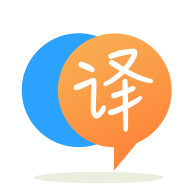
[英]Update an input if checkbox is selected on table row in javascript
[英]How to display selected HTML table row value into input type radio and checkbox using JavaScript
我有一个HTML表,该表从MySQL数据库中加载信息,还有一个表单来添加,更新和加载该Tabla的选定行。 为了使表格和表格适合HTML文档,该表格的某些行被隐藏。
当选择或单击一行时,表单中的字段会加载表的可见和不可见行中包含的信息,但是我不知道该怎么做,将输入类型设置为radio和checkbox选中。 我的意思是,如果某行在“状态”(Status)的隐藏列中的值为3,该如何设置单选输入为“ Widower”形式。 另外,如果某些复选框为true(或值为1),如何将其正确设置为选中状态? 任何想法?
SAMPLE OF HTML TABLE
+----+---------+---------+---------+-----+--------+----------+
| id | Name | L-name | Month | Day | Status | Category |
+----+---------+---------+---------+-----+--------+----------+
| 1 | Luis | Lopez | January | 7 | 1 | 3 |
| 2 | Carlos | Cooper | March | 12 | 3 | 1 |
| 3 | Ana | Snow | December| 3 | 2 | 1 |
+----+---------+---------+---------+-----+--------+----------+
我总结了一下代码,它可以工作,但是脚本中需要单选和复选框:
<!--Here's the form-->
<div class="fields">
<form action="" method="POST">
<fieldset>
<legend>Personal Information: </legend>
Name: <input type="text" required name="nombre" id="name"><br>
Last Name: <input type="text" required name="apellido" id="l-name"><br>
<label>Birthday: </label>
<select name="mes" id="month">
<option value="">Choose month</option>
<option value="January ">January </option>
<option value="February ">February </option>
<!--And so on till December-->
</select>
Day: <input type="number" name="dia" min="1" max="31" value="1" id="day"><br>
Status:<input type="radio" name="estado" value="1" id="stage" checked> Single
<input type="radio" name="estado" value="2"> Married
<input type="radio" name="estado" value="3"> Widower
<input type="radio" name="estado" value="4"> Divorced<br><br>
<fieldset>
<legend>Category:</legend>
<input type="radio" name="categoria" value="1" id="tag"> Gentleman
<input type="radio" name="categoria" value="2" id="tag"> Lady
<input type="radio" name="categoria" value="3" id="tag"> young
<input type="radio" name="categoria" value="4" id="tag"> Child<br>
</fieldset>
</fieldset>
<fieldset>
<legend>Other Information: </legend>
Available:
<input type="radio" name="yes" value="1">Yes
<input type="radio" name="no" value="0">No<br><br>
<fieldset>
<legend>Skill:</legend>
<input type="checkbox" name="skill" value="1"> HTML
<input type="checkbox" name="skill" value="2"> JS
<input type="checkbox" name="skill" value="3"> Python
<input type="checkbox" name="skill" value="4"> JAVA
</fieldset>
<input type="reset" value="Reset">
<input type="submit" value="Update">
<input type="submit" name="send" value="Agregar">
</fieldset>
</form>
</div> <!--End field-->
<!--Here's the form-->
<div class="tabla">
<table id="table">
<thead>
<th style="display:none";>id</th>
<th>Name</th>
<th>Last name</th>
<th style="display:none";>Other</th>
<th style="display:none";>Other</th>
</thead>
<tbody>
<?php while($user = mysqli_fetch_array($datos)){ ?>
<tr>
<td style="display:none";><?php echo $user['id']; ?></td>
<td><?php echo $user['nombre']; ?></td>
<td><?php echo $user['apellido']; ?></td>
<td style="display:none";><?php echo $user['mes']; ?></td>
<td style="display:none";><?php echo $user['dia']; ?></td>
<td style="display:none";><?php echo $user['estado']; ?></td>
<td style="display:none";><?php echo $user['categoria']; ?></td>
</tr>
<?php } ?>
</tbody>
</table>
</div> <!--End tabla-->
<!--JS to load row information to form using id-->
<script>
var table = document.getElementById('table'),rIndex;
for (var i = 1; i < table.rows.length; i++){
table.rows[i].onclick = function(){
rIndex = this.rowsIndex;
document.getElementById("name").value = this.cells[1].innerHTML;
document.getElementById("l-name").value = this.cells[2].innerHTML;
document.getElementById("month").value = this.cells[3].innerHTML;
document.getElementById("day").value = this.cells[4].innerHTML;
document.getElementById("stage").value = this.cells[5].innerHTML;
document.getElementById("tag").value = this.cells[6].innerHTML;
};
}
</script>
我还尝试了一些创建了MySQL行以保存名为skill1,skill2,skill3等技能的操作,但没有成功:
<!--radioboxes skills-->
<input type="checkbox" id="php" value="php"> PHP
<input type="checkbox" id="python" value="python"> PYTHON
<input type="checkbox" id="html" value="html"> HTML
<input type="checkbox" id="js" value="js"> JS
<!--hidden rows of the table that load the MySQL data-->
<td style="display:none";><?php echo $user['php']; ?></td>
<td style="display:none";><?php echo $user['python']; ?></td>
<td style="display:none";><?php echo $user['html']; ?></td>
<td style="display:none";><?php echo $user['js']; ?></td>
<!--js code-->
....
document.getElementById("php" + this.cells[4].innerHTML.trim().toLowerCase()).checked = true;
document.getElementById("python" + this.cells[5].innerHTML.trim().toLowerCase()).checked = true;
document.getElementById("html" + this.cells[6].innerHTML.trim().toLowerCase()).checked = true;
document.getElementById("js" + this.cells[7].innerHTML.trim().toLowerCase()).checked = true;
id
属性应该是唯一的。 有四个具有相同id
input
元素,所以这是错误的:
<input type="radio" name="categoria" value="1" id="tag"> Gentleman
<input type="radio" name="categoria" value="2" id="tag"> Lady
<input type="radio" name="categoria" value="3" id="tag"> young
<input type="radio" name="categoria" value="4" id="tag"> Child
我假设<?php echo $user['categoria']; ?>
<?php echo $user['categoria']; ?>
计算出已知值列表中的某个字符串值,因此我建议以这种方式更改id
:
<input type="radio" name="categoria" value="1" id="tag_gentleman"> Gentleman
<input type="radio" name="categoria" value="2" id="tag_lady"> Lady
<input type="radio" name="categoria" value="3" id="tag_young"> young
<input type="radio" name="categoria" value="4" id="tag_child"> Child
然后,您可以更改此JS代码:
document.getElementById("tag").value = this.cells[6].innerHTML;
变成这个:
document.getElementById("tag_" + this.cells[6].innerHTML.trim().toLowerCase()).checked = true;
这应该针对并设置适当的无线电输入。 您可以通过这种方式设置其他广播和复选框输入。 另外,您可能希望在将所有复选框设置为另一行之前清除所有复选框的状态。
编辑:
我已经对上面的代码进行了更正:1.固定trim
函数-我以PHP方式而不是JS方式使用了它; 2.添加到toLowerCase
并将所有id
重命名为小写。
jsFiddle中有一个基于您的PasteBin示例的工作示例 。
编辑2:
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.