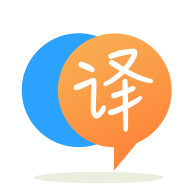
[英]click on the list item, it toggles the line-through sytle class on and off
[英]Why does line-through work only for every other item on this jQuery to do list?
我正在创建一个jQuery待办事项列表,在其中我希望能够添加一个项目,然后单击一个复选框以添加一个直行。 最后,当我单击垃圾箱按钮时,应删除附加的项目。
只要我仅添加一个项目,添加一个直行,然后删除该项目,代码就可以正常工作。
如果添加了更多项目,我仍然可以添加和删除项目,但直通并不适用于所有项目。 看来我只能选择偶数或奇数项作为直通项目。
我的代码如下,这是一个有效的演示 。 作为编码领域的新手,非常感谢您的帮助!
的HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>To Do List</title>
<link href="style.css" type="text/css" rel="stylesheet">
<!--link for the font-awesome garbage bin icon -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
</head>
<body>
<div class="main">
<h1 class="title">To Do List</h1>
<form class="form-box">
<input type="text" class="input-box" placeholder="Add An Item to Your To Do List" onclick="this.placeholder = ''" onblur="this.placeholder = 'Add An Item to Your To Do List'" >
<button type="submit" value="Add" class="input-button" name="input-button">Add</button>
</form>
<!-- A couple of commented-out sample divs that would be added on when <button> is clicked (see app.js)-->
<!--<div class="item-box">
<h3 class="item-text">Lorem ipsum dolor sit amet</h3>
<input type="checkbox" class="checkbox">
<i class="fa fa-trash fa-2x bin-button" aria-hidden="true"></i>
</div>
<div class="item-box">
<h3 class="item-text strikethrough">Lorem ipsum dolor sit amet</h3>
<input type="checkbox" class="checkbox" checked>
<i class="fa fa-trash fa-2x bin-button" aria-hidden="true"></i>
</div>-->
</div>
<!-- JAVASCRIPT -->
<script src="https://code.jquery.com/jquery-2.2.4.min.js" integrity="sha256-BbhdlvQf/xTY9gja0Dq3HiwQF8LaCRTXxZKRutelT44=" crossorigin="anonymous"></script>
<script src="app.js"></script>
<!-- End of JAVASCRIPT -->
</body>
</html>
jQuery的
$(document).ready(function() {
$(".input-button").click(function(evt){
evt.preventDefault();
var value = $(".input-box").val();
var item = "<div class='item-box'> <h3 class='item-text'>" + value + "</h3> <input type='checkbox' class='checkbox'> <i class='fa fa-trash fa-2x bin-button' aria-hidden='true'></i> </div>";
$(".main").append(item);
$(".bin-button").click(function() {
$(this).parent().remove();
});
$(".checkbox").click(function() {
$(this).prev().toggleClass("strikethrough");
});
});
});
的CSS
* {
box-sizing: border-box;
}
body {
background-color: #666;
font-size: 1em;
width: 100%;
}
.main {
margin: 20px;
}
.title {
margin-top: 20px;
margin-bottom: 50px;
text-decoration: underline;
}
.form-box {
width: 40%;
margin: 0 auto 50px auto;
height: auto;
}
.input-box {
width: 50%;
display: block;
margin: 20px auto 0 auto;
}
.input-button {
width: 10%;
display: block;
margin: 20px auto 20px auto;
}
/* styling for div items added through jQuery */
.item-box {
width: 40%;
height: 50px;
margin: 0 auto;
margin-bottom: 10px;
clear: both;
}
.item-box h3, input, i {
display: inline-block;
}
.item-box h3 {
margin-top: 15px;
}
.item-box input {
margin-top: 18.5px;
}
.item-box i {
margin-top: 9px;
}
.item-box input, i {
float: right;
}
.bin-button {
margin-right: 10px;
}
/* Typography */
h1.title {
font-family: sans-serif;
font-size: 3rem;
text-align: center;
}
h3.item-text {
font-size: 1.3rem;
font-family: sans-serif;
font-weight: bold;
letter-spacing: 0.1rem;
}
h3.strikethrough {
text-decoration: line-through;
}
这是因为您使用以下代码重新注册了许多事件:
$(".bin-button").click(function() {
$(this).parent().remove();
});
$(".checkbox").click(function() {
...
});
因此,对于每个添加的待办事项,注册到该复选框的事件数将增加。
例如:第一个待办事项,生活美好,并记录了一个事件。 第二个待办事项本身可以正常工作,但第一个待办事项现在将两个事件绑定到该复选框。 这意味着toggleClass被调用两次。
例如,执行以下操作将显示click事件被多次触发:
let called = 0;
$(document).ready(function() {
$(".input-button").click(function(evt){
evt.preventDefault();
var value = $(".input-box").val();
var item = "<div class='item-box'> <h3 class='item-text'>" + value + "</h3> <input type='checkbox' class='checkbox'> <i class='fa fa-trash fa-2x bin-button' aria-hidden='true'></i> </div>";
$(".main").append(item);
$(".bin-button").click(function() {
$(this).parent().remove();
});
$(".checkbox").click(function() {
called++;
$(this).prev().toggleClass("strikethrough");
console.log(called);
});
});
});
您可以改用$(item)
创建该元素,并将click事件添加到该元素,然后再将其添加到dom中。 例如:
let called = 0;
$(document).ready(function() {
$(".input-button").click(function(evt){
evt.preventDefault();
var value = $(".input-box").val();
var item = $("<div class='item-box'> <h3 class='item-text'>" + value + "</h3> <input type='checkbox' class='checkbox'> <i class='fa fa-trash fa-2x bin-button' aria-hidden='true'></i> </div>");
item.find(".bin-button").click(function() {
$(this).parent().remove();
});
item.find(".checkbox").click(function() {
called++;
$(this).prev().toggleClass("strikethrough");
console.log(called);
});
$(".main").append(item);
});
});
您应该在输入框的click函数范围之外声明复选框的click函数。 另外,由于您的复选框是动态生成的,因此您将需要使用以下语法:
$(document).on('click','.checkbox',function() {
$(this).prev().toggleClass("strikethrough");
}
因此,完整的JQuery代码应如下所示:
$(document).ready(function() {
$(".input-button").click(function(evt){
evt.preventDefault();
var value = $(".input-box").val();
var item = "<div class='item-box'> <h3 class='item-text'>" + value + "</h3> <input type='checkbox' class='checkbox'> <i class='fa fa-trash fa-2x bin-button' aria-hidden='true'></i> </div>";
$(".main").append($(item));
});
$(document).on("click", ".bin-button", function() {
$(this).parent().remove();
});
$(document).on("click", ".checkbox", function() {
$(this).prev().toggleClass("strikethrough");
});
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.