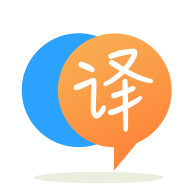
[英]Passing basic authentication details in spring security using http headers in java
[英]Spring Security using HTTP headers
我正在尝试为我的 Spring Boot 应用程序添加安全性。 我当前的应用程序正在使用 REST 控制器,每次收到GET
或POST
请求时,我都会读取 HTTP 标头以检索用户和密码,以便根据我存储的所有用户的属性文件来验证它们。 我想将其更改为使用 Spring Security,这就是我到目前为止所得到的:
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Bean
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/index.html").permitAll()
.antMatchers("/swagger-ui.html").hasRole("ADMIN")
.anyRequest().authenticated();
}
@Bean
public UserDetailsService userDetailsService() {
InMemoryUserDetailsManager manager = new InMemoryUserDetailsManager();
manager.createUser(User.withUsername("admin").password("password").roles("ADMIN").build());
}
}
如何告诉configure
方法是从标题中检索用户凭据,而不是从登录表单中检索?
最少的代码添加是定义一个过滤器并将其添加到安全配置中,smth like
XHeaderAuthenticationFilter.java
@Component
public class XHeaderAuthenticationFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(HttpServletRequest request,
HttpServletResponse response, FilterChain filterChain)
throws ServletException, IOException {
String xAuth = request.getHeader("X-Authorization");
User user = findByToken(xAuth);
if (user == null) {
response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Token invalid");
} else {
final UsernamePasswordAuthenticationToken authentication =
new UsernamePasswordAuthenticationToken(user, null, user.getAuthorities());
SecurityContextHolder.getContext().setAuthentication(authentication);
filterChain.doFilter(request, response);
}
}
//need to implement db user validation...
private User findByToken(String token) {
if (!token.equals("1234"))
return null;
final User user = new User(
"username",
"password",
true,
true,
true,
true,
Collections.singletonList(new SimpleGrantedAuthority("ROLE_USER")));
return user;
}
}
安全配置.java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(final HttpSecurity http) throws Exception {
http.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.csrf().disable()
.authorizeRequests().anyRequest().authenticated()
.and()
.exceptionHandling()
.authenticationEntryPoint(new HttpStatusEntryPoint(HttpStatus.UNAUTHORIZED))
.and()
.addFilterBefore(new XHeaderAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
}
另一种方法是使用spring的AOP
在进入带注释的控制器方法之前定义一些要执行的逻辑的注释
您应该避免使用默认的org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter
因为它从您的请求参数中获取客户端提供的用户名和密码,并且您确实需要从标头中获取它们。
因此,您应该编写一个自定义AuthenticationFilter
扩展引用的UsernamePasswordAuthenticationFilter
以更改其行为以满足您的要求:
public class HeaderUsernamePasswordAuthenticationFilter extends UsernamePasswordAuthenticationFilter {
public HeaderUsernamePasswordAuthenticationFilter() {
super();
this.setFilterProcessesUrl("/**");
this.setPostOnly(false);
}
/* (non-Javadoc)
* @see org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter#obtainPassword(javax.servlet.http.HttpServletRequest)
*/
@Override
protected String obtainPassword(HttpServletRequest request) {
return request.getHeader(this.getPasswordParameter());
}
/* (non-Javadoc)
* @see org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter#obtainUsername(javax.servlet.http.HttpServletRequest)
*/
@Override
protected String obtainUsername(HttpServletRequest request) {
return request.getHeader(this.getPasswordParameter());
}
}
这个过滤器示例扩展了org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter
监听每个请求并从 headers 而不是parameters
获取username
和password
。
然后您应该以这种方式更改配置,在UsernamePasswordAuthenticationFilter
位置设置您的过滤器:
@Override
protected void configure(HttpSecurity http) throws Exception {
http.addFilterAt(
new HeaderUsernamePasswordAuthenticationFilter(),
UsernamePasswordAuthenticationFilter.class)
.authorizeRequests()
.antMatchers("/index.html").permitAll()
.antMatchers("/swagger-ui.html").hasRole("ADMIN")
.anyRequest().authenticated();
}
记忆中的身份验证将服务于您的目的
@Configuration
@EnableWebMvc
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user1").password("password1").roles("USER")
.and()
.withUser("user2").password("password2").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests().anyRequest().fullyAuthenticated();
http.httpBasic();
}
}
在 Spring Boot 应用程序中,您可以将以下内容添加到 application.properties
security.user.name=user
security.user.password=password
它会做剩下的事情,比如从标题和验证中获取更多访问https://docs.spring.io/spring-boot/docs/current/reference/html/boot-features-security.html
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.