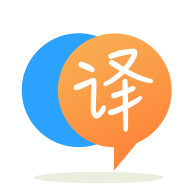
[英]How do I compare two values from two vectors and then update the vector values in C++?
[英]In C++, How do I store values into a vector that is inside of a vector when the two vectors are of different types?
我正在编写一个程序,需要使用以下数据结构:
struct shape
{
std::vector<float> verts; // contains the x & y values for each vertex
char type; // the type of shape being stored.
float shapeCol[3]; // stores the color of the shape being stored.
float shapeSize; // stores the size of the shape if it is a line or point
};
在我的主程序中,我需要一个shape
类型的向量。 如何使用结构形状的向量将值存储到结构形状内部的向量中。
例如, vector<shape> myshapes
;
如果我想的值存储到我的第一个索引verts
载体,我对我的第一个索引里面myshapes
矢量我会怎么做呢?
用伪代码看起来像这样, i
是索引:
myshapes[i].vector[i] = 4; // but I know this is incorrect
改为使用STL列表更容易实现吗?如果是,该语法将是什么样?
感谢您的帮助,我是vector的新手,所以我们将不胜感激。
vector
支持使用[]
运算符。 语法和语义与对数组使用[]
运算符非常相似。 请参阅: http : //en.cppreference.com/w/cpp/container/vector/operator_at 。
与任何struct成员一样,您需要按名称访问它。 myshapes[i].verts[j] = 4;
。
给出的一般建议是使用std::vector
作为您选择的默认容器。 自然,如果您有特定需求(例如在容器的中间添加/删除项目),其他容器可能具有更好的性能特征。
如果向量开始为空,则必须先向它们添加元素,然后才能使用operator[]
对其进行索引。 通常使用push_back
(添加现有shape
对象)或emplace_back
(直接在向量中构造新的shape
对象)完成此操作。
给定vector<shape> myshapes
,您可以添加一些形状,如下所示:
// add 10 shapes
for (size_t n = 0; n < 10; n++) {
shape s; // creates a new, blank shape object
// initialize the shape's data
s.type = ...;
s.shapeSize = ...;
// etc.
// add verts
s.verts.push_back(1.0f);
s.verts.push_back(2.0f);
s.verts.push_back(3.0f);
// etc.
// add the shape to the vector
myshapes.push_back(std::move(s));
}
(由于在最后一行使用s
完成,因此我们可以使用std::move
。这允许push_back
将形状的数据移动到向量中,而不是复制它。查找move语义以获取更多信息。)
一旦向量中包含内容,就可以按如下所示按索引访问元素:
myshapes[index of shape].verts[index of vertex within that shape]
将[]
与无效索引一起使用,或者当向量为空时,将调用未定义的行为 (不这样做,否则程序将崩溃/发生故障)。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.