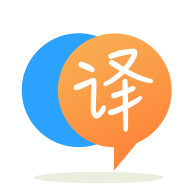
[英]I am trying to create dynamic html options from a JSON file using javascript
[英]I am trying to pluck the median from a json file using javascript
尝试从各个年龄段中提取平均值并处理其中一个年龄段的缺失值。
var data={"users":[
{
"first_name":"Mikey",
"last_name":"Mouse",
"age": 24
},
{
"first_name":"Donald",
"lastName":"Duck",
"age": 29
},
{
"first_name":"Woody",
"lastName":"Woodpecker",
"age":
},
{
"first_name":"Bugs",
"lastName":"Bunny",
"age": 32
}
]}
使用Ramda ,您可以执行以下操作(不知道您使用的是什么库,没有提供任何代码):
const ages = R.compose(R.map(R.propOr(0, 'age')), R.propOr([], 'users'))
const mean = R.converge(R.divide, [R.sum, R.length])
const meanAges = R.compose(mean, ages)
console.log(meanAges(data)) // 21.25
如果未指定年龄,则R.propOr(0, 'age')
年龄为0
。
const data={"users":[ { "first_name":"Mikey", "last_name":"Mouse", "age": 24 }, { "first_name":"Donald", "lastName":"Duck", "age": 29 }, { "first_name":"Woody", "lastName":"Woodpecker" }, { "first_name":"Bugs", "lastName":"Bunny", "age": 32 } ]} const ages = R.compose(R.map(R.propOr(0, 'age')), R.propOr([], 'users')) const mean = R.converge(R.divide, [R.sum, R.length]) const meanAges = R.compose(mean, ages) console.log(meanAges(data))
<script src="https://cdnjs.cloudflare.com/ajax/libs/ramda/0.22.1/ramda.min.js"></script>
删除没有设定年龄的人,然后映射以仅获取年龄,然后排序并返回medean:
function getMedeanAge(data) {
let ages = data
.filter(cartoon => cartoon.age !== undefined)
.map(cartoon => cartoon.age)
.sort((a,b) => a > b);
var middleIndex = ages.length / 2;
return ages[Math.floor(middleIndex)] + ages[Math.ceil(middleIndex) / 2;
}
您可以对Array#forEach
使用单循环方法,方法是收集所有值的sum
和有效age
的计数。 然后,可以将所有年龄段的总和除以count
得到平均值。
var data = { users: [{ first_name: "Mikey", last_name: "Mouse", age: 24 }, { first_name: "Donald", lastName: "Duck", age: 29 }, { first_name: "Woody", lastName: "Woodpecker", age: null }, { first_name: "Bugs", lastName: "Bunny", age: 32 }] }, sum = 0, count = 0, average; data.users.forEach(function (user) { if (typeof user.age === 'number') { sum += user.age; ++count; } }); average = sum / count; console.log(average);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.