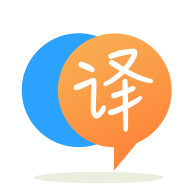
[英]How to store characters into a char pointer using the strcpy() function
[英]How to copy an array of characters to a char pointer without using strcpy
我将如何在不手动使用 strcpy 的情况下将 char 数组的字符复制到 char 指针中。 例如:
char *Strings[NUM];
char temp[LEN];
int i;
for (i = 0; i < NUM; i++){
fgets(temp, LEN, stdin);
Strings[i] = malloc(strlen(temp)+1);
Strings[i] = temp; // What would go here instead of this,
// because this causes this to happen->
}
Input:
Hello
Whats up?
Nothing
Output (when the strings in the array of char pointers are printed):
Nothing
Nothing
Nothing
我不知道如何解决这个问题。
在您的示例中,您使用以下两行:
Strings[i] = malloc(strlen(temp)+1); /* you should check return of malloc() */
Strings[i] = temp;
这是不正确的。 第二行只是覆盖了从malloc()
返回的指针。 您需要改为使用<string.h>
strcpy()
:
Strings[i] = malloc(strlen(temp)+1);
strcpy(Strings[i], temp);
char *strcpy(char *dest, const char *src)
将指向的字符串从src
复制到dest
。dest
是目标,src
是要复制的字符串。 返回一个指向dest
的指针。
您也没有检查fgets()
返回,它在失败时返回NULL
。 您还应该考虑删除fgets()
附加的\\n
字符,因为您复制到Strings[i]
将有一个尾随换行符,这可能不是您想要的。
由于另一个答案显示了如何手动完成,您可能还需要考虑仅使用strdup()
为您进行复制。
strdup()
返回一个指向与字符串str重复的新字符串的指针。 内存从malloc()
获得,并用free()
从堆中释放。
这是一些执行额外错误检查的示例代码。
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LEN 3
#define BUFFSIZE 20
int main(void) {
char *strings[LEN] = {NULL};
char buffer[BUFFSIZE] = {'\0'};
size_t slen, strcnt = 0, i;
printf("Input:\n");
for (i = 0; i < LEN; i++) {
if (fgets(buffer, BUFFSIZE, stdin) == NULL) {
fprintf(stderr, "Error from fgets()\n");
exit(EXIT_FAILURE);
}
slen = strlen(buffer);
if (slen > 0 && buffer[slen-1] == '\n') {
buffer[slen-1] = '\0';
} else {
fprintf(stderr, "Too many characters entered\n");
exit(EXIT_FAILURE);
}
if (*buffer) {
strings[strcnt] = strdup(buffer);
if (strings[strcnt] == NULL) {
fprintf(stderr, "Cannot allocate buffer\n");
exit(EXIT_FAILURE);
}
strcnt++;
}
}
printf("\nOutput:\n");
for (i = 0; i < strcnt; i++) {
printf("%s\n", strings[i]);
free(strings[i]);
strings[i] = NULL;
}
return 0;
}
所以发生的事情是你改变了 temp 的值,但所有的指针都指向 temp 的一个实例。 您需要分配内存,然后手动复制数组。
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main()
{
int LEN = 20;
int NUM = 3;
char* Strings[NUM];
char temp[LEN];
int i,j;
for (i=0;i<NUM;i++){
fgets(temp,LEN,stdin);
Strings[i] = (char*)malloc(strlen(temp)+1);
for(j=0;j<=strlen(temp);j++) { /* this part */
if (j == strlen(temp))
Strings[i][j - 1] = temp[j]; /* overwrite \n with the terminating \0 */
else
Strings[i][j] = temp[j];
}
}
for (i=0;i<NUM;i++)
printf("%s\n", Strings[i]);
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.