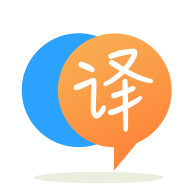
[英]How to find controls on a dynamically created user control in ASP.NET?
[英]How to dynamically create ASP.net controls within dynamically created ASP.net controls
我正在用ASP.net制作应用程序。 我有一个按钮,单击后会生成一些html,一些asp文本框和一个asp按钮(第二个按钮)。 据我所知,这工作正常。 现在我想要的是,当我单击第二个新创建的按钮时,我希望它创建一些html + asp.net文本框。
这让我感到困惑,有没有更简单的方法? 我似乎无法弄清楚,我为按钮2创建了onclick事件,但它尚不存在。
非常感谢。
认为以防万一,您想看一下代码可能会容易一些,以防万一。
namespace ConnorMackayWebForm
{
public partial class InspectionCreate : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
//Set the initial amount of areas and hazards
int areaCount = 0;
int hazardCount = 0;
//Check if the viewstate with the area count already exists
if (ViewState["areaCount"] != null)
{
//Convert the view state back to an int
areaCount = Convert.ToInt32(ViewState["areaCount"]);
}
else
{
ViewState["areaCount"] = areaCount;
}
//Check if the viewstate with the hazard count already exists
if (ViewState["hazardCount"] != null)
{
//Convert the view state back to an int
hazardCount = Convert.ToInt32(ViewState["hazardCount"]);
}
else
{
ViewState["hazardCount"] = hazardCount;
}
//Create the required number of areas
for (int i = 1; i <= areaCount; i++)
{
createArea(i);
}
//Create the required number of hazards
for (int i = 1; i <= hazardCount; i++)
{
createHazard(i);
}
}
protected void btnCreateArea_Click(object sender, EventArgs e)
{
//Get the current number of areas
int areaCount = Convert.ToInt32(ViewState["areaCount"]) + 1;
//Create the area
createArea(areaCount);
//Set the new area into the viewstate
ViewState["areaCount"] = areaCount;
}
protected void btnCreateHazard_Click(object sender, CommandEventArgs areaCount)
{
//Get the current number of areas
int hazardCount = Convert.ToInt32(ViewState["hazardCount"]) + 1;
//Get the argument from the button
int placeholderID = Convert.ToInt32(areaCount.CommandArgument);
//Create the hazard
createHazard(hazardCount, placeholderID);
//Set the new hazard into the viewstate
ViewState["hazardCount"] = hazardCount;
}
private void createArea(int areaCount)
{
//Start generating the HTML
pnlArea.Controls.Add(new LiteralControl("<div class='box'>"));
pnlArea.Controls.Add(new LiteralControl("<div class='box-header with-border'>"));
pnlArea.Controls.Add(new LiteralControl("<h2 class='box-title'>Area / Equipment Inspected 1: "));
//Create the title dropdown
DropDownList ddArea = new DropDownList();
ddArea.ID = "ddArea" + areaCount;
pnlArea.Controls.Add(ddArea);
//Create the Other textbox in the title
pnlArea.Controls.Add(new LiteralControl(" Other: "));
TextBox txtOther = new TextBox();
txtOther.ID = "txtOther" + areaCount;
pnlArea.Controls.Add(txtOther);
//Generate HTML for the box body and begining of first column
pnlArea.Controls.Add(new LiteralControl("<div class='box-tools pull-right'>"));
pnlArea.Controls.Add(new LiteralControl("</div>"));
pnlArea.Controls.Add(new LiteralControl("</div>"));
pnlArea.Controls.Add(new LiteralControl("<div class='box-body'>"));
//Placeholder to put future hazards into
PlaceHolder phHazard = new PlaceHolder();
phHazard.ID = "phHazard" + areaCount;
pnlArea.Controls.Add(phHazard);
//Create hazard button
pnlArea.Controls.Add(new LiteralControl("<br>"));
Button btnCreateHazard = new Button();
//btnCreateHazard.Click += btnCreateHazard_Click;
btnCreateHazard.ID = "btnCreateHazard" + areaCount;
btnCreateHazard.Text = "Create Hazard";
btnCreateHazard.CssClass = "form-control";
//Pass along the current area count, allowing the onclick to pick it up, pass it to
//the create hazard method. That method will then take the argument and search for a place
//holder with matching ID and assign the controls to that placeholder.
