android:text="@string/str4"
android:onClick="method2"/>
我想显示在另一个活动中输入的文本,但不像活动教程中那样显示(在第二个活动中创建另一个 TextView 并且仅在那里设置文本)。
这是我的 display2.java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display2);
Intent intent2 = getIntent();
这是在我的activity_display2.xml
<TextView
android:id="@+id/pwdisplay"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="20sp"
android:textStyle="bold|italic"
android:padding="30dp"
/>
这就是我想要做的(在 main_activity.java 中)
public void method2(View view) {
Intent intent2 = new Intent(this, display2.class);
EditText enteredpw = (EditText) findViewById(R.id.line2);
String pw = enteredpw.getText().toString();
findViewById(R.id.pwdisplay).setText(pw); <--Error here
startActivity(intent2);
}
我试图在 main_activity.java 本身中设置pwdisplay
TextView 的文本,但收到错误“无法解析方法 setText(java.lang.String)” 。
或者
用户在 EditText @id/line2
输入的文本是否可以通过 activity_display2.xml 显示?
findViewById(R.id.pwdisplay)
返回View
。 View
没有setText
方法。 你需要投:
((TextView)findViewById(R.id.pwdisplay)).setText(pw);
您不能从另一个 Activity 操作一个 Activity 中的小部件。 要执行我认为您要执行的操作,您必须传递通过 Intent 输入的文本。 例如:
public void method2(View view) {
Intent intent2 = new Intent(this, display2.class);
EditText enteredpw = (EditText) findViewById(R.id.line2);
String pw = enteredpw.getText().toString();
intent2.putExtra("KEY", pw);
startActivity(intent2);
}
//display2.java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display2);
Intent intent2 = getIntent();
String pw = intent2.getStringExtra("KEY");
findViewById(R.id.pwdisplay).setText(pw);
您与您的代码不一致。 这解决了您的问题,因为您必须将 findViewById 转换为 TextView,否则编译器将不知道您正在处理 TextView。
Intent intent2 = new Intent(this, display2.class);
EditText enteredpw = (EditText) findViewById(R.id.line2);
String pw = enteredpw.getText().toString();
((TextView)findViewById(R.id.text)).setText(pw);
startActivity(intent2);
但话又说回来,你做这样的一切:
EditText enteredpw = (EditText) findViewById(R.id.line2);
您必须选择使用这样的简短版本:
((TextView)findViewById(R.id.text)).setText(pw);
或者
TextView textView = (TextView)findViewById(R.id.text);
textView.setText(pw);
但现在我读到你想将用户输入的文本发送到第二个活动。 这可以通过通过调用将其提供给意图来完成:
intent2.putExtra("text",pws);
并在新活动中使用它
Bundle extras = getIntent().getExtras();
String text;
if (extras != null) {
text = extras.getString("text");
// set the text to the textview.
((TextView)findViewById(R.id.text)).setText(text);
}
欢迎来到安卓开发
你不能从其他类似的活动中设置 TextView 的文本,你可以做一些事情,但我会向你解释最简单的。
在 MainActivity 中输入:
public void method2(View view) {
Intent intent2 = new Intent(this, display2.class);
EditText enteredpw = (EditText) findViewById(R.id.line2);
String pw = enteredpw.getText().toString();
intent2.putExtra("message", pw);
startActivity(intent2);
}
在你的 display2 类中
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display2);
String message;
message = getIntent().getExtras().getString("message");
TextView pwdisplay = (TextView) findViewById(R.id.pwdisplay);
pwdisplay.setText(message);
}
这是发生的事情,把你的 MainActivity 中的 Intent 想象成一封信,你可以在信中写一些你在 display2 活动中需要的东西,在这种情况下我们有intent2.putExtra("message", pw);
这是我们发送字符串的地方,在 display2 类中,我们在message = getIntent().getExtras().getString("message");
行中接收到它message = getIntent().getExtras().getString("message");
然后我们把这个字符串放在 TextView pwdisplay.setText(message);
.
您无法找到 ID 为“pwdisplay”的文本视图,因为它不在 main_activity 中,因此请修改您的代码,如下所示:
// MainActivity code
public void method2(View view) {
Intent intent2 = new Intent(this, display2.class);
EditText enteredpw = (EditText) findViewById(R.id.line2);
String pw = enteredpw.getText().toString();
Intent intent2 = new Intent(this, MainActivity.class);
// passed your entered text to the next activity using the line below:
// KEY= text_to_display, his value equal the entered text
intent2.putExtra("text_to_display","some text here");
startActivity(intent2);
}
// on display2.java "Activity" do modify it like below code:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display2);
Intent intent2 = getIntent();
// get the passed text from the given intent
String pw = intent2.getExtras().getString("text_to_display",null);
TextView myTxView = (TextView) findViewById(R.id.pwdisplay);
if(pw != null){
myTxView.setText(pw);
}
}
享受!
package com.kibjob50.provaspeec;
import android.content.ActivityNotFoundException;
import android.content.Intent;
import android.speech.RecognizerIntent;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;import android.os.Bundle;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import java.util.ArrayList;
import java.util.Locale;
public class MainActivity extends AppCompatActivity {
private final int REQ_CODE = 100;
TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.text);
ImageView speak = findViewById(R.id.speak);
speak.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH);
intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL,
RecognizerIntent.LANGUAGE_MODEL_FREE_FORM);
intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE, Locale.getDefault());
intent.putExtra(RecognizerIntent.EXTRA_PROMPT, "Need to speak");
try {
startActivityForResult(intent, REQ_CODE);
} catch (ActivityNotFoundException a) {
Toast.makeText(getApplicationContext(),
"Sorry your device not supported",
Toast.LENGTH_SHORT).show();
}
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
switch (requestCode) {
case REQ_CODE: {
if (resultCode == RESULT_OK && null != data) {
ArrayList result = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS);
textView.setText(result.get(0));
}
break;
}
}
}
}
上面的例子,在网上找到的,在语句“textView.setText(result.get(0));更改为“textView.setText((CharSequence) result.get(0));”时给出了同样的错误!我希望这可以帮到你。
使用黄油刀绑定视图:
@Bind(R.id.text_view) TextView myTextView;
然后在你的方法中:
myTextView.setText("Hello!");
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.