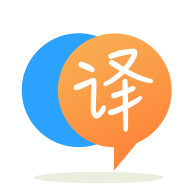
[英]Getting pl/sql array of struct from stored procedure using JdbcSimpleCall
[英]Read an ARRAY from a STRUCT returned by a stored procedure
数据库中是三种Oracle自定义类型(简化)如下:
create or replace TYPE T_ENCLOSURE AS OBJECT(
ENCLOSURE_ID NUMBER(32,0),
ENCLOSURE_NAME VARCHAR2(255 BYTE),
ANIMALS T_ARRAY_ANIMALS,
MEMBER FUNCTION CHECK_IF_RED RETURN BOOLEAN
);
create or replace TYPE T_ARRAY_ANIMALS is TABLE OF T_ANIMAL;
create or replace TYPE T_ANIMAL AS OBJECT(
ANIMAL_ID NUMBER(32,0),
NUMBER_OF_HAIRS NUMBER(32,0)
);
和一个构建对象树的函数
FUNCTION GET_ENCLOSURE ( f_enclosure_id zoo_schema.ENCLOSURE_TABLE.ENCLOSURE_ID%TYPE ) RETURN T_ENCLOSURE
AS
v_ENC T_ENCLOSURE;
v_idx pls_integer;
BEGIN
v_ENC := T_ENCLOSURE(
f_enclosure_id,
NULL,
T_ARRAY_ANIMALS(T_ANIMAL(NULL,NULL))
);
SELECT ENCLOSURE_NAME
INTO v_ENC.ENCLOSURE_NAME
FROM ENCLOSURE_TABLE WHERE ENCLOSURE_ID = f_ENCLOSURE_ID;
SELECT
CAST(MULTISET(
SELECT ANIMAL_ID, NUMBER_OF_HAIRS
FROM ANIMAL_TABLE
WHERE ENCLOSURE_ID = f_ENCLOSURE_ID
) AS T_ARRAY_ANIMALS
)
INTO v_ENC.ANIMALS
FROM dual;
RETURN v_ENC;
END;
现在我想调用GET_ENCLOSURE
函数并在我的 Java 代码中使用它的结果T_ENCLOSURE
对象。
// prepare the call
Connection connection = MyConnectionFactory.getConnection(SOME_CONNECTION_CONFIG);
CallableStatement stmt = connection.prepareCall("{? = call zoo_schema.zoo_utils.GET_ENCLOSURE( ? )}");
stmt.registerOutParameter(1, OracleTypes.STRUCT, "zoo_schema.T_ENCLOSURE");
stmt.setInt(2, 6); // fetch data for ENCLOSURE#6
// execute function
stmt.executeQuery();
// extract the result
Struct resultStruct = (Struct)stmt.getObject(1); // java.sql.Struct
我可以通过以下方式访问ID和NAME
Integer id = ((BigInteger)resultStruct.getAttributes()[0]).intValue(); // works for me
String name = (String)resultStruct.getAttributes()[1]); // works for me
但是,我似乎无法获得动物列表
resultStruct.getAttributes()[2].getClass().getCanonicalName(); // oracle.sql.ARRAY
ARRAY arrayAnimals = (ARRAY)jdbcStruct.getAttributes()[2];
arrayAnimals.getArray(); // throws a java.sql.SQLException("Internal Error: Unable to resolve name")
我在这里进行了一些试验和错误,包括
OracleConnection oracleConnection = connection.unwrap(OracleConnection.class);
STRUCT resultOracleStruct = (STRUCT) stmt.getObject(1); // oracle.sql.STRUCT
oracleConnection.createARRAY("zoo_schema.T_ARRAY_ANIMALS", resultOracleStruct.getAttributes()[2]) // throws an SQLException("Fail to convert to internal representation: oracle.sql.ARRAY@8de7cfc4")
但也没有运气。
如何将动物列表放入List<TAnimal>
?
