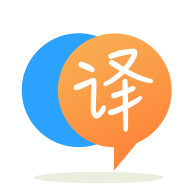
[英]How can I call a JavaScript function in a callback of another function?
[英]In .filter() how do I make a callback function call another function?
我试图摆脱callback functions
但我遇到了一些我不完全理解的东西。
在下面的示例中使用时,此代码块非常有用。
zombieCreatures = creatures.filter(filterCreatures);
不幸的是,当在同一个例子中使用时,这段代码不起作用。
zombieCreatures = creatures.filter(function(v) {
filterCreatures(v);
});
对我来说,他们看起来像是相同的指示,但显然我不对。 有什么不同? 是否有可能使回调函数调用.filter()
内的另一个函数?
'use strict'; var creatures = [], zombieCreatures = []; var filterCreatures; creatures = [ {species: 'Zombie', hitPoints: 90}, {species: 'Orc', hitPoints: 40}, {species: 'Skeleton', hitPoints: 15}, {species: 'Zombie', hitPoints: 85} ]; filterCreatures = function(v) { return v.species === 'Zombie'; } zombieCreatures = creatures.filter(function(v) { filterCreatures(v); }); console.log(zombieCreatures);
是的,您当然可以调用filter
回调中的任何其他函数。
你唯一缺少的是回调需要返回一个值。 你的回调没有return
语句。 它调用filterCreatures()
函数,但随后它会丢弃该函数返回的值,默认情况下返回undefined
。
与原始版本的代码比较:
creatures.filter(filterCreatures);
在此代码中, filter()
直接调用filterCreatures
函数,然后使用其返回值来决定是否包含该元素。 您没有在代码中明确地看到这一点,但filter()
正在寻找该返回值。
这是一个练习,以帮助澄清这一点。 获取损坏的代码并将内联过滤器回调移动到命名函数。 我们称之为filterCreaturesWrapper
。 所以不是这样的:
filterCreatures = function(v) {
return v.species === 'Zombie';
}
zombieCreatures = creatures.filter(function(v) {
filterCreatures(v);
});
我们有这个:
filterCreatures = function(v) {
return v.species === 'Zombie';
}
filterCreaturesWrapper = function(v) {
filterCreatures(v);
}
zombieCreatures = creatures.filter(filterCreaturesWrapper);
如果你研究它,你会发现它与以前的代码完全相同,我们只是稍微移动了一下。 filterCreaturesWrapper
与.filter()
调用内联函数相同。 现在问题应该跳出来了: filterCreatures
有一个return
语句而filterCreaturesWrapper
没有。
回到原来破碎的代码,就好像我们写了:
zombieCreatures = creatures.filter(function(v) {
filterCreatures(v);
return undefined; // .filter treats this as 'false'
});
所以只需在回调中添加一个return
就可以了:
'use strict'; var creatures = [], zombieCreatures = []; var filterCreatures; creatures = [ {species: 'Zombie', hitPoints: 90}, {species: 'Orc', hitPoints: 40}, {species: 'Skeleton', hitPoints: 15}, {species: 'Zombie', hitPoints: 85} ]; filterCreatures = function(v) { return v.species === 'Zombie'; } zombieCreatures = creatures.filter(function(v) { return filterCreatures(v); // <-- added "return" }); console.log(zombieCreatures);
并非每种语言都是这样的。 例如,Ruby会返回方法(函数)中的最后一个表达式,无论您是否明确表示return
。 因此,如果您正在编写Ruby代码(假设所有其他位也转换为Ruby),您的代码就可以了!
filter
将调用为数组中的每个项目指定的函数。 它将检查每个项目的该函数的返回值,如果返回值是真实的,它将在新数组中添加该项目。
换句话说,它返回一个数组,其中所有项目的回调都返回一个truthy值。
在第一个示例中,您直接传递filterCreatures
函数,当该生物是僵尸时返回true
。
在第二个示例中,您正在传递另一个函数并filterCreatures
内部调用filterCreatures
,但是没有返回任何意味着传递给过滤器的回调返回每个项目的undefined
,这是假的并且没有任何过滤。
要使其工作,您必须返回调用filterCreatures
的结果:
zombieCreatures = creatures.filter(function(v) {
return filterCreatures(v);
});
话虽这么说,周围的附加功能filterCreatures
没有多大用处时filterCreatures
本身并没有你所需要的一切。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.