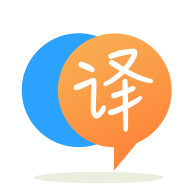
[英]Nested struct in templated class with std::map::const_iterator?
[英]const_iterator: nested class or friend class?
我在为这个SparseGraph
类制作这个const_iterator时遇到了一些严重的问题
SparseGraph类:
对于本文的意图和目的,可以将SparseGraph
视为边的列表(或向量矢量)列表(这通常称为邻接列表)。 然后const_iterator
遍历列表列表中的每个边。
考虑:
template <typename NodeType, typename EdgeType>
class SparseGraph
{
public:
//...
using EdgeList = std::vector<EdgeType>;
using AdjacencyList = std::vector<EdgeList>;
//...
private:
//...
AdjacencyList m_adj_list;
//...
}
const_iterator的初始尝试(嵌套类)
//----SparseGraph
template <typename NodeType, typename EdgeType>
class SparseGraph
{
public:
class const_iterator; //nested
//...
}
//----SparseGraph::const_iterator
template <typename NodeType, typename EdgeType>
class SparseGraph<NodeType, EdgeType>::const_iterator
{
private:
using NodeIterator = typename SparseGraph<NodeType, EdgeType>::AdjacencyList::const_iterator;
using EdgeIterator = typename SparseGraph<NodeType, EdgeType>::EdgeList::const_iterator;
//this is used to iterate through each edge list corresponding to each node
NodeIterator m_node_it;
//this is used to iterate through each edge in each edge list.
EdgeIterator m_edge_it;
public:
const_iterator(const NodeIterator& node_it, const EdgeIterator& edge_it)
: m_node_it{node_it}, m_edge_it{edge_it} {}
const_iterator& operator++(int)
{
//TODO
return *this;
}
//...
正如人们可能注意到的那样, operator++()
给了我“非静态引用”错误:
错误:无效使用非静态数据成员'SparseGraph :: m_adj_list'
从:
//...
if(m_node_it == m_adj_list.end()) { //<--here,
//loop back around to the beginning
m_node_it = m_adj_list.begin(); //<--here,
}
m_edge_it = m_node_it.begin();//<--and here.
//...
const_iterator的初始尝试(朋友类)
现在我们有以下几点:
//----SparseGraph
template <typename NodeType, typename EdgeType>
class SparseGraph
{
public:
friend class const_iterator; //friend
//as before...
}
//----SparseGraph::const_iterator-------------------------------------------------------------------------------------------------------------
template <typename NodeType, typename EdgeType>
class const_iterator
{
private:
using NodeIterator = typename SparseGraph<NodeType, EdgeType>::AdjacencyList::const_iterator;
using EdgeIterator = typename SparseGraph<NodeType, EdgeType>::EdgeList::const_iterator;
const SparseGraph<NodeType, EdgeType>& m_graph;
NodeIterator m_node_it;
EdgeIterator m_edge_it;
public:
const_iterator(const SparseGraph<NodeType, EdgeType>& graph,
const NodeIterator& node_it,
const EdgeIterator& edge_it)
: m_graph{graph} {}
//...
这给了我错误
错误:'const_iterator'没有命名类型
对于
template <typename NodeType, typename EdgeType>
class SparseGraph
{
//...
public:
//...
const_iterator begin() const;
//...
}
和
错误:在'SparseGraph :: const_iterator'之前需要'typename',因为'SparseGraph'是一个依赖范围
对于
template <typename NodeType, typename EdgeType> typename
SparseGraph<NodeType, EdgeType>::const_iterator SparseGraph<NodeType, EdgeType>::begin() const
{
return const_iterator(*this, m_adj_list.begin(), m_adj_list.begin() >begin());
然后变为
错误:没有'typename SparseGraph :: const_iterator SparseGraph :: begin()const'在'SparseGraph'类中声明的成员函数
当我添加该类型名称。 我怀疑如果我解决了第一个错误就会解决这个问题,但是我不知道那里有什么问题。 此外,朋友类现在正在污染包含它的任何翻译单元的范围。
我目前的守则
#ifndef SPARSE_GRAPH_H
#define SPARSE_GRAPH_H
#include <iostream>
#include <vector>
enum
{
invalid_node_index=-1
};
template <typename NodeType, typename EdgeType>
class SparseGraph
{
public:
class const_iterator;
using Node = NodeType;
using Edge = EdgeType;
using NodeList = std::vector<Node>;
using EdgeList = std::vector<Edge>;
using AdjacencyList = std::vector<EdgeList>;
private:
//...
//Similarly to the NodeList, each node index is analogous
//to its index in this list. Each element of this list contains
//a sub-list of edges associated with a particular node.
//For example, the list at index 3 will contain all edges associated
//with the node with an index of 3. This is so we can have an O(1) lookup time.
AdjacencyList m_adj_list;
//...
public:
SparseGraph() {}
//...
const_iterator begin() const;
const_iterator end() const;
//...
