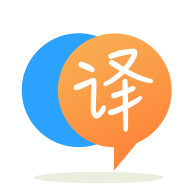
[英]WPF when I change the string to which binded Text of TextBlock, the text doesn't change
[英]Getting error when I try to change TextBlock text
我正在关注 Head First C# 3rd edition,在第一章中它要求我更改 TextBlock 的文本,但是当我尝试时出现以下错误:
InvalidCastException:无法将“Windows.UI.Text.TextDecorations”类型的对象转换为“System.Windows.TextDecorationCollection”类型。
这本书建议我将 Visual Studio Express 2012 用于 Windows 8,我正在遵循这些建议。
XAML 代码:
<common:LayoutAwarePage
x:Name="pageRoot"
x:Class="Save_The_Humans.MainPage"
DataContext="{Binding DefaultViewModel, RelativeSource={RelativeSource Self}}"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:Save_The_Humans"
xmlns:common="using:Save_The_Humans.Common"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Page.Resources>
<!-- TODO: Delete this line if the key AppName is declared in App.xaml -->
<x:String x:Key="AppName">Save The Humans</x:String>
</Page.Resources>
<!--
This grid acts as a root panel for the page that defines two rows:
* Row 0 contains the back button and page title
* Row 1 contains the rest of the page layout
-->
<Grid Style="{StaticResource LayoutRootStyle}">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="160"/>
<ColumnDefinition/>
<ColumnDefinition Width="160"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="140"/>
<RowDefinition/>
<RowDefinition Height="160"/>
</Grid.RowDefinitions>
<!-- Back button and page title -->
<Grid Grid.ColumnSpan="3">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Button x:Name="backButton" Click="GoBack" IsEnabled="{Binding Frame.CanGoBack, ElementName=pageRoot}" Style="{StaticResource BackButtonStyle}"/>
<TextBlock x:Name="pageTitle" Grid.Column="1" Text="{StaticResource AppName}" Style="{StaticResource PageHeaderTextStyle}"/>
</Grid>
<Button x:Name="startButton" Content="Start!" HorizontalAlignment="Center" Grid.Row="2" VerticalAlignment="Center"
RenderTransformOrigin="0.562,0.526"/>
<ProgressBar Grid.Column="1" HorizontalAlignment="Left" Height="10" Margin="475,37,0,0" Grid.Row="2" VerticalAlignment="Top" Width="100"/>
<StackPanel Grid.Column="2" Margin="33,37,50,85" Orientation="Vertical" Grid.Row="2">
<ContentControl Content="ContentControl" HorizontalAlignment="Left" VerticalAlignment="Top"/>
</StackPanel>
<Canvas Grid.Column="1" HorizontalAlignment="Left" Height="100"
Margin="475,175,0,0" Grid.Row="1" VerticalAlignment="Top" Width="100"/>
<TextBlock Grid.Column="2" HorizontalAlignment="Left" Margin="24,2,0,0" Grid.Row="2" TextWrapping="Wrap" Text="TextBlock" VerticalAlignment="Top" Style="{StaticResource SubheaderTextStyle}"/>
<VisualStateManager.VisualStateGroups>
<!-- Visual states reflect the application's view state -->
<VisualStateGroup x:Name="ApplicationViewStates">
<VisualState x:Name="FullScreenLandscape"/>
<VisualState x:Name="Filled"/>
<!-- The entire page respects the narrower 100-pixel margin convention for portrait -->
<VisualState x:Name="FullScreenPortrait">
<Storyboard>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="backButton" Storyboard.TargetProperty="Style">
<DiscreteObjectKeyFrame KeyTime="0" Value="{StaticResource PortraitBackButtonStyle}"/>
</ObjectAnimationUsingKeyFrames>
</Storyboard>
</VisualState>
<!-- The back button and title have different styles when snapped -->
<VisualState x:Name="Snapped">
<Storyboard>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="backButton" Storyboard.TargetProperty="Style">
<DiscreteObjectKeyFrame KeyTime="0" Value="{StaticResource SnappedBackButtonStyle}"/>
</ObjectAnimationUsingKeyFrames>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="pageTitle" Storyboard.TargetProperty="Style">
<DiscreteObjectKeyFrame KeyTime="0" Value="{StaticResource SnappedPageHeaderTextStyle}"/>
</ObjectAnimationUsingKeyFrames>
</Storyboard>
</VisualState>
</VisualStateGroup>
</VisualStateManager.VisualStateGroups>
</Grid>
</common:LayoutAwarePage>
源代码
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Basic Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234237
namespace Save_The_Humans
{
/// <summary>
/// A basic page that provides characteristics common to most applications.
