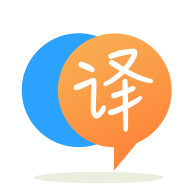
[英]When using the Camera app in Android, how can I return the whole image instead of just the thumbnail?
[英]How can i get the output as just the city name instead of whole address in my App when using Google API?
即使用户搜索城市中的任何地区,输出也应该是城市名称。
package com.example.rajat.location;
import android.content.Intent; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.util.Log; import android.view.View; import android.widget.TextView;
import com.google.android.gms.common.GooglePlayServicesNotAvailableException; import com.google.android.gms.common.GooglePlayServicesRepairableException; import com.google.android.gms.common.api.Status; import com.google.android.gms.location.places.Place; import com.google.android.gms.location.places.ui.PlaceAutocomplete;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
/*
* In this method, Start PlaceAutocomplete activity
* PlaceAutocomplete activity provides--
* a search box to search Google places
*/
public void findPlace(View view) {
try {
Intent intent =
new PlaceAutocomplete
.IntentBuilder(PlaceAutocomplete.MODE_FULLSCREEN)
.build(this);
startActivityForResult(intent, 1);
} catch (GooglePlayServicesRepairableException e) {
// TODO: Handle the error.
} catch (GooglePlayServicesNotAvailableException e) {
// TODO: Handle the error.
}
}
// A place has been received; use requestCode to track the request.
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == 1) {
if (resultCode == RESULT_OK) {
// retrive the data by using getPlace() method.
Place place = PlaceAutocomplete().getPlace(this, data);
Log.e("Tag", "Place: " + place.getAddress() + place.getPhoneNumber());
((TextView) findViewById(R.id.searched_address))
.setText(place.getName()+",\n"+
place.getAddress() +"\n" + place.getPhoneNumber());
} else if (resultCode == PlaceAutocomplete.RESULT_ERROR) {
Status status = PlaceAutocomplete.getStatus(this, data);
// TODO: Handle the error.
Log.e("Tag", status.getStatusMessage());
} else if (resultCode == RESULT_CANCELED) {
// The user canceled the operation.
}
}
} }
您需要使用特定于Google Places API的密钥来从JSON响应对象中检索城市名称。
此链接显示您返回的JSON响应(键值对) https://developers.google.com/places/web-service/details
检查您的JSON响应对象(我相信这是您的“ Place”对象)。
在这行代码上运行调试语句。 Place place = PlaceAutocomplete()。getPlace(this,data);
知道城市的键后,获取城市值,然后仅用城市值填充UI。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.