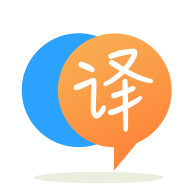
[英]How do you convert integer to character in Java? (5 --> '5')
[英]How to convert a character into an integer in Java?
我是 Java 的初学者,试图弄清楚如何将文本文件中的字符转换为整数。 在这个过程中,我编写了一个程序,它生成一个文本文件,显示哪些字符由哪些整数生成。
package numberchars;
import java.io.FileWriter;
import java.io.IOException;
import java.io.FileReader;
import java.lang.Character;
public class Numberchars {
public static void main(String[] args) throws IOException {
FileWriter outputStream = new FileWriter("NumberChars.txt");
//Write to the output file the char corresponding to the decimal
// from 1 to 255
int counter = 1;
while (counter <256)
{
outputStream.write(counter);
outputStream.flush();
counter++;
}
outputStream.close();
这生成了 NumberChars.txt,其中包含所有数字、所有大写和小写字母,每一端都被其他符号和字形包围。
然后我尝试读取此文件并将其字符转换回整数:
FileReader inputStream = new FileReader("NumberChars.txt");
FileWriter outputStream2 = new FileWriter ("CharNumbers.txt");
int c;
while ((c = inputStream.read()) != -1)
{
outputStream2.write(Character.getNumericValue(c));
outputStream2.flush();
}
}
}
生成的文件CharNumbers.txt
以与 NumberChars.txt 相同的字形开头,但随后为空白。 在 MS Word 中打开文件,我发现 NumberChars 有 248 个字符(包括 5 个空格),而 CharNumbers 有 173 个(包括 8 个空格)。
那么为什么Character.getNumericValue(c)
产生一个整数写入 CharNumbers.txt 呢? 鉴于它没有,为什么至少它不写一个 NumberChars.txt 的精确副本? 非常感谢任何帮助。
Character.getNumericValue
不会做您认为的那样。 如果您阅读Javadoc :
返回指定字符(Unicode 代码点)表示的
int
值。 例如,字符'\Ⅼ'
(罗马数字 50)将返回一个值为50
的 int 。
出错时它返回-1
(在 2s 补码中看起来像0xFF_FF_FF_FF
)。
大多数字符没有这样的“数值”,所以你写出整数,每个填充到 2 个字节(稍后会详细介绍),以相同的方式读回它们,然后开始写入大量0xFFFF
( -1
截断为 2 个字节)由放错位置的Character.getNumericValue
。 我不知道微软Word正在做,但它可能是越来越糊涂你的文件的编码是什么,glomming所有这些字节到0xFF_FF_FF_FF
(因为每个字节的最高位设置)和治疗,作为一个字符。 (使用更适合这类东西的文本编辑器,例如 Notepad++,顺便说一句。)如果您要以字节为单位测量磁盘上文件的大小,它可能仍然是256 chars * 2 bytes/chars = 512 bytes
。
我不确定您在这里要做什么,所以我会注意到InputStreamReader
和OutputStreamWriter
在 (Unicode) 字符基础上工作,编码器默认为系统编码器。 这就是为什么您的整数被填充/截断为 2 个字节的原因。 如果您想要纯字节 IO,请使用FileInputStream
/ FileOutputStream
。 如果您想将int
s 读写为String
s,则需要使用FileWriter
/ FileReader
,但不像您那样。
// Just bytes
// This is a try-with-resources. It executes the code with the decls in it
// but is also like an implicit finally block that calls `close()` on each resource.
try(FileOutputStream fos = new FileOutputStream("bytes.bin")) {
for(int b = 0; b < 256; b++) { // Bytes are signed so we use int.
// This takes an int and truncates it for the lowest byte
fos.write(b);
// Can also fill a byte[] and dump it all at once with overloaded write.
}
}
byte[] bytes = new bytes[256];
try(FileInputStream fis = new FileInputStream("bytes.bin")) {
// Reads up to bytes.length bytes into bytes
fis.read(bytes);
}
// Foreach loop. If you don't know what this does, I think you can figure out from the name.
for(byte b : bytes) {
System.out.println(b);
}
// As Strings
try(FileWriter fw = new FileWriter("strings.txt")) {
for(int i = 0; i < 256; i++) {
// You need a delimiter lest you not be able to tell 12 from 1,2 when you read
// Uses system default encoding
fw.write(Integer.toString(i) + "\n");
}
}
byte[] bytes = new byte[256];
try(
FileReader fr = new FileReader("strings.txt");
// FileReaders can't do stuff like "read one line to String" so we wrap it
BufferedReader br = new BufferedReader(fr);
) {
for(int i = 0; i < 256; i++) {
bytes[i] = Byte.valueOf(br.readLine());
}
}
for(byte b : bytes) {
System.out.println(b);
}
public class MyCLAss {
public static void main(String[] args)
{
char x='b';
System.out.println(+x);//just by witting a plus symbol before the variable you can find it's ascii value....it will give 98.
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.