/// </summary>
public sealed partial class MainPage : Save_The_Humans.Common.LayoutAwarePage
{
public MainPage()
{
this.InitializeComponent();
}
/// <summary>
/// Populates the page with content passed during navigation. Any saved state is also
/// provided when recreating a page from a prior session.
/// </summary>
/// <param name="navigationParameter">The parameter value passed to
/// <see cref="Frame.Navigate(Type, Object)"/> when this page was initially requested.
/// </param>
/// <param name="pageState">A dictionary of state preserved by this page during an earlier
/// session. This will be null the first time a page is visited.</param>
protected override void LoadState(Object navigationParameter, Dictionary<String, Object> pageState)
{
}
/// <summary>
/// Preserves state associated with this page in case the application is suspended or the
/// page is discarded from the navigation cache. Values must conform to the serialization
/// requirements of <see cref="SuspensionManager.SessionState"/>.
/// </summary>
/// <param name="pageState">An empty dictionary to be populated with serializable state.</param>
protected override void SaveState(Dictionary<String, Object> pageState)
{
}
}
}
错误文本:
InvalidCastException: Unable to cast object of type 'Windows.UI.Text.TextDecorations' to type 'System.Windows.TextDecorationCollection'.
堆栈跟踪
at Microsoft.Expression.DesignSurface.Tools.Text.TextBlockEditProxy.Instantiate()
at Microsoft.Expression.DesignSurface.Tools.Text.TextEditProxy.AddToScene(Boolean visible)
at Microsoft.Expression.DesignSurface.Tools.Text.TextToolBehavior.AddEditProxyToScene(TextEditProxy textEditProxy, Boolean visible)
at Microsoft.Expression.DesignSurface.Tools.Text.TextToolBehavior.FindOrCreateEditProxy(SceneNode textElement, Boolean active)
at Microsoft.Expression.DesignSurface.Tools.Text.TextToolBehavior.BeginTextEdit(SceneNode textElement)
at Microsoft.Expression.DesignSurface.Tools.Text.TextToolBehavior.EditDifferentElement(SceneNode element, Boolean returnFocus)
at Microsoft.Expression.DesignSurface.Tools.Text.TextToolBehavior.OnAttach()
at Microsoft.Expression.DesignSurface.Tools.EventRouter.PushBehavior(ToolBehavior newActiveBehavior)
at Microsoft.Expression.DesignSurface.View.SceneView.TryEnterTextEditMode(Boolean textElementOnly)
at Microsoft.Expression.DesignSurface.SceneCommands.EditTextCommand.Execute()
at Microsoft.Expression.Utility.Commands.CommandTarget.ExecuteCommand(String commandName, CommandInvocationSource invocationSource)
at Microsoft.Expression.Utility.UserInterface.CommandBarButtonBase.<>c__DisplayClass1.<Execute>b__0()
at System.Windows.Threading.ExceptionWrapper.InternalRealCall(Delegate callback, Object args, Int32 numArgs)
at System.Windows.Threading.ExceptionWrapper.TryCatchWhen(Object source, Delegate callback, Object args, Int32 numArgs, Delegate catchHandler)
InnerException: None
为什么我有两个减号?!
我遇到了同样的问题,事实证明,这是因为我使用的是 Windows 10。
就我而言,您无法在较旧或较新的操作系统上构建 Windows 8 应用程序——归根结底,这个 Visual Studio 是为 Windows 8 明确设计的,所以我想这是有道理的。
无论如何,解决问题的方法是使用本书的WPF版本。 你可以在作者的 Github 页面上免费找到它 -> https://github.com/head-first-csharp/third-edition 。
所以我所做的是按照本书的规定安装适用于 Windows 桌面的 Visual Studio 2013 Express,并遵循这条路线。
您首先构建完全相同的 Alien Invasion 应用程序,当我不得不重命名文本块时,一切正常。 其他步骤也是如此。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.