只要特定于 Oracle 的解决方案就足够了,关键在于 DTO。 他们都必须实现ORAData
和ORADataFactory
public class TAnimal implements ORAData, ORADataFactory {
Integer animal_id, number_of_hairs;
public TAnimal() { }
// [ Getter and Setter omitted here ]
@Override
public Datum toDatum(Connection connection) throws SQLException {
OracleConnection oracleConnection = connection.unwrap(OracleConnection.class);
StructDescriptor structDescriptor = StructDescriptor.createDescriptor("zoo_schema.T_ANIMAL", oracleConnection);
Object[] attributes = {
this.animal_id,
this.number_of_hairs
};
return new STRUCT(structDescriptor, oracleConnection, attributes);
}
@Override
public TAnimal create(Datum datum, int sqlTypeCode) throws SQLException {
if (datum == null) {
return null;
}
Datum[] attributes = ((STRUCT) datum).getOracleAttributes();
TAnimal result = new TAnimal();
result.animal_id = asInteger(attributes[0]); // see TEnclosure#asInteger(Datum)
result.number_of_hairs = asInteger(attributes[1]); // see TEnclosure#asInteger(Datum)
return result;
}
}
和
public class TEnclosure implements ORAData, ORADataFactory {
Integer enclosureId;
String enclosureName;
List<Animal> animals;
public TEnclosure() {
this.animals = new ArrayList<>();
}
// [ Getter and Setter omitted here ]
@Override
public Datum toDatum(Connection connection) throws SQLException {
OracleConnection oracleConnection = connection.unwrap(OracleConnection.class);
StructDescriptor structDescriptor = StructDescriptor.createDescriptor("zoo_schema.T_ENCLOSURE", oracleConnection);
Object[] attributes = {
this.enclosureId,
this.enclosureName,
null // TODO: solve this; however, retrieving data works without this
};
return new STRUCT(structDescriptor, oracleConnection, attributes);
}
@Override
public TEnclosure create(Datum datum, int sqlTypeCode) throws SQLException {
if (datum == null) {
return null;
}
Datum[] attributes = ((STRUCT) datum).getOracleAttributes();
TEnclosure result = new TEnclosure();
result.enclosureId = asInteger(attributes[0]);
result.enclosureName = asString(attributes[1]);
result.animals = asListOfAnimals(attributes[2]);
return result;
}
// Utility methods
Integer asInteger(Datum datum) throws SQLException {
if (datum == null)
return null;
else
return ((NUMBER) datum).intValue(); // oracle.sql.NUMBER
}
String asString(Datum datum) throws SQLException {
if (datum = null)
return null;
else
return ((CHAR) datum).getString(); // oracle.sql.CHAR
}
List<TAnimal> asListOfAnimals(Datum datum) throws SQLException {
if (datum == null)
return null;
else {
TAnimal factory = new TAnimal();
List<TAnimal> result = new ArrayList<>();
ARRAY array = (ARRAY) datum; // oracle.sql.ARRAY
Datum[] elements = array.getOracleArray();
for (int i = 0; i < elements.length; i++) {
result.add(factory.create(elements[i], 0));
}
return result;
}
}
}
然后获取数据的工作方式如下:
TEnclosure factory = new TEnclosure();
Connection connection = null;
OracleConnection oracleConnection = null;
OracleCallableStatement oracleCallableStatement = null;
try {
connection = MyConnectionFactory.getConnection(SOME_CONNECTION_CONFIG);
oracleConnection = connection.unwrap(OracleConnection.class);
oracleCallableStatement = (OracleCallableStatement) oracleConnection.prepareCall("{? = call zoo_schema.zoo_utils.GET_ENCLOSURE( ? )}");
oracleCallableStatement.registerOutParameter(1, OracleTypes.STRUCT, "zoo_schema.T_ENCLOSURE");
oracleCallableStatement.setInt(2, 6); // fetch data for ENCLOSURE#6
// Execute query
oracleCallableStatement.executeQuery();
// Check result
Object oraData = oracleCallableStatement.getORAData(1, factory);
LOGGER.info("oraData is a {}", oraData.getClass().getName()); // acme.zoo.TEnclosure
} finally {
ResourceUtils.closeQuietly(oracleCallableStatement);
ResourceUtils.closeQuietly(oracleConnection);
ResourceUtils.closeQuietly(connection); // probably not necessary...
}
创建实现java.sql.SQLData
对象。 在这种情况下,创建TEnclosure
和TAnimal
类,它们都实现SQLData
。
仅供参考,在较新的 Oracle JDBC 版本中,不推荐使用诸如oracle.sql.ARRAY之类的类型以支持java.sql
类型。 尽管我不确定如何仅使用java.sql
API 编写数组(如下所述)。
当您实现readSQL()
您会按顺序读取字段。 您使用sqlInput.readArray()
获得java.sql.Array
。 所以TEnclosure.readSQL()
看起来像这样。
@Override
public void readSQL(SQLInput sqlInput, String s) throws SQLException {
id = sqlInput.readBigDecimal();
name = sqlInput.readString();
Array animals = sqlInput.readArray();
// what to do here...
