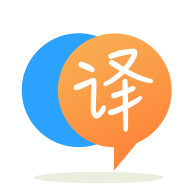
[英]ReactJS: How to change state property value in a component, when the state property value in another component is a certain value
[英]ReactJS: How to get state property of another component?
有一个主要组件,它使用菜单组件。 菜单组件正在使用状态属性来保存有关所选菜单项的信息。 但是现在我需要在主组件中获取选定的模块。 我怎么做?
class Main extends Component {
doSomething(module) {
console.log(module) // should get 'targetValue'
// I need to get the info, which module is selected.
// This info is stored as a state value in the `MainMenu` Component
// How do I get this information? I can't use the parameter `selectModule` as it is done here.
}
render() {
return (
<div>
<MainMenu />
<Button
onClick={ this.doSomething.bind(this, selectedModule) }
/>
</div>
)
}
}
在此组件中,将为( modules
阵列的)每个模块生成一个菜单。 通过单击一项,此模块将存储到module
状态变量中。
class MainMenu extends Component {
constructor(props) {
super(props)
this.state = {
module: 'initialValue'
}
}
selectModule(module) {
this.setState({ module })
}
render() {
return (
<Menu>
<Menu.Item onClick={ this.selectModule.bind(this, 'targetValue') } >
{ title }
</Menu.Item>
</Menu>
)
}
}
如果子组件将状态提升到父级,则不必做任何魔术,也不能检查内部状态。 孩子变得无国籍。
class Main extends Component {
state = {
module: 'initialValue'
}
setActiveModule = (module) => {
this.setState({ module })
}
render() {
return (
<MainMenu onChange={this.setActiveModule} />
)
}
}
class MainMenu extends Component {
onClick = (module) => () => {
this.props.onChange(module)
}
render() {
return (
<Menu>
<Menu.Item onClick={this.onClick(title)} >
{title}
</Menu.Item>
</Menu>
)
}
}
而是在维护MainMenu组件中的state
时,在父组件Main中维护,并在props
传递模块值,还将function
传递给MainMenu以从子MainMenu更新父组件Main的state
。
像这样写:
class Main extends Component {
constructor(props) {
super(props)
this.state = {
module: 'initialValue'
}
this.update = this.update.bind(this);
}
update(value){
this.setState({
module: value
});
}
doSomething(){
console.log(this.state.module);
}
render() {
return (
<div>
<MainMenu module={this.state.module} update={this.update}/>
<Button
onClick={ this.doSomething.bind(this) }
/>
</div>
)
}
}
class MainMenu extends Component {
selectModule(module) {
this.props.update(module);
}
render() {
console.log(this.props.module);
return (
<Menu>
<Menu.Item onClick={this.selectModule.bind(this, 'targetValue') } >
{ title }
</Menu.Item>
</Menu>
)
}
}
与react共享状态有时会有点困难。
反应哲学倾向于说我们必须从上到下管理状态。 这个想法是要修改您父母的状态,并将信息作为道具传递。 例如,让我们想象以下情形:
class Main extends React.Component {
contructor(props) {
super(props);
this.state = { currentMenuSelected: 'Home' };
}
onPageChange(newPage) {
this.setState({ currentMenuSelected: newPage });
}
render() {
return(
<div>
<AnotherComponent currentMenu={this.state.currentMenuSelected} />
<MenuWrapper onMenuPress={this.onPageChange} />
</div>
)
}
}
在我的例子中,我们告诉MenuWrapper
使用Main.onPageChange
改变页面时。 这样,我们现在可以使用道具将当前所选菜单传递给AnotherComponent
。
这是使用react管理状态共享的第一种方法,也是库提供的默认方法
如果要管理更复杂的内容,共享更多状态,则应查看助焊剂架构https://facebook.github.io/flux/docs/overview.html
以及最常见的flux实现: http : //redux.js.org/
将菜单状态存储在主要组件中,然后将状态更新程序传递给菜单。
这对于进入自上而下的状态非常有用https://facebook.github.io/react/docs/thinking-in-react.html
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.