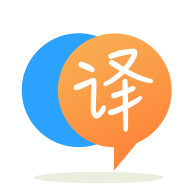
[英]Material-components-web : How to increase width of a Tempory Drawer?
[英]Using “material-components-web” within “react-boilerplate” code
在对GitHub上的现有样板代码进行了一些研究之后,我决定使用react-boilerplate来开始我的React应用程序。 我打算在我的网站上添加Material样式,并且由于现在不推荐使用react-mdl ,所以我打算在我的项目中使用material-components-web依赖项。
这是我的第一个React应用程序,我想知道一种清除默认样式并为项目上的material-components-web添加依赖项的干净方法。
非常感谢您的帮助。 谢谢。
编辑:更新的答案(v0.22.0)
对于设置CSS,您仍然可以使用普通的CSS:
<link rel="stylesheet" href="LINK_TO/material-components-web.css">
但是我建议您使用scss,这样您就可以覆盖不同的值(例如主题颜色)并按所需方式自定义所有内容。 例:
$mdc-theme-primary: #404040;
$mdc-theme-accent: #a349a3;
$mdc-theme-background: #fff;
@import "@material/ripple/mdc-ripple";
@import "@material/typography/mdc-typography";
@import "@material/theme/mdc-theme";
@import "@material/button/mdc-button";
为了使用需要使用javascript逻辑的组件,我通常为此组件编写react.js包装器。 这是一个接受/拒绝对话框组件的示例:
import React from 'react';
import { MDCDialog } from '@material/dialog/dist/mdc.dialog';
class AcceptDialog extends React.Component {
constructor(props) {
super(props);
this.passThroughInfo;
}
componentDidMount() {
this.dialog = new MDCDialog(this.refs.dialog);
// provide control to parent component to open the dialog from there
this.props.provideCtrl({
show: (passThroughInfo) => {
this.passThroughInfo = passThroughInfo;
this.dialog.show();
},
close: () => {
this.passThroughInfo = undefined;
this.dialog.close();
}
});
this.dialog.listen('MDCDialog:accept', () => {
this.props.acceptCb(this.passThroughInfo);
})
this.dialog.listen('MDCDialog:cancel', () => {
this.props.declineCb(this.passThroughInfo);
})
}
componentWillUnmount() {
this.props.provideCtrl(null);
}
defaultProps = {
className: "",
id: "",
header: "",
text: "",
acceptCb: function () { },
declineCb: function () { }
}
render() {
let className = "mdc-dialog accept_dialog" + this.props.className;
return (
<aside className={className} role="alertdialog" ref="dialog" id={this.props.id}>
<div className="mdc-dialog__surface">
{this.props.header !== "" &&
<header className="mdc-dialog__header">
<h2 className="mdc-dialog__header__title">
{this.props.header}
</h2>
</header>
}
<section className="mdc-dialog__body">
{this.props.section}
</section>
<footer className="mdc-dialog__footer">
<button type="button" className="mdc-button mdc-dialog__footer__button mdc-dialog__footer__button--cancel">Decline</button>
<button type="button" className="mdc-button mdc-dialog__footer__button mdc-dialog__footer__button--accept">Accept</button>
</footer>
</div>
<div className="mdc-dialog__backdrop"></div>
</aside>
);
}
}
export default AcceptDialog
然后像这样在其他组件中使用:
// How to open the accept dialog
this.accept_dialog.show(data);
// The callback called on accept
callback(data){...}
<AcceptDialog
acceptCb={this.callback}
provideCtrl={ctrl => this.accept_dialog = ctrl}>
</AcceptDialog>
____________OLD答案_________________
1)添加CSS
那真的很容易。 您可以将其添加到index.html文件中:
<link rel="stylesheet" href="LINK_TO/material-components-web.css">
如果您使用的是SASS,则还可以访问sass文件(必须安装@material npm模块:npm install --save @material)。 然后,您需要node-sass,并在webpack配置中包含@material文件夹的路径。 我还没有尝试过,但是从理论上讲,这应该可行。
2)添加JavaScript
同样,您有更多的可能性。 这是我所做的。 您可以像这样导入mdc
class Foo extends Component(){
componentDidMount(){
// either this like that
mdc.textfield.MDCTextfield.attachTo(this.refs.textfield);
// or like that
const MDCTextfield = mdc.textfield.MDCTextfield;
const textfield = new MDCTextfield(this.refs.textfield);
}
render() {
return(
<form className="form-group" onSubmit={this.submitForm}>
<div className="mdc-textfield" ref="textfield">
<input type="text" id="my-textfield" className="mdc-textfield__input"/>
<label className="mdc-textfield__label" htmlFor="my-textfield">Hint text</label>
</div>
</form>
);
}
}
现在,您可以为“输入”字段创建一个组件(包装)。 如果您不想这样做,还可以通过添加以下内容自动初始化所有组件:
// app.component.js
import * as mdc from 'material-components-web/dist/material-components-web';
class App extends React.Component {
componentDidMount(){
mdc.autoInit();
}
render(){
return(
<div className="my-app">
{this.props.children}
</div>
);
}
}
并将其添加到您的文本字段:
<div className="mdc-textfield" data-mdc-auto-init="MDCTextfield">
现在另一种可能性是这样导入:
import {MDCCheckbox} from '@material/checkbox';
在这里,您需要告诉webpack配置文件中的bable-loader也要包含node_modules / @ material文件夹。 其他一切基本保持不变。
修改@Jodo答案有点,这对我有用:
import React from 'react'
import {observer} from 'mobx-react'
import * as mdc from 'material-components-web/dist/material-components-web'
@observer
export class RegisterForm extends React.Component{
/**
* Notice I'm calling mdc.autoInit() on the "RegisterForm" component NOT on any parent component
* Actually, when I call mdc.autoInit() on any parent component, it won't work
*/
componentDidMount=()=>{mdc.autoInit()}
setMeta=(e)=>{
this.props.store.storeReg.setMeta(e.target.name,e.target.value)
}
preview=()=>{
this.props.store.storeReg.preview()
}
render=()=>{
return(
<form onChange={this.setMeta.bind(this)}>
<div class="mdc-form-field">
<div class="mdc-textfield" data-mdc-auto-init="MDCTextfield">
<input class="mdc-textfield__input" type="text" required name="email" value={this.props.store.storeReg.meta.get('email')}/>
<label class="mdc-textfield__label" for="email">Email</label>
</div>
</div>
<div class="mdc-form-field">
<div class="mdc-textfield" data-mdc-auto-init="MDCTextfield">
<input class="mdc-textfield__input" type="text" required name="first_name" value={this.props.store.storeReg.meta.get('first_name')}/
<label class="mdc-textfield__label" for="first_name">First Name</label>
</div>
</div>
<div class="mdc-form-field">
<div class="mdc-textfield" data-mdc-auto-init="MDCTextfield">
<input class="mdc-textfield__input" type="text" required name="last_name" value={this.props.store.storeReg.meta.get('last_name')}/>
<label class="mdc-textfield__label" for="last_name">Last Name</label>
</div>
</div>
<div class="group">
<button class="mdc-button mdc-button--raised mdc-button--primary mdc-ripple-surface" data-mdc-auto-init="MDCRipple" type="button" onClic
</div>
</form>
)
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.