}
注意: readInt()
也存在,但Oracle JDBC 似乎总是为NUMBER
提供BigDecimal
您会注意到某些 API(例如java.sql.Array
具有采用类型映射Map<String, Class<?>>
这是 Oracle 类型名称到实现SQLData
的相应 Java 类的映射( ORAData
也可以工作? )。
如果您只是调用Array.getArray()
,您将获得Struct
对象,除非 JDBC 驱动程序通过Connection.setTypeMap(typeMap)
知道您的类型映射。 但是,在连接上设置 typeMap 对我不起作用,所以我使用getArray(typeMap)
在某处创建您的Map<String, Class<?>> typeMap
并为您的类型添加条目:
typeMap.put("T_ENCLOSURE", TEnclosure.class);
typeMap.put("T_ANIMAL", TAnimal.class);
在SQLData.readSQL()
实现中,调用sqlInput.readArray().getArray(typeMap)
,它返回Object[]
,其中Object
条目或类型为TAnimal
。
当然,转换为List<TAnimal>
的代码会变得乏味,所以只需使用此实用程序函数并根据您的需要调整它,就 null 与空列表策略而言:
/**
* Constructs a list from the given SQL Array
* Note: this needs to be static because it's called from SQLData classes.
*
* @param <T> SQLData implementing class
* @param array Array containing objects of type T
* @param typeClass Class reference used to cast T type
* @return List<T> (empty if array=null)
* @throws SQLException
*/
public static <T> List<T> listFromArray(Array array, Class<T> typeClass) throws SQLException {
if (array == null) {
return Collections.emptyList();
}
// Java does not allow casting Object[] to T[]
final Object[] objectArray = (Object[]) array.getArray(getTypeMap());
List<T> list = new ArrayList<>(objectArray.length);
for (Object o : objectArray) {
list.add(typeClass.cast(o));
}
return list;
}
写数组
弄清楚如何编写数组令人沮丧,Oracle API 需要一个 Connection 来创建一个数组,但是在writeSQL(SQLOutput sqlOutput)
的上下文中没有明显的 Connection 。 幸运的是, 这个博客有一个技巧/技巧来获取我在这里使用的OracleConnection
。
当您使用createOracleArray()
创建数组时,您为类型名称指定列表类型( T_ARRAY_ANIMALS
),而不是单一对象类型。
这是用于编写数组的通用函数。 在您的情况下, listType
将是"T_ARRAY_ANIMALS"
,您将传入List<TAnimal>
/**
* Write the list out as an Array
*
* @param sqlOutput SQLOutput to write array to
* @param listType array type name (table of type)
* @param list List of objects to write as an array
* @param <T> Class implementing SQLData that corresponds to the type listType is a list of.
* @throws SQLException
* @throws ClassCastException if SQLOutput is not an OracleSQLOutput
*/
public static <T> void writeArrayFromList(SQLOutput sqlOutput, String listType, @Nullable List<T> list) throws SQLException {
final OracleSQLOutput out = (OracleSQLOutput) sqlOutput;
OracleConnection conn = (OracleConnection) out.getSTRUCT().getJavaSqlConnection();
conn.setTypeMap(getTypeMap()); // not needed?
if (list == null) {
list = Collections.emptyList();
}
final Array array = conn.createOracleArray(listType, list.toArray());
out.writeArray(array);
}
笔记:
setTypeMap
是必需的,但现在当我删除那行时,我的代码仍然有效,所以我不确定是否有必要。有关 Oracle 类型的提示
SCHEMA.TYPE_NAME
。grant execute
权限。getArray()
在尝试查找类型元数据时将抛出异常。春天
对于使用Spring 的开发人员,您可能需要查看Spring Data JDBC Extensions ,它提供了SqlArrayValue
和SqlReturnArray
,它们对于为将数组作为参数或返回数组的过程创建SimpleJdbcCall
非常有用。
第7.2.1章使用 SqlArrayValue 为 IN 参数设置 ARRAY 值解释了如何使用数组参数调用过程。
您可能会像这样转换为java.sql.Array
:
Object array = ( (Array) resultOracleStruct.getAttributes()[2]) ).getArray();
我只是分享对我有用的逻辑。 您可以尝试将 ARRAY 响应从 PL/SQL 检索到 Java。
CallableStatement callstmt = jdbcConnection.prepareCall("{call PROCEDURE_NAME(?, ?)}");
callstmt.setArray(1, array);
callstmt.registerOutParameter(2,Types.ARRAY, <ARRAY_NAME_DECLARED_IN_PL/SQL>);
// Do all execute operations
Array arr = callstmt.getArray(1);
if (arr != null) {
Object[] data = (Object[]) arr.getArray();
for (Object a : data) {
OracleStruct empstruct = (OracleStruct) a;
Object[] objarr = empstruct.getAttributes();
<Your_Pojo_class> r = new <Your_Pojo_class>(objarr[0].toString(), objarr[1].toString());
System.out.println("Response-> : "+ r.toString());
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.