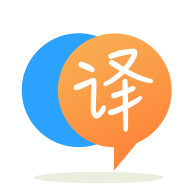
[英]How to search specific attribute within a tag and remove it using javascript regex
[英]how to search within a p tag using Javascript
我想用普通的 javascript 搜索<p>
但我不知道怎么做。 我试过用谷歌搜索它,我已经搜索了 stackOverflow,但我不知道如何在<p>
内部搜索。
<input id="search">
<p>Some text content I want</p>
<script>
//script to search the above "p"
</script>
我希望 javascript 为其找到的匹配项设置样式。
这是我所拥有的Js Fiddle 。
概括:
你可以这样做:
function myfunction(ctrl) {
var TextInsideP = ctrl.getElementsByTagName('p')[0].innerHTML;
}
您的p
标签内容现在位于变量TextInsideP
,您可以TextInsideP
使用它们。
您可以先获取#search 元素中第一个p 元素的内容,然后执行搜索。 将返回搜索元素的字符串位置,如果未找到,将返回 -1。
var text = document.querySelector('#search p')[0].innerHTML;
var n = text.search("text to find");
if (n !== -1) {
// Found!
} else {
// Not found
}
如果您要在 'p' 标签中搜索特定单词,则必须使用选择器来抓取它。 然后你必须拆分单词串,遍历它们并使用 indexOf 搜索(见下文)
var input = document.getElementById('search'); input.addEventListener('keyup', function() { var val = input.value; searchExactMatch(val); searchPartialMatch(val); }) var p = document.getElementById('test'); var text = p.innerHTML; var splits = text.split(' '); function searchExactMatch(value) { var word = value; var index = splits.indexOf(word); if (index > -1) { console.log('full match with: ', splits[index]); } } function searchPartialMatch(value) { var word = value; for (var t = 0; t < splits.length; t++) { var split = splits[t]; if (split.indexOf(word) > -1) { console.log('the typed string is a partial match with: ', split); } } }
<p id="test">text goes here</p> <input id="search" />
您可以使用document.getElementsBytagName('tagName')
访问打开和关闭标签之间的文本。
<!DOCTYPE html> <html> <body> <p>Sed ut perspiciatis, unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam eaque ipsa, quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt, explicabo. Nemo enim ipsam voluptatem, quia voluptas sit, aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos, qui ratione voluptatem sequi nesciunt, neque porro quisquam est, qui dolorem ipsum, quia dolor sit amet consectetur adipisci[ng] velit, sed quia non numquam [do] eius modi tempora inci[di]dunt, ut labore et dolore magnam aliquam quaerat voluptatem. Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur? Quis autem vel eum iure reprehenderit, qui in ea voluptate velit esse, quam nihil molestiae consequatur, vel illum, qui dolorem eum fugiat, quo voluptas nulla pariatur?</p> <button onclick="myFunction()">Try it</button> <script> function myFunction() { //document.write(document.getElementsByTagName("p")[0].innerText); //Old text from the tag <p> document.getElementsByTagName("p")[0].innerText = "This works great."; //new text inside tag <p>. } </script> </body> </html>
我认为如果您想突出显示内容,则需要将某些内容替换为其他内容。 我的想法如下:使 yourSearchWord 加粗:
let paragraph = document.getElementById('idHere').innerHTML;
document.getElementById('idHere').innerHTML = paragraph.split('yourSearchWord').join('<b>yourSearchWord</b>');
我编写了一个函数,当输入中键入的值与p
元素中的字符串完全匹配时,该函数将为您突出显示p
元素。
var elements = document.querySelectorAll('p'); var HIGHLIGHT_STYLE_PREFIX = '<span style="background-color:yellow">'; var HIGHLIGHT_STYLE_SUFFIX = '</span>'; document.getElementById('search').addEventListener('input', highlightMatches); function highlightMatches() { var queryTerm = this.value.trim(); for (let i = 0; i < elements.length; i++) { elements[i].childNodes.forEach(function(currentValue) { if (currentValue.data === queryTerm) { elements[i].innerHTML = HIGHLIGHT_STYLE_PREFIX + elements[i].innerHTML + HIGHLIGHT_STYLE_SUFFIX; } else { if (elements[i].innerHTML.indexOf(HIGHLIGHT_STYLE_SUFFIX) > -1) { elements[i].innerHTML = elements[i].innerHTML.replace(HIGHLIGHT_STYLE_PREFIX, '').replace(HIGHLIGHT_STYLE_SUFFIX, ''); } } }); } }
<input id="search" /> <p>Sed ut perspiciatis</p>, unde <p>omnis</p> iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam eaque ipsa, quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt, explicabo. <p>Nemo enim ipsam voluptatem, quia voluptas sit, aspernatur aut odit aut fugit, sed quia</p> <p>omnis</p> <p>consequuntur magni dolores eos, qui ratione voluptatem sequi</p> nesciunt, neque porro quisquam est, qui dolorem ipsum, quia dolor sit amet consectetur adipisci[ng] velit, sed quia non numquam [do] eius modi tempora inci[di]dunt, ut labore et dolore magnam aliquam quaerat <p>voluptatem. Ut enim ad minima veniam, quis nostrum exercitationem</p> ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur? Quis autem vel eum iure reprehenderit, qui in ea voluptate velit esse, quam nihil molestiae <p>consequatur, vel illum, qui dolorem eum fugiat, quo voluptas nulla pariatur?</p>
我找到了答案。 感谢所有回答我问题的人!
$(function() {
// the input field
var $input = $("input[type='search']"),
// clear button
$clearBtn = $("button[data-search='clear']"),
// prev button
$prevBtn = $("button[data-search='prev']"),
// next button
$nextBtn = $("button[data-search='next']"),
// the context where to search
$content = $(".content"),
// jQuery object to save <mark> elements
$results,
// the class that will be appended to the current
// focused element
currentClass = "current",
// top offset for the jump (the search bar)
offsetTop = 50,
// the current index of the focused element
currentIndex = 0;
/**
* Jumps to the element matching the currentIndex
*/
function jumpTo() {
if ($results.length) {
var position,
$current = $results.eq(currentIndex);
$results.removeClass(currentClass);
if ($current.length) {
$current.addClass(currentClass);
position = $current.offset().top - offsetTop;
window.scrollTo(0, position);
}
}
}
/**
* Searches for the entered keyword in the
* specified context on input
*/
$input.on("input", function() {
var searchVal = this.value;
$content.unmark({
done: function() {
$content.mark(searchVal, {
separateWordSearch: true,
done: function() {
$results = $content.find("mark");
currentIndex = 0;
jumpTo();
}
});
}
});
});
/**
* Clears the search
*/
$clearBtn.on("click", function() {
$content.unmark();
$input.val("").focus();
});
/**
* Next and previous search jump to
*/
$nextBtn.add($prevBtn).on("click", function() {
if ($results.length) {
currentIndex += $(this).is($prevBtn) ? -1 : 1;
if (currentIndex < 0) {
currentIndex = $results.length - 1;
}
if (currentIndex > $results.length - 1) {
currentIndex = 0;
}
jumpTo();
}
});
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.