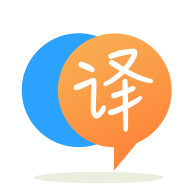
[英]“TypeError: Object(…) is not a function” react-redux-firebase
[英]react-redux-firebase - TypeError: Cannot read property 'firebase' of undefined at FirebaseConnect.componentWillMount
我已经从https://github.com/tiberiuc/redux-react-firebase复制了示例:
rootReducer.js:
import { createStore, combineReducers, compose } from 'redux'
import { reactReduxFirebase, firebaseStateReducer } from 'react-redux-firebase'
// Add Firebase to reducers
const rootReducer = combineReducers({
firebase: firebaseStateReducer
})
// Firebase config
const config = {
apiKey: 'AIzaSyCXydC2cGp_8BNEMqLqpHVSczGyti7j6A8',
authDomain: 'cabal-production.firebaseapp.com',
databaseURL: 'https://cabal-production.firebaseio.com',
storageBucket: 'cabal-production.appspot.com'
}
// Add redux Firebase to compose
const createStoreWithFirebase = compose(
reactReduxFirebase(config, { userProfile: 'users' }),
)(createStore)
// Create store with reducers and initial state
const initialState = {}
const store = createStoreWithFirebase(rootReducer, initialState)
todos.js:
import React, { Component } from 'react'
import PropTypes from 'prop-types' // can also come from react if react <= 15.4.0
import { connect } from 'react-redux'
import { compose } from 'redux'
import {
firebaseConnect,
isLoaded,
isEmpty,
dataToJS,
pathToJS
} from 'react-redux-firebase'
class Todos extends Component {
static propTypes = {
todos: PropTypes.object,
auth: PropTypes.object,
firebase: PropTypes.object
}
addTodo = () => {
const { newTodo } = this.refs
return this.props.firebase
.push('/todos', { text: newTodo.value, done: false })
.then(() => {
newTodo.value = ''
console.log('Todo Created!')
})
.catch((err) => {
console.log('Error creating todo:', err) // error is also set to authError
})
}
render() {
const { todos } = this.props;
// Build Todos list if todos exist and are loaded
const todosList = !isLoaded(todos)
? 'Loading'
: isEmpty(todos)
? 'Todo list is empty'
: Object.keys(todos).map(
(key, id) => (
<TodoItem key={key} id={id} todo={todos[key]}/>
)
)
return (
<div>
<h1>Todos</h1>
<ul>
{todosList}
</ul>
<input type="text" ref="newTodo" />
<button onClick={this.handleAdd}>
Add
</button>
</div>
)
}
}
export default compose(
firebaseConnect([
'todos' // { path: 'todos' } // object notation
]),
connect(
({ firebase }) => ({ // state.firebase
todos: dataToJS(firebase, 'todos'), // in v2 todos: firebase.data.todos
auth: pathToJS(firebase, 'auth') // in v2 todos: firebase.auth
})
)
)(Todos)
但是,当我运行它时,出现以下错误:
500
Internal Server Error.
Cannot read property 'firebase' of undefined
TypeError: Cannot read property 'firebase' of undefined
at FirebaseConnect.componentWillMount ([MYAPP]/node_modules/react-redux-firebase/lib/firebaseConnect.js:72:45)
at [MYAPP]/node_modules/react-dom/lib/ReactCompositeComponent.js:347:23
at measureLifeCyclePerf ([MYAPP]/node_modules/react-dom/lib/ReactCompositeComponent.js:75:12)
at ReactCompositeComponentWrapper.performInitialMount ([MYAPP]/node_modules/react-dom/lib/ReactCompositeComponent.js:346:9)
at ReactCompositeComponentWrapper.mountComponent ([MYAPP]/node_modules/react-dom/lib/ReactCompositeComponent.js:257:21)
at Object.mountComponent ([MYAPP]/node_modules/react-dom/lib/ReactReconciler.js:45:35)
at ReactCompositeComponentWrapper.performInitialMount ([MYAPP]/node_modules/react-dom/lib/ReactCompositeComponent.js:370:34)
at ReactCompositeComponentWrapper.mountComponent ([MYAPP]/node_modules/react-dom/lib/ReactCompositeComponent.js:257:21)
at Object.mountComponent ([MYAPP]/node_modules/react-dom/lib/ReactReconciler.js:45:35)
at ReactCompositeComponentWrapper.performInitialMount ([MYAPP]/node_modules/react-dom/lib/ReactCompositeComponent.js:370:34)
我在许多项目中将redux与firebase一起使用,对我来说,这种方式更干净:
首先,您必须安装firebase npm install --save firebase redux-thunk
在您的操作文件中初始化firebase配置
import * as firebase from 'firebase'
const CONFIG={
apiKey: 'AIzaSyCXydC2cGp_8BNEMqLqpHVSczGyti7j6A8',
authDomain: 'cabal-production.firebaseapp.com',
databaseURL: 'https://cabal-production.firebaseio.com',
storageBucket: 'cabal-production.appspot.com'
}
firebase.initializeApp(CONFIG);
//then you have to create an action creators for make the request to your firebase database, in this case, would be like this:
export function fetchTodos(){
return dispatch => {
const todos = firebase.database().ref()
return todos.on('value', snapshot => {
dispatch({
type:FETCH_TODOS,
payload: snapshot.val()
})
})
}
}
每次数据库更改时,它将向您的reducer派发一个操作,并使您的reducer保持清洁。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.