btnCreateHazard.Command += btnCreateHazard_Click;
btnCreateHazard.CommandArgument = areaCount.ToString();
pnlArea.Controls.Add(btnCreateHazard);
pnlArea.Controls.Add(new LiteralControl("</div>"));
pnlArea.Controls.Add(new LiteralControl("</div>"));
}
private void createHazard (int hazardCount, int placeholderID)
{
//The starting of the HTML rows, etc
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("<div class='row'>"));
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("<div class='col-lg-3'>"));
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("Hazard: "));
//Create the Hazard Dropdown
DropDownList ddHazard = new DropDownList();
ddHazard.ID = "ddHazard" + hazardCount;
FindControl("phHazard" + placeholderID).Controls.Add(ddHazard);
//HTML ending the first column, starting second
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("</div>"));
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("<div class='col-lg-3'>"));
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("Hazard Description: "));
//Create the hazard description textbox
TextBox txtHazardDesc = new TextBox();
txtHazardDesc.ID = "txtHazardDesc" + hazardCount;
FindControl("phHazard" + placeholderID).Controls.Add(txtHazardDesc);
//HTML ending second column, starting third
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("</div>"));
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("<div class='col-lg-3'>"));
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("Corrective Action Due Date: "));
//Create the due date textbox
TextBox txtDueDate = new TextBox();
txtDueDate.ID = "txtDueDate" + hazardCount;
FindControl("phHazard" + placeholderID).Controls.Add(txtDueDate);
//HTML ending the third column, starting fourth
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("</div>"));
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("<div class='col-lg-3'>"));
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("Corrective Action Description: "));
//Create the corrective action description text box
TextBox txtActionDesc = new TextBox();
txtActionDesc.ID = "txtActionDesc" + hazardCount;
FindControl("phHazard" + placeholderID).Controls.Add(txtActionDesc);
//End the row
FindControl("phHazard" + placeholderID).Controls.Add(new LiteralControl("<br>"));
}
}
}
这是向页面连续添加按钮的示例。 每个新创建的按钮也可以向页面添加另一个按钮。 可以与所有类型的控件一起使用。 另外,您还必须在每个PostBack上重新创建动态控件,否则它们及其内容将丢失。
protected void Page_Load(object sender, EventArgs e)
{
//set the initial number of buttons
int buttonCount = 1;
//check if the viewstate with the buttoncount already exists (= postback)
if (ViewState["buttonCount"] != null)
{
//convert the viewstate back to an integer
buttonCount = Convert.ToInt32(ViewState["buttonCount"]);
}
else
{
ViewState["buttonCount"] = buttonCount;
}
//create the required number of buttons
for (int i = 1; i <= buttonCount; i++)
{
createButton(i);
}
}
private void createButton(int cnt)
{
//create a new button control
Button button = new Button();
button.Text = "Add another Button (" + cnt + ")";
//add the correct method to the button
button.Click += DynamicButton_Click;
//another control, in this case a literal
Literal literal = new Literal();
literal.Text = "<br>";
//add the button and literal to the placeholder
PlaceHolder1.Controls.Add(button);
PlaceHolder1.Controls.Add(literal);
}
protected void DynamicButton_Click(object sender, EventArgs e)
{
//get the current number of buttons
int buttonCount = Convert.ToInt32(ViewState["buttonCount"]) + 1;
//create another button
createButton(buttonCount);
//set the new button count into the viewstate
ViewState["buttonCount"] = buttonCount;
}
更新
您还可以将Command
委派给按钮而不是Click
,并可以将变量和按钮一起作为CommandArgument
。 您将不得不稍微更改按钮的创建。
//add the correct method to the button
button.Command += DynamicButton_Command;
//now you can also add an argument to the button
button.CommandArgument = "Create Hazard";
您还需要其他方法来处理按钮单击。
protected void DynamicButton_Command(object sender, CommandEventArgs e)
{
//get the current number of buttons
int buttonCount = Convert.ToInt32(ViewState["buttonCount"]) + 1;
//create another button
createButton(buttonCount);
//set the new button count into the viewstate
ViewState["buttonCount"] = buttonCount;
//get the commandargument from the button
string buttonArgument = e.CommandArgument.ToString();
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.