};
//----SparseGraph::const_iterator-------------------------------------------
template <typename NodeType, typename EdgeType>
class const_iterator
{
private:
using NodeIterator = typename SparseGraph<NodeType, EdgeType>::AdjacencyList::const_iterator;
using EdgeIterator = typename SparseGraph<NodeType, EdgeType>::EdgeList::const_iterator;
NodeIterator m_node_it;
EdgeIterator m_edge_it;
const NodeIterator m_begin;
const NodeIterator m_end;
public:
const_iterator(NodeIterator node_it,
EdgeIterator edge_it,
NodeIterator begin,
NodeIterator end)
: m_node_it{node_it}, m_edge_it{edge_it}, m_begin{begin}, m_end{end} {}
const_iterator& operator++(int)
{
//are we at the end of a valid edge list?
if(m_node_it != end && m_edge_it == m_node_it->end()) {
//move to the next non-empty edge list or to the end of the adjacency list
while(m_node_it != end && m_node_it->empty()) {
m_node_it++;
}
if(m_node_it != end) {
m_edge_it = m_node_it->begin();
}
}
else {
m_edge_it++;
}
return *this;
}
const_iterator& operator--(int)
{
//TODO
return *this;
}
const typename SparseGraph<NodeType, EdgeType>::Edge& operator*() const
{
return *m_edge_it;
}
const typename SparseGraph<NodeType, EdgeType>::Edge* operator->() const
{
return &(*m_edge_it);
}
bool operator==(const const_iterator& other) const
{
return m_node_it == other.m_node_it &&
m_edge_it == other.m_edge_it;
}
bool operator!=(const const_iterator& other) const
{
return !(*this == other);
}
};
//----SPARSE_GRAPH----------------------------------------------------------
//----PUBLIC FUNCTIONS------------------------------------------------------
//----begin()
template <typename NodeType, typename EdgeType>
typename SparseGraph<NodeType, EdgeType>::const_iterator SparseGraph<NodeType, EdgeType>::begin() const
{
return const_iterator(m_adj_list.begin(),
m_adj_list.begin()->begin(),
m_adj_list.begin(),
m_adj_list.back().end());
}
//----end()
template <typename NodeType, typename EdgeType>
typename SparseGraph<NodeType, EdgeType>::const_iterator SparseGraph<NodeType, EdgeType>::end() const
{
return const_iterator(m_adj_list.end(),
m_adj_list.end()->end(),
m_adj_list.begin(),
m_adj_list.back().end());
}
#endif // SPARSE_GRAPH_H
我的问题是 ,我该怎么做呢? 我应该使用嵌套的const_iterator类还是朋友的const_iterator类,或者,哪种方式是标准的? 我应该如何实现const_iterator来避免这些问题? 总的来说,我正在寻找一个双向const_iterator,它将遍历AdjacencyList(列表列表)中的每个Edge,而不允许更改所述AdjacencyList。
我查看了本站点上有关“const_pointer实现”的其他帖子,甚至还提到了Stroustrup的“C ++编程语言”,但到目前为止还没有什么能让我深入了解如何正确实现类似于此的const_iterator。
我对如何正确地解决这个问题感到不自在,所以对任何事情的任何帮助(可能与手头的主要问题无关)都会非常感激。
通过将友元类转换为嵌套类很容易编译(没有充分的理由不使用嵌套类)。 但是,您的代码不会实例化任何模板。 当你尝试时,真正的问题就开始了。
这是您需要应用的差异:
friend class const_iterator; (ca. line 26) ===>
class const_iterator;
class const_iterator (ca. line 98) ===>
template <typename NodeType, typename EdgeType>
class SparseGraph<NodeType, EdgeType>::const_iterator
const_iterator SparseGraph<NodeType, EdgeType>::begin() const (ca. line 301) ===>
typename SparseGraph<NodeType, EdgeType>::const_iterator
SparseGraph<NodeType, EdgeType>::begin() const
const_iterator SparseGraph<NodeType, EdgeType>::end() const (ca. line 308) ===>
typename SparseGraph<NodeType, EdgeType>::const_iterator
SparseGraph<NodeType, EdgeType>::end() const
但是,您的代码不会实例化任何模板。 当您尝试创建const_iterator并将其递增时,会出现真正的问题。 也就是说,你的边缘列表,顶点列表和邻接列表类型及它们的迭代器,const迭代器和const_iterator都混合在一个大沙拉碗里。 他们需要仔细分离和清理。
const_iterator类也没有copy / move ctor,可能还有其他一些小问题。
你原来的
错误:无效使用非静态数据成员'SparseGraph :: m_adj_list'
表示范围内没有SparseGraph
来获取m_adj_list.begin()
。 你不需要调用它,只需在你的const_iterator中存储SparseGraph :: m_adj_list的开头和结尾
template <typename NodeType, typename EdgeType>
class SparseGraph<NodeType, EdgeType>::const_iterator
{
private:
using NodeIterator = typename SparseGraph<NodeType, EdgeType>::AdjacencyList::const_iterator;
using EdgeIterator = typename SparseGraph<NodeType, EdgeType>::AdjacencyList::const_iterator;
const_iterator(NodeIterator node_it, EdgeIterator edge_it, NodeIterator adjacent_begin, NodeIterator adjacent_end) : m_node_it{node_it}, m_edge_it{edge_it}, m_adjacent_begin{adjacent_begin}, m_adjacent_end{adjacent_end} {}
const NodeIterator m_adjacent_begin;
const NodeIterator m_adjacent_end;
NodeIterator m_node_it;
EdgeIterator m_edge_it;
const_iterator& operator++(int)
{
//are we at the end of this edge list?
if(m_edge_it == m_node_it->end()) {
//move to the next node's edge list.
m_node_it++;
//are we past the last node's edge list?
if(m_node_it == m_adjacent_end) {
//loop back around to the beginning
m_node_it = m_adjacent_begin;
}
m_edge_it = m_node_it.begin();
}
else {
m_edge_it++;
}
return *this;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.