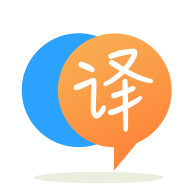
[英]SVG smoothly morphing shape into other predefined shapes with Javascript
[英]Creating svg paths with javascript(shape morphing)
所以我有一个用于形状变形的类:
class ShapeOverlays {
constructor(elm) {
this.elm = elm;
this.path = elm.querySelectorAll('path');
this.numPoints = 18;
this.duration = 600;
this.delayPointsArray = [];
this.delayPointsMax = 300;
this.delayPerPath = 100;
this.timeStart = Date.now();
this.isOpened = false;
this.isAnimating = false;
}
toggle() {
this.isAnimating = true;
const range = 4 * Math.random() + 6;
for (var i = 0; i < this.numPoints; i++) {
const radian = i / (this.numPoints - 1) * Math.PI;
this.delayPointsArray[i] = (Math.sin(-radian) + Math.sin(-radian * range) + 2) / 4 * this.delayPointsMax;
}
if (this.isOpened === false) {
this.open();
} else {
this.close();
}
}
open() {
this.isOpened = true;
this.elm.classList.add('is-opened');
this.timeStart = Date.now();
this.renderLoop();
}
close() {
this.isOpened = false;
this.elm.classList.remove('is-opened');
this.timeStart = Date.now();
this.renderLoop();
}
updatePath(time) {
const points = [];
for (var i = 0; i < this.numPoints + 1; i++) {
points[i] = ease.cubicInOut(Math.min(Math.max(time - this.delayPointsArray[i], 0) / this.duration, 1)) * 100
}
let str = '';
str += (this.isOpened) ? `M 0 0 V ${points[0]} ` : `M 0 ${points[0]} `;
for (var i = 0; i < this.numPoints - 1; i++) {
const p = (i + 1) / (this.numPoints - 1) * 100;
const cp = p - (1 / (this.numPoints - 1) * 100) / 2;
str += `C ${cp} ${points[i]} ${cp} ${points[i + 1]} ${p} ${points[i + 1]} `;
}
str += (this.isOpened) ? `V 0 H 0` : `V 100 H 0`;
return str;
}
render() {
if (this.isOpened) {
for (var i = 0; i < this.path.length; i++) {
this.path[i].setAttribute('d', this.updatePath(Date.now() - (this.timeStart + this.delayPerPath * i)));
}
} else {
for (var i = 0; i < this.path.length; i++) {
this.path[i].setAttribute('d', this.updatePath(Date.now() - (this.timeStart + this.delayPerPath * (this.path.length - i - 1))));
}
}
}
renderLoop() {
this.render();
if (Date.now() - this.timeStart < this.duration + this.delayPerPath * (this.path.length - 1) + this.delayPointsMax) {
requestAnimationFrame(() => {
this.renderLoop();
});
}
else {
this.isAnimating = false;
}
}
}
(function() {
const elmHamburger = document.querySelector('.hamburger');
const gNavItems = document.querySelectorAll('.global-menu__item');
const elmOverlay = document.querySelector('.shape-overlays');
const overlay = new ShapeOverlays(elmOverlay);
elmHamburger.addEventListener('click', () => {
if (overlay.isAnimating) {
return false;
}
overlay.toggle();
if (overlay.isOpened === true) {
elmHamburger.classList.add('is-opened-navi');
for (var i = 0; i < gNavItems.length; i++) {
gNavItems[i].classList.add('is-opened');
}
} else {
elmHamburger.classList.remove('is-opened-navi');
for (var i = 0; i < gNavItems.length; i++) {
gNavItems[i].classList.remove('is-opened');
}
}
});
}());
有人可以解释一下此代码吗? 我真的不知道如何使用时间创建路径,如何放置点以及如何修改它。范围是做什么用的? 为什么三角函数用于delayPointsArray?
基本上这是我不了解的部分:
updatePath(time) {
const points = [];
for (var i = 0; i < this.numPoints + 1; i++) {
points[i] = ease.cubicInOut(Math.min(Math.max(time - this.delayPointsArray[i], 0) / this.duration, 1)) * 100
}
let str = '';
str += (this.isOpened) ? `M 0 0 V ${points[0]} ` : `M 0 ${points[0]} `;
for (var i = 0; i < this.numPoints - 1; i++) {
const p = (i + 1) / (this.numPoints - 1) * 100;
const cp = p - (1 / (this.numPoints - 1) * 100) / 2;
str += `C ${cp} ${points[i]} ${cp} ${points[i + 1]} ${p} ${points[i + 1]} `;
}
str += (this.isOpened) ? `V 0 H 0` : `V 100 H 0`;
return str;
}
render() {
if (this.isOpened) {
for (var i = 0; i < this.path.length; i++) {
this.path[i].setAttribute('d', this.updatePath(Date.now() - (this.timeStart + this.delayPerPath * i)));
}
} else {
for (var i = 0; i < this.path.length; i++) {
this.path[i].setAttribute('d', this.updatePath(Date.now() - (this.timeStart + this.delayPerPath * (this.path.length - i - 1))));
}
}
}
为什么要用时间? 这样做的目的是什么:
points[i] = ease.cubicInOut(Math.min(Math.max(time - this.delayPointsArray[i], 0) / this.duration, 1)) * 100
如果查看如何调用updatePath()
,则如下所示:
this.updatePath(Date.now() - (this.timeStart + this.delayPerPath * i))
因此,传入的time
值是当前时间与我们正在使用的路径的开始时间之间的差。
那么您感兴趣的代码行是做什么的呢?
points[i] = ease.cubicInOut(Math.min(Math.max(time - this.delayPointsArray[i], 0) / this.duration, 1)) * 100
我将忽略delayPointsArray
。 它会根据角度稍微修改开始时间。 没有看到完整的演示,我不确定原因。
这行代码的目的是计算当前路径动画的距离。 结果的形式为从0到100的坐标值。
只需一行代码即可完成很多工作。 因此,让我们分解各个步骤。
首先,我们将经过time
为最小0。
Math.max(time, 0)
换句话说,动画开始时间之前的所有内容都为零。
然后我们除以动画的持续时间。
Math.max(time, 0) / duration
这将导致从0(代表动画的开始)到1(代表动画的结束)的值。 但是,如果经过的时间是在动画结束之后,则该值也可能大于1。 因此,下一步。
现在将此值限制为最大值1。
Math.min( Math.max(time, 0) / duration, 1)
现在,我们有一个值> = 0和<= 1,该值描述了在动画过程中路径应位于的位置。 如果我们应该在动画开始位置,则为0。 1,如果我们应该处于动画结束位置。 如果动画正在进行,则介于两者之间。
但是,该值严格线性,与时间的推移相对应。 通常,直线运动不是您想要的。 这是不自然的。 物体在开始移动时会加速,在停止时会减速。 这将是easeInOut()
函数将要执行的操作。 如果您不熟悉缓和曲线,请查看下图。
资料来源: Google:轻松的基础
因此,我们传入一个从0..1(水平轴)开始的线性时间值。 它将返回修改后的值,其中考虑了加速和减速。
最后一步是乘以100,以转换为最终坐标值(0..100)。
希望这可以帮助。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.