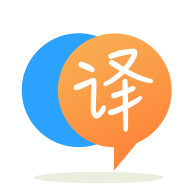
[英]Using Highmaps, how can I use jQuery fadeIn/fadeOut to fade the data while keeping the map visible?
[英]HighMaps how to update data using jQuery
我有一个 HighMaps 美国县地图,在带有固定数据的网页上显示得很漂亮(在下面的代码中命名为 tableResult)。 这是 jQuery 网页的一部分,该网页根据用户从页面上的各种选项中选择的参数为美国各县着色。 在他们选择参数后,他们点击一个按钮来查看他们选择的最佳县(三种不同的颜色)。 结果可能只是几个县或数百个。 提交按钮正在调用一个 Web 服务,该服务返回一个字符串,其格式与下面的 tableResult 类似,但具有基于其选择的个性化值。 所有这些都很好用!
我很确定这是一个范围问题,但我无法弄清楚每次用户更改他们的选择并激活提交按钮时如何更新地图。 现在,用户参数都是以这个开头的 jQuery 控件:
<script type="text/javascript">
jQuery(document).ready(function () {
alert("jQuery document ready");
var e = jQuery(this).val();
return e && (window.location = e), !1
});
</script>
接下来是几个用户输入选择控件,所有这些都可以工作,然后是我下面的 HighMaps 代码。
我很尴尬地说我不知道如何用从 Web 服务返回的数据字符串填充 tableResult 值。 这是我调用 Web 服务的函数(并且 Web 服务正按照我的需要返回一个不错的 JSON 字符串):
function GetCounties(pk, rk) {
jQuery.support.cors = true;
$.ajax({
url: "http://www.mywebsite.com/api/products/2",
type: "GET",
dataType: "json",
success: function (d) {
data = JSON.stringify(d);
alert("API found data: " + JSON.stringify(d));
},
error: function (d) {
alert("API found error: " + JSON.stringify(d));
}
});
}
下面是显示地图的 HighMaps 代码(目前只有 tableResult 变量中的两个固定县作为占位符)。
$.noConflict();
(function ($) {
// eliminate the following two lines when actual tableResult data is coming from table service
var tableResult = '[{"code":"us-al-001","name":"Autauga County, AL","value":1}, {"code":"us-il-019","name":"Champaign County, IL","value":3}]';
data = JSON.parse(tableResult);
var countiesMap = Highcharts.geojson(Highcharts.maps["countries/us/us-all-all"]);
var lines = Highcharts.geojson(Highcharts.maps["countries/us/us-all-all"], "mapline");
// add state acronym for tooltip
Highcharts.each(countiesMap, function (mapPoint) {
var state = mapPoint.properties["hc-key"].substring(3, 5);
mapPoint.name = mapPoint.name + ", " + state.toUpperCase();
});
var options = {
chart: {
borderWidth: 1,
marginRight: 50 // for the legend
},
exporting: {
enabled: false
},
title: {
text: "My Best Locations"
},
legend: {
layout: "vertical",
align: "right",
floating: true,
valueDecimals: 0,
valueSuffix: "",
backgroundColor: "white",
symbolRadius: 0,
symbolHeight: 0
},
mapNavigation: {
enabled: false
},
colorAxis: {
dataClasses: [{
from: 1,
to: 1,
color: "#004400",
name: "Perfect!"
}, {
from: 2,
to: 2,
color: "#116611",
name: "Very Nice!"
}, {
from: 3,
to: 3,
color: "#55AA55",
name: "Good Fit"
}]
},
tooltip: {
headerFormat: "",
formatter: function () {
str = "Error";
if (this.point.value == 1) {
str = "Perfect!";
}
if (this.point.value == 2) {
str = "Very Nice!";
}
if (this.point.value == 3) {
str = "Good Fit";
}
return this.point.name + ": <b>" + str + "</b>";
}
},
plotOptions: {
mapline: {
showInLegend: false,
enableMouseTracking: false
}
},
series: [{
mapData: countiesMap,
data: data,
joinBy: ["hc-key", "code"],
borderWidth: 1,
states: {
hover: {
color: "brown"
}
}
}, {
type: "mapline",
name: "State borders",
data: [lines[0]],
color: "black"
}]
};
// Instanciate the map
$("#map-container").highcharts("Map", options);
})(jQuery);
我需要的是,每次用户点击提交按钮时,都会使用 Web 服务的结果更新地图。 任何想法将不胜感激!
更改事件添加到按钮的方式(例如使用bind
)。 bind
函数的第二个参数是包含附加参数(你的pk
和rk
)的对象。 它看起来像这样:
jQuery('.button').bind('click', {
pk: 'C4-7P4-6L3-5',
rk: 'H5F4M3B4T3'
}, function(e) {
var data = e.data,
pk = data.pk,
rk = data.rk;
GetCounties(pk, rk);
});
另外,请记住使用来自 ajax 的数据更新图表:
$("#map-container").highcharts().series[0].update({
data: JSON.parse(d)
});
就像你说的,这可能是一个范围问题。 检查的一种方法是更改单击功能以使用类似的功能。
$("body").on("click", ".button", function() { // just some test variables, pass the correct values for pk rk however you need. var pk = $("#pk").val(); var rk = $("#rk").val(); GetCounties(pk,rk); }); function GetCounties(pk, rk) { // do something console.log(pk, rk); jQuery.support.cors = true; $.ajax({ url: "http://www.mywebsite.com/api/products/2", type: "GET", dataType: "json", success: function(d) { data = JSON.stringify(d); alert("API found data: " + JSON.stringify(d)); }, error: function(d) { alert("API found error: " + JSON.stringify(d)); } }); }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> TEST pk:<input id="pk" type="text" value="C4-7P4-6L3-5"><br> TEST rk:<input id="rk" type="text" value="H5F4M3B4T3"> <br> <li><button class="button">Update Counties</button